col_sum = np.sum(img, axis=0)
时间: 2023-07-24 19:17:15 浏览: 82
这行代码使用了 numpy 库中的 `np.sum()` 函数,对二维数组 `img` 进行了求和操作。其中,`axis=0` 参数表示按列进行求和,即将每一列的元素相加,得到一个一维数组 `col_sum`。
在图像处理中,可以使用 `np.sum()` 函数对图像的像素值进行统计,例如计算每一列或每一行的像素值总和,得到一个表示图像亮度分布的曲线。通过观察这个曲线,可以了解到图像中哪些区域的亮度较高或较低,从而进行后续的图像处理。
需要注意的是,`np.sum()` 函数对于像素值为负数的图像,会将负数像素值转换为 0,因此在进行像素值统计时需要注意数据类型和像素值范围。
相关问题
def find_center(img): h, w = img.shape roi_h = int(h * 2 / 3) roi_img = img[roi_h:, :] img_blur = cv2.GaussianBlur(roi_img, (15, 15), 0) # 高斯模糊 ret, th2 = cv2.threshold(img_blur, 0, 255, cv2.THRESH_BINARY + cv2.THRESH_OTSU) g2 = cv2.getStructuringElement(cv2.MORPH_RECT, (3, 3)) open_img = cv2.morphologyEx(th2, cv2.MORPH_OPEN, g2, iterations=3) x_sum = np.sum(open_img, axis=0) x_point = np.where(x_sum > 0) point_x = int((x_point[0][0] + x_point[0][-1]) / 2) # print(roi_h, w) # np.savetxt('reshape_data.txt', x_point, delimiter=' ', fmt='%i') return point_x c++ Eigen opencv
这段代码是一个Python函数,用于从一张图像中找到中心点的x坐标。其中使用了OpenCV库中的一些函数进行图像处理。
如果需要将这段代码转化为C++代码,可以使用Eigen和OpenCV库来实现:
```c++
#include <opencv2/opencv.hpp>
#include <Eigen/Core>
int find_center(cv::Mat img) {
int h = img.rows;
int w = img.cols;
int roi_h = h * 2 / 3;
cv::Mat roi_img = img(cv::Rect(0, roi_h, w, h - roi_h));
cv::Mat img_blur;
cv::GaussianBlur(roi_img, img_blur, cv::Size(15, 15), 0);
cv::Mat th2;
cv::threshold(img_blur, th2, 0, 255, cv::THRESH_BINARY + cv::THRESH_OTSU);
cv::Mat g2 = cv::getStructuringElement(cv::MORPH_RECT, cv::Size(3, 3));
cv::Mat open_img;
cv::morphologyEx(th2, open_img, cv::MORPH_OPEN, g2, cv::Point(-1, -1), 3);
Eigen::MatrixXi open_mat(h - roi_h, w);
for (int i = 0; i < h - roi_h; i++) {
for (int j = 0; j < w; j++) {
open_mat(i, j) = open_img.at<uchar>(i, j);
}
}
Eigen::VectorXi x_sum(w);
for (int i = 0; i < w; i++) {
x_sum(i) = open_mat.col(i).sum();
}
Eigen::ArrayXi x_point = (x_sum.array() > 0).select(Eigen::ArrayXi::LinSpaced(w, 0, w - 1), -1);
int point_x = (x_point(0) + x_point(w - 1)) / 2;
return point_x;
}
```
注意,此处使用了Eigen库来进行矩阵计算,需要在代码中引入相应的头文件和命名空间。同时,将OpenCV中的Mat转换为Eigen库中的Matrix类型,需要使用循环将每个像素的值拷贝到矩阵中。
import torch, os, cv2 from model.model import parsingNet from utils.common import merge_config from utils.dist_utils import dist_print import torch import scipy.special, tqdm import numpy as np import torchvision.transforms as transforms from data.dataset import LaneTestDataset from data.constant import culane_row_anchor, tusimple_row_anchor if __name__ == "__main__": torch.backends.cudnn.benchmark = True args, cfg = merge_config() dist_print('start testing...') assert cfg.backbone in ['18','34','50','101','152','50next','101next','50wide','101wide'] if cfg.dataset == 'CULane': cls_num_per_lane = 18 elif cfg.dataset == 'Tusimple': cls_num_per_lane = 56 else: raise NotImplementedError net = parsingNet(pretrained = False, backbone=cfg.backbone,cls_dim = (cfg.griding_num+1,cls_num_per_lane,4), use_aux=False).cuda() # we