设计一个 Point 类表示平面坐标系中的点。该类包括私有的double型的x 和y属性。两个构造方法,一个是无参的构造方法,该方法中×和y的值为0;另外一个构造方法包含两个参数,分别为x和y赋值。为×和y属性提供 setter 和getter 方法,重写object 类的hashcode 和equals 方法。最后重写 object 类的tostring ( 方法。 编写一个类,包含如下功能:(1)定义一个数组,数组的长度是50,数组元素是Point 的对象。Point对象的×和y值随机赋值。 (2)判断数组中是否有两个相等的点(点的×和y的值相等) (3)指定一个点,求该点到其他点的距离(两种理解) (4)求距离最近的两个点。
时间: 2023-05-25 14:02:15 浏览: 100
Java代码如下:
Point类的实现:
public class Point {
private double x;
private double y;
// 无参构造方法
public Point() {
this.x = 0;
this.y = 0;
}
// 带参构造方法
public Point(double x, double y) {
this.x = x;
this.y = y;
}
// x属性的setter和getter方法
public void setX(double x) {
this.x = x;
}
public double getX() {
return this.x;
}
// y属性的setter和getter方法
public void setY(double y) {
this.y = y;
}
public double getY() {
return this.y;
}
// 重写Object类的hashCode方法
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
long temp;
temp = Double.doubleToLongBits(x);
result = prime * result + (int) (temp ^ (temp >>> 32));
temp = Double.doubleToLongBits(y);
result = prime * result + (int) (temp ^ (temp >>> 32));
return result;
}
// 重写Object类的equals方法
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
Point other = (Point) obj;
if (Double.doubleToLongBits(x) != Double.doubleToLongBits(other.x))
return false;
if (Double.doubleToLongBits(y) != Double.doubleToLongBits(other.y))
return false;
return true;
}
// 重写Object类的toString方法
@Override
public String toString() {
return "Point [x=" + x + ", y=" + y + "]";
}
// 计算该点到另一个点的距离
public double distance(Point point) {
double dx = this.x - point.x;
double dy = this.y - point.y;
return Math.sqrt(dx * dx + dy * dy);
}
}
主程序的实现:
import java.util.Random;
public class Main {
public static void main(String[] args) {
Point[] points = new Point[50];
Random random = new Random();
// 初始化数组,随机赋值x和y
for (int i = 0; i < points.length; i++) {
double x = random.nextInt(101);
double y = random.nextInt(101);
points[i] = new Point(x, y);
}
// 判断数组中是否有两个相等的点
boolean hasDuplicate = false;
for (int i = 0; i < points.length; i++) {
for (int j = i + 1; j < points.length; j++) {
if (points[i].equals(points[j])) {
hasDuplicate = true;
System.out.println("数组中存在相等的点:" + points[i].toString() + "和" + points[j].toString());
}
}
}
if (!hasDuplicate) {
System.out.println("数组中不存在相等的点。");
}
// 指定一个点,求该点到其他点的距离
Point referencePoint = new Point(50, 50);
System.out.println("与参照点" + referencePoint.toString() + "的距离:");
for (int i = 0; i < points.length; i++) {
System.out.println(points[i].toString() + ": " + points[i].distance(referencePoint));
}
// 求距离最近的两个点
int index1 = 0;
int index2 = 1;
double minDistance = points[0].distance(points[1]);
for (int i = 0; i < points.length; i++) {
for (int j = i + 1; j < points.length; j++) {
double distance = points[i].distance(points[j]);
if (distance < minDistance) {
index1 = i;
index2 = j;
minDistance = distance;
}
}
}
System.out.println("距离最近的两个点:" + points[index1].toString() + "和" + points[index2].toString() + ",距离为" + minDistance);
}
}
阅读全文
相关推荐
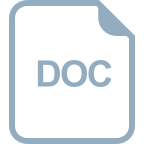
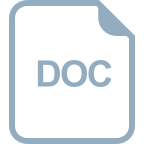
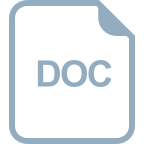










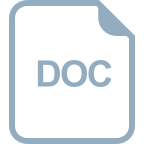




