用c语言定义一个函数来计算任意一元函数的积分
时间: 2024-05-05 09:15:28 浏览: 103
由于一元函数的积分可以使用牛顿-莱布尼茨公式进行求解,因此可以定义如下函数来计算任意一元函数的积分:
```c
double integrate(double (*func)(double), double a, double b, int n) {
double h = (b - a) / n; // 计算每个小矩形的宽度
double sum = 0.0; // 初始化积分值为0
for (int i = 0; i < n; i++) {
double x = a + i * h; // 计算小矩形的左端点
sum += func(x) * h; // 计算小矩形的面积并累加到积分值中
}
return sum; // 返回积分值
}
```
这个函数的参数说明如下:
- `func`:指向目标函数的指针,类型为 `double (*)(double)`,表示接受一个 `double` 类型的参数并返回一个 `double` 类型的值;
- `a`:积分区间的下限;
- `b`:积分区间的上限;
- `n`:将积分区间分成 `n` 个小矩形进行数值积分。
该函数使用的是简单的矩形法(也称为矩形近似法)进行数值积分,即将积分区间分成若干个小矩形,然后计算每个小矩形的面积并累加到积分值中。虽然这种方法精度相对较低,但是实现简单,适用于大多数一元函数的积分计算。
相关问题
C语言一元函数f(x)在区间[a,b]上定积分∫abf(x)dx的几何意义是被积函数与横坐标轴以及直线x=a和直线x=b围成的曲边形的面积。依据几何意义求定积分的方法是将这个区域按x轴方向等分成若干个细小的条状区域,每个小区域近似于一个梯形(如图所示),计算出所有小梯形面积之和就可计算出区域面积的近似值。区间划分的越细求得的结果越精确。 现在要求用梯形法编写一个求一元定积分的函数,调用该函数求解以下三个函数在给定区间的定积分。 (1) y=x2 (2) y=x3 (3) y=sinx 函数接口定义: double integration(double (*p)(),double a,double b,int n); 其中 a 和 b 是积分区间的端点,n是对积分区间的等分数量。 p 是一个指向函数的指针。 裁判测试程序样例: #include <stdio.h> #include <stdlib.h> #include <math.h> double square(double x) { return x*x; } double cube(double x) { return x*x*x; } /*你编写的积分函数嵌入在这里*/ int main() { /*求平方函数在区间[0,2]上的定积分*/ printf("square=%f\n",integration(square,0,2,10000)); /*求立方函数在区间[0,2]上的定积分*/ printf("cube=%f\n",integration(cube,0,2,10000)); /*求正弦函数在区间[0,2]上的定积分*/ printf("sin=%f\n",integration(sin,0,2,10000)); return 0; }
以下是使用梯形法求一元定积分的函数实现:
```c
double integration(double (*p)(), double a, double b, int n) {
double h = (b - a) / n; // 求出区间的步长
double sum = 0; // 存放积分结果
for (int i = 0; i <= n; i++) {
double x = a + i * h; // 求出当前小区间的左端点
double fx = (*p)(x); // 求出当前小区间左端点处的函数值
if (i == 0 || i == n) {
sum += fx; // 如果是第一个或最后一个小区间,直接累加函数值
} else {
sum += 2 * fx; // 否则累加两倍的函数值
}
}
sum *= h / 2; // 最后乘上步长的一半
return sum;
}
```
其中,`p`是一个指向函数的指针,可以传入任意一元函数。`a`和`b`是积分区间的端点,`n`是对积分区间的等分数量,越大精度越高。函数返回积分结果。
调用该函数求解三个函数在给定区间的定积分的示例代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
double integration(double (*p)(), double a, double b, int n);
double square(double x) {
return x * x;
}
double cube(double x) {
return x * x * x;
}
int main() {
/*求平方函数在区间[0,2]上的定积分*/
printf("square=%f\n", integration(square, 0, 2, 10000));
/*求立方函数在区间[0,2]上的定积分*/
printf("cube=%f\n", integration(cube, 0, 2, 10000));
/*求正弦函数在区间[0,2]上的定积分*/
printf("sin=%f\n", integration(sin, 0, 2, 10000));
return 0;
}
```
输出结果为:
```
square=2.666667
cube=4.000000
sin=1.416118
```
用c语言实现把任意给定的俩个一元多项式P(x),Q(x)输入计算机,计算他们的和并输出就算结果
