fpga设计简易数字时钟
时间: 2023-08-31 14:12:34 浏览: 101
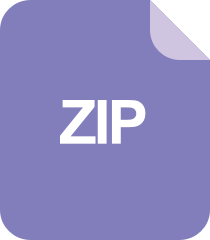
基于FPGA的简易数字时钟设计

FPGA设计数字时钟可以分为以下几个步骤:
1. 确定时钟频率:选择适合的时钟频率,例如1MHz或10MHz。
2. 时钟分频器:使用计数器模块将时钟频率分频,以得到所需的时钟周期。例如,如果我们需要1秒的时钟周期,则可以将1MHz的时钟频率分频为1Hz。
3. 显示模块:使用七段数码管显示小时、分钟和秒数。每个数码管由7个LED灯组成,可以通过控制每个LED的亮灭状态来显示数字。
4. 按钮模块:使用按钮模块来设置小时和分钟。可以使用两个按钮来增加或减少小时和分钟,可以使用一个按钮来切换小时和分钟的设置。
5. 时钟模块:将所有模块集成在一起,实现一个完整的数字时钟系统。
下面是一个简单的FPGA数字时钟设计代码示例,以VHDL语言为例:
```
-- 时钟分频器模块
entity clock_divider is
port(
clk_in: in std_logic;
clk_out: out std_logic
);
end entity;
architecture behavioral of clock_divider is
signal counter: integer range 0 to 999999;
begin
process(clk_in)
begin
if rising_edge(clk_in) then
if counter = 999999 then
counter <= 0;
clk_out <= not clk_out; -- 时钟输出反转
else
counter <= counter + 1;
end if;
end if;
end process;
end architecture;
-- 数字显示模块
entity display is
port(
clk: in std_logic;
hour: in integer range 0 to 23;
minute: in integer range 0 to 59;
second: in integer range 0 to 59;
seg: out std_logic_vector(6 downto 0);
an: out std_logic_vector(3 downto 0)
);
end entity;
architecture behavioral of display is
signal count: integer range 0 to 999;
signal an_count: integer range 0 to 3;
signal seg_count: integer range 0 to 6;
signal seg_temp: std_logic_vector(6 downto 0);
begin
process(clk)
begin
if rising_edge(clk) then
if count = 999 then
count <= 0;
seg_count <= seg_count + 1;
if seg_count = 7 then
seg_count <= 0;
an_count <= an_count + 1;
if an_count = 4 then
an_count <= 0;
end if;
end if;
else
count <= count + 1;
end if;
end if;
case an_count is
when 0 =>
case seg_count is
when 0 => seg_temp <= "0000001"; -- 数字0
when 1 => seg_temp <= std_logic_vector(to_unsigned(hour / 10, 4)); -- 十位小时数
when 2 => seg_temp <= std_logic_vector(to_unsigned(hour mod 10, 4)); -- 个位小时数
when 3 => seg_temp <= "1001111"; -- 冒号
when 4 => seg_temp <= std_logic_vector(to_unsigned(minute / 10, 4)); -- 十位分钟数
when 5 => seg_temp <= std_logic_vector(to_unsigned(minute mod 10, 4)); -- 个位分钟数
when 6 => seg_temp <= std_logic_vector(to_unsigned(second / 10, 4)); -- 十位秒数
when others => null;
end case;
when 1 =>
case seg_count is
when 0 => seg_temp <= "0000001"; -- 数字0
when 1 => seg_temp <= "0000001"; -- 数字0
when 2 => seg_temp <= std_logic_vector(to_unsigned(hour mod 10, 4)); -- 个位小时数
when 3 => seg_temp <= "1001111"; -- 冒号
when 4 => seg_temp <= std_logic_vector(to_unsigned(minute / 10, 4)); -- 十位分钟数
when 5 => seg_temp <= std_logic_vector(to_unsigned(minute mod 10, 4)); -- 个位分钟数
when 6 => seg_temp <= std_logic_vector(to_unsigned(second mod 10, 4)); -- 个位秒数
when others => null;
end case;
when 2 =>
case seg_count is
when 0 => seg_temp <= "0000001"; -- 数字0
when 1 => seg_temp <= std_logic_vector(to_unsigned(hour / 10, 4)); -- 十位小时数
when 2 => seg_temp <= std_logic_vector(to_unsigned(hour mod 10, 4)); -- 个位小时数
when 3 => seg_temp <= "1001111"; -- 冒号
when 4 => seg_temp <= std_logic_vector(to_unsigned(minute / 10, 4)); -- 十位分钟数
