qt编写一段程序,实现导入图片,并实现用按钮对图片亮度增大和减小
时间: 2023-11-27 12:49:57 浏览: 35
以下是使用Qt编写的程序,实现导入图片并实现用按钮对图片亮度增大和减小的功能。在实现过程中,我们使用了Qt的GUI组件QPixmap、QGraphicsScene、QGraphicsView和QPushButton。
```cpp
#include <QtWidgets/QApplication>
#include <QtWidgets/QFileDialog>
#include <QtWidgets/QGraphicsScene>
#include <QtWidgets/QGraphicsView>
#include <QtWidgets/QHBoxLayout>
#include <QtWidgets/QPushButton>
class MainWindow : public QWidget {
public:
MainWindow(QWidget* parent = nullptr) : QWidget(parent) {
// 创建布局
QHBoxLayout* layout = new QHBoxLayout(this);
// 创建按钮
QPushButton* increaseButton = new QPushButton("Increase Brightness", this);
QPushButton* decreaseButton = new QPushButton("Decrease Brightness", this);
// 添加按钮到布局中
layout->addWidget(increaseButton);
layout->addWidget(decreaseButton);
// 创建场景
scene = new QGraphicsScene(this);
// 创建视图
view = new QGraphicsView(this);
view->setScene(scene);
// 添加视图到布局中
layout->addWidget(view);
// 连接按钮的信号和槽函数
connect(increaseButton, &QPushButton::clicked, this, &MainWindow::increaseBrightness);
connect(decreaseButton, &QPushButton::clicked, this, &MainWindow::decreaseBrightness);
}
private:
// 增加亮度
void increaseBrightness() {
if (pixmap.isNull()) return;
QPixmap newPixmap = pixmap;
newPixmap.setAlphaChannel(newPixmap.alphaChannel().adjusted(0, 0, 0, brightnessStep));
scene->clear();
scene->addPixmap(newPixmap);
view->fitInView(scene->itemsBoundingRect(), Qt::KeepAspectRatio);
pixmap = newPixmap;
}
// 减少亮度
void decreaseBrightness() {
if (pixmap.isNull()) return;
QPixmap newPixmap = pixmap;
newPixmap.setAlphaChannel(newPixmap.alphaChannel().adjusted(0, 0, 0, -brightnessStep));
scene->clear();
scene->addPixmap(newPixmap);
view->fitInView(scene->itemsBoundingRect(), Qt::KeepAspectRatio);
pixmap = newPixmap;
}
// 加载图片
void openImage() {
QString fileName = QFileDialog::getOpenFileName(this, "Open Image", "", "Images (*.png *.xpm *.jpg)");
if (!fileName.isEmpty()) {
QPixmap newPixmap(fileName);
scene->clear();
scene->addPixmap(newPixmap);
view->fitInView(scene->itemsBoundingRect(), Qt::KeepAspectRatio);
pixmap = newPixmap;
}
}
QPixmap pixmap;
QGraphicsScene* scene;
QGraphicsView* view;
int brightnessStep = 10;
};
int main(int argc, char* argv[]) {
QApplication app(argc, argv);
MainWindow mainWindow;
mainWindow.show();
return app.exec();
}
```
在程序中,我们使用QHBoxLayout创建一个水平布局,然后创建两个QPushButton作为按钮,分别处理增加亮度和减少亮度的操作。接着,我们创建一个QGraphicsScene作为场景,将其添加到QGraphicsView中,最后将QGraphicsView添加到布局中。
在增加亮度和减少亮度的槽函数中,我们通过调整QPixmap的alpha通道来改变图片的亮度。在每次操作后,我们需要清除场景并重新添加修改后的QPixmap,然后调用QGraphicsView的fitInView方法使其适应场景的大小。
在打开图片的槽函数中,我们使用QFileDialog让用户选择要导入的图片,并将其加载到QPixmap中。如果用户没有选择图片,则不做任何操作。
最后,在main函数中,我们创建一个QApplication并将MainWindow显示出来。
相关推荐
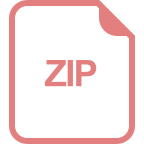
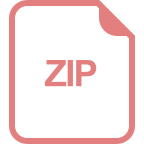