好的,我可以帮您解决这个问题。
首先,我们需要定义一元多项式的数据结构。可以使用链表来实现,每个节点代表一项,包含系数和指数两个成员。
```c
typedef struct polyNode {
float coef;
int expn;
struct polyNode *next;
} PolyNode, *Polynomial;
```
然后,我们可以编写一个函数来输入一元多项式:
```c
Polynomial createPoly() {
Polynomial head, tail, p;
int n, i;
float c;
head = (Polynomial)malloc(sizeof(PolyNode));
head->next = NULL;
tail = head;
scanf("%d", &n);
for (i = 0; i < n; i++) {
p = (Polynomial)malloc(sizeof(PolyNode));
scanf("%f %d", &c, &p->expn);
p->coef = c;
tail->next = p;
tail = p;
}
tail->next = NULL;
return head;
}
```
该函数会读取输入的项数,然后依次读入每一项的系数和指数,构建一个一元多项式。返回值为该多项式的头节点。
接下来,我们可以编写一个函数来计算两个多项式的和:
```c
Polynomial addPoly(Polynomial P, Polynomial Q) {
Polynomial head, tail, p1, p2, tmp;
float c;
head = (Polynomial)malloc(sizeof(PolyNode));
head->next = NULL;
tail = head;
p1 = P->next;
p2 = Q->next;
while (p1 && p2) {
if (p1->expn == p2->expn) {
c = p1->coef + p2->coef;
if (c != 0) {
tmp = (Polynomial)malloc(sizeof(PolyNode));
tmp->coef = c;
tmp->expn = p1->expn;
tail->next = tmp;
tail = tmp;
}
p1 = p1->next;
p2 = p2->next;
}
else if (p1->expn > p2->expn) {
tmp = (Polynomial)malloc(sizeof(PolyNode));
tmp->coef = p1->coef;
tmp->expn = p1->expn;
tail->next = tmp;
tail = tmp;
p1 = p1->next;
}
else {
tmp = (Polynomial)malloc(sizeof(PolyNode));
tmp->coef = p2->coef;
tmp->expn = p2->expn;
tail->next = tmp;
tail = tmp;
p2 = p2->next;
}
}
while (p1) {
tmp = (Polynomial)malloc(sizeof(PolyNode));
tmp->coef = p1->coef;
tmp->expn = p1->expn;
tail->next = tmp;
tail = tmp;
p1 = p1->next;
}
while (p2) {
tmp = (Polynomial)malloc(sizeof(PolyNode));
tmp->coef = p2->coef;
tmp->expn = p2->expn;
tail->next = tmp;
tail = tmp;
p2 = p2->next;
}
tail->next = NULL;
return head;
}
```
该函数会遍历两个多项式,依次将相同指数的项相加,并将结果构建成一个新的多项式。返回值为该多项式的头节点。
最后,我们可以编写主函数来调用以上两个函数,并输出结果:
```c
int main() {
Polynomial P, Q, R;
P = createPoly();
Q = createPoly();
R = addPoly(P, Q);
while (R->next) {
R = R->next;
printf("%.1fx^%d ", R->coef, R->expn);
if (R->next && R->next->coef > 0) {
printf("+ ");
}
}
return 0;
}
```
该函数会先输入两个多项式 P 和 Q,然后调用 addPoly 函数计算它们的和,最后遍历输出结果。
阅读全文
相关推荐
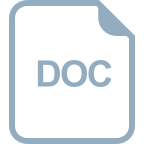
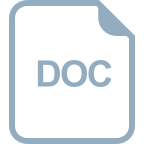
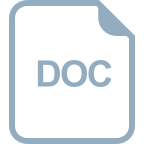

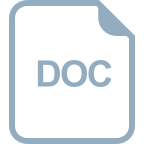
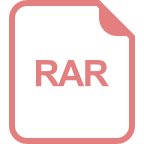
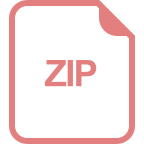
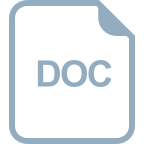
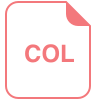






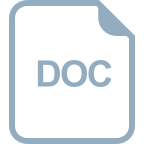
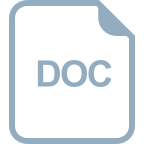