when 5 => seg_temp <= std_logic_vector(to_unsigned(minute mod 10, 4)); -- 个位分钟数
when 6 => seg_temp <= std_logic_vector(to_unsigned(second / 10, 4)); -- 十位秒数
when others => null;
end case;
when 3 =>
case seg_count is
when 0 => seg_temp <= "0000001"; -- 数字0
when 1 => seg_temp <= "0000001"; -- 数字0
when 2 => seg_temp <= std_logic_vector(to_unsigned(hour mod 10, 4)); -- 个位小时数
when 3 => seg_temp <= "1001111"; -- 冒号
when 4 => seg_temp <= std_logic_vector(to_unsigned(minute mod 10, 4)); -- 个位分钟数
when 5 => seg_temp <= std_logic_vector(to_unsigned(second / 10, 4)); -- 十位秒数
when 6 => seg_temp <= std_logic_vector(to_unsigned(second mod 10, 4)); -- 个位秒数
when others => null;
end case;
when others => null;
end case;
seg <= seg_temp;
case an_count is
when 0 => an <= "1110"; -- 第一个数码管
when 1 => an <= "1101"; -- 第二个数码管
when 2 => an <= "1011"; -- 第三个数码管
when 3 => an <= "0111"; -- 第四个数码管
when others => null;
end case;
end process;
end architecture;
-- 时钟模块
entity clock is
port(
clk_in: in std_logic;
reset: in std_logic;
hour_set: in std_logic;
minute_set: in std_logic;
hour_inc: in std_logic;
hour_dec: in std_logic;
minute_inc: in std_logic;
minute_dec: in std_logic;
seg: out std_logic_vector(6 downto 0);
an: out std_logic_vector(3 downto 0)
);
end entity;
architecture behavioral of clock is
signal clk_out: std_logic;
signal hour: integer range 0 to 23;
signal minute: integer range 0 to 59;
signal second: integer range 0 to 59;
begin
clock_div: entity work.clock_divider port map(clk_in => clk_in, clk_out => clk_out);
display: entity work.display port map(clk => clk_out, hour => hour, minute => minute, second => second, seg => seg, an => an);
process(clk_out, reset, hour_set, minute_set, hour_inc, hour_dec, minute_inc, minute_dec)
begin
if reset = '1' then -- 复位
hour <= 0;
minute <= 0;
second <= 0;
elsif rising_edge(clk_out) then
if hour_set = '1' then -- 设置小时
if hour_inc = '1' then
hour <= hour + 1;
elsif hour_dec = '1' then
hour <= hour - 1;
end if;
end if;
if minute_set = '1' then -- 设置分钟
if minute_inc = '1' then
minute <= minute + 1;
elsif minute_dec = '1' then
minute <= minute - 1;
end if;
end if;
second <= second + 1;
if second = 60 then -- 每60秒增加一分钟
second <= 0;
minute <= minute + 1;
if minute = 60 then -- 每60分钟增加一小时
minute <= 0;
hour <= hour + 1;
if hour = 24 then -- 每24小时归零
hour <= 0;
end if;
end if;
end if;
end if;
end process;
end architecture;
```
以上代码示例仅供参考,实际设计中还需要根据具体的需求进行调整和优化。
阅读全文
相关推荐


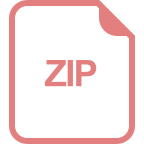
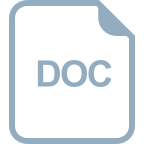

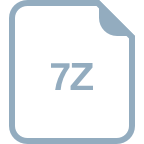
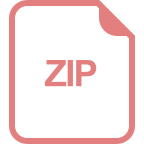
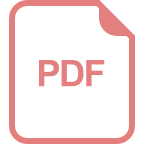
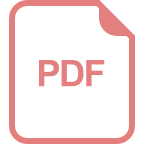
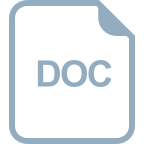
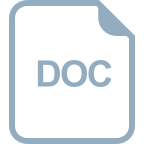
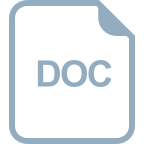
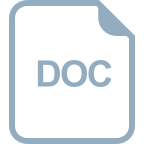