dont need auxiliary segmentation in testing state_dict = torch.load(cfg.test_model, map_location='cpu')['model'] compatible_state_dict = {} for k, v in state_dict.items(): if 'module.' in k: compatible_state_dict[k[7:]] = v else: compatible_state_dict[k] = v net.load_state_dict(compatible_state_dict, strict=False) net.eval() img_transforms = transforms.Compose([ transforms.Resize((288, 800)), transforms.ToTensor(), transforms.Normalize((0.485, 0.456, 0.406), (0.229, 0.224, 0.225)), ]) if cfg.dataset == 'CULane': splits = ['test0_normal.txt', 'test1_crowd.txt', 'test2_hlight.txt', 'test3_shadow.txt', 'test4_noline.txt', 'test5_arrow.txt', 'test6_curve.txt', 'test7_cross.txt', 'test8_night.txt'] datasets = [LaneTestDataset(cfg.data_root,os.path.join(cfg.data_root, 'list/test_split/'+split),img_transform = img_transforms) for split in splits] img_w, img_h = 1640, 590 row_anchor = culane_row_anchor elif cfg.dataset == 'Tusimple': splits = ['test.txt'] datasets = [LaneTestDataset(cfg.data_root,os.path.join(cfg.data_root, split),img_transform = img_transforms) for split in splits] img_w, img_h = 1280, 720 row_anchor = tusimple_row_anchor else: raise NotImplementedError for split, dataset in zip(splits, datasets): loader = torch.utils.data.DataLoader(dataset, batch_size=1, shuffle = False, num_workers=1) fourcc = cv2.VideoWriter_fourcc(*'MJPG') print(split[:-3]+'avi') vout = cv2.VideoWriter(split[:-3]+'avi', fourcc , 30.0, (img_w, img_h)) for i, data in enumerate(tqdm.tqdm(loader)): imgs, names = data imgs = imgs.cuda() with torch.no_grad(): out = net(imgs) col_sample = np.linspace(0, 800 - 1, cfg.griding_num) col_sample_w = col_sample[1] - col_sample[0] out_j = out[0].data.cpu().numpy() out_j = out_j[:, ::-1, :] prob = scipy.special.softmax(out_j[:-1, :, :], axis=0) idx = np.arange(cfg.griding_num) + 1 idx = idx.reshape(-1, 1, 1) loc = np.sum(prob * idx, axis=0) out_j = np.argmax(out_j, axis=0) loc[out_j == cfg.griding_num] = 0 out_j = loc # import pdb; pdb.set_trace() vis = cv2.imread(os.path.join(cfg.data_root,names[0])) for i in range(out_j.shape[1]): if np.sum(out_j[:, i] != 0) > 2: for k in range(out_j.shape[0]): if out_j[k, i] > 0: ppp = (int(out_j[k, i] * col_sample_w * img_w / 800) - 1, int(img_h * (row_anchor[cls_num_per_lane-1-k]/288)) - 1 ) cv2.circle(vis,ppp,5,(0,255,0),-1) vout.write(vis) vout.release()
这是一个使用PyTorch框架测试模型的代码,代码中导入了许多必要的库以及自定义的模型和数据集等。通过调用PyTorch的后端,启用一些加速技术,然后解析配置参数,并根据数据集类型设置类别数。创建一个模型实例,传入必要的参数并将其转移到GPU上以加速处理。最后进行模型测试。
阅读全文
相关推荐
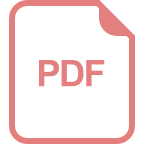
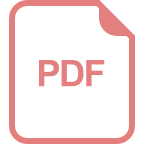
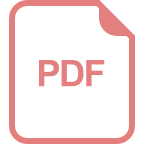
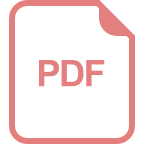
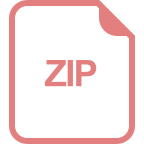
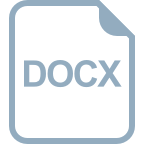
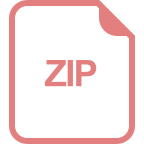
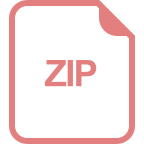
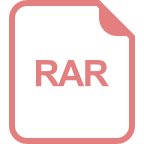
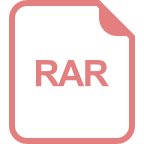