输入格式: 在一行中输入若干个数,以空格分隔。 输出格式: 输出升序排序后的数,以空格分隔,最后一个数后也有空格 输入样例: 在这里给出一组输入。例如: 4 5 67 3 99 2 7 输出样例: 在这里给出相应的输出。例如: 2 3 4 5 7 67 99
时间: 2023-08-31 18:43:38 浏览: 70
### 回答1:
您好!这是一个简单的排序问题,可以使用快速排序或归并排序等算法进行排序。以下是使用快速排序的示例代码:
```
#include <stdio.h>
#include <stdlib.h>
void swap(int *a, int *b) {
int tmp = *a;
*a = *b;
*b = tmp;
}
int partition(int arr[], int low, int high) {
int pivot = arr[high];
int i = low - 1;
for (int j = low; j <= high - 1; j++) {
if (arr[j] < pivot) {
i++;
swap(&arr[i], &arr[j]);
}
}
swap(&arr[i + 1], &arr[high]);
return i + 1;
}
void quick_sort(int arr[], int low, int high) {
if (low < high) {
int pivot_index = partition(arr, low, high);
quick_sort(arr, low, pivot_index - 1);
quick_sort(arr, pivot_index + 1, high);
}
}
int main() {
int arr[1000];
int n = 0;
// 读入数据
while (scanf("%d", &arr[n]) != EOF) {
n++;
}
// 使用快速排序对数据进行升序排序
quick_sort(arr, 0, n - 1);
// 输出升序排序后的数据
for (int i = 0; i < n; i++) {
printf("%d ", arr[i]);
}
printf("\n");
return 0;
}
```
示例代码中使用了快速排序算法,可以对输入的数据进行升序排序。输入的数据可以通过循环读入,直到读入结束符(EOF)为止。最后将排序后的数据输出即可。
希望这个回答能够解决您的问题,如果还有任何疑问,请随时问我。
### 回答2:
题目要求对一行输入的若干个数进行升序排序并输出。
可以使用以下步骤解决该问题:
1. 读取一行输入,并使用空格分隔成若干个字符串。
2. 将这些字符串转换为整数,并存入一个列表中。
3. 使用Python内置的sorted函数对列表进行升序排序。
4. 使用空格将排序后的列表转换为字符串。
5. 输出排序后的字符串。
以下是使用Python代码实现上述步骤的示例:
```python
# 读取输入并分隔为字符串列表
input_str = input()
nums_str = input_str.split()
# 将字符串列表转换为整数列表
nums = [int(num_str) for num_str in nums_str]
# 对整数列表进行排序
sorted_nums = sorted(nums)
# 将排序后的列表转换为字符串,并使用空格连接
output_str = ' '.join(str(num) for num in sorted_nums)
# 输出排序后的字符串
print(output_str)
```
运行示例:
```
输入:
4 5 67 3 99 2 7
输出:
2 3 4 5 7 67 99
```
### 回答3:
可以使用 Python 的内置函数`sorted()`对输入的数进行升序排序,并使用`join()`方法将排序后的数以空格分隔输出。
代码如下:
```python
# 获取输入
nums = input().split()
# 将输入的数转换为整型
nums = [int(num) for num in nums]
# 升序排序
sorted_nums = sorted(nums)
# 将排序后的数以空格分隔输出
output = ' '.join(map(str, sorted_nums))
# 打印输出结果
print(output)
```
对于输入样例`4 5 67 3 99 2 7`,代码的输出结果为`2 3 4 5 7 67 99`。
相关推荐
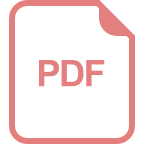
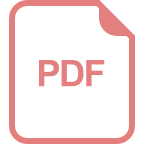
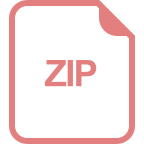









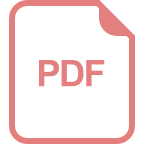
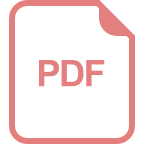
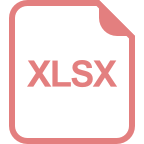