# assume we have Xtr_rows, Ytr, Xte_rows, Yte as before # recall Xtr_rows is 50,000 x 3072 matrix Xval_rows = Xtr_rows[:1000, :] # take first 1000 for validation Yval = Ytr[:1000] Xtr_rows = Xtr_rows[1000:, :] # keep last 49,000 for train Ytr = Ytr[1000:] # find hyperparameters that work best on the validation set validation_accuracies = [] for k in [1, 3, 5, 10, 20, 50, 100]: # use a particular value of k and evaluation on validation data nn = NearestNeighbor() nn.train(Xtr_rows, Ytr) # here we assume a modified NearestNeighbor class that can take a k as input Yval_predict = nn.predict(Xval_rows, k = k) acc = np.mean(Yval_predict == Yval) print 'accuracy: %f' % (acc,) # keep track of what works on the validation set validation_accuracies.append((k, acc))
时间: 2024-04-06 19:30:10 浏览: 91
这段代码是使用 k-最近邻算法对 CIFAR-10 数据集进行分类,使用前 1000 个数据作为验证集,剩下的 49000 个数据作为训练集。代码中的循环用于尝试不同的 k 值,并在验证集上测试它们的准确率,最终选择最优的 k 值作为算法的超参数。
具体地,循环中每次选择一个 k 值,用训练集训练一个 k-最近邻分类器,并使用验证集评估其准确率。这里假设 NearestNeighbor 类已经被修改,可以接受 k 作为输入,用于指定 k-最近邻算法中的 k 值。
每次循环中,用一个元组保存 k 值和在验证集上的准确率,最后将这些元组保存在 validation_accuracies 列表中。代码中的 print 语句用于输出每个 k 值在验证集上的准确率。
相关问题
脚本中 OPT="/opt" # Ardupilot Tools ARDUPILOT_TOOLS="Tools/autotest" ASSUME_YES=false QUIET=false sep="##############################################"
这段脚本的作用是什么?
这段脚本定义了几个变量,分别是:
- OPT:表示一个目录的路径,这个目录路径可以被脚本中的其他命令使用。
- ARDUPILOT_TOOLS:表示 Ardupilot 工具的路径,这个路径也可以被脚本中的其他命令使用。
- ASSUME_YES:表示是否自动确认执行命令,默认值为 false,如果设置为 true,则执行命令时不需要手动确认。
- QUIET:表示是否关闭脚本的输出信息,默认值为 false,如果设置为 true,则脚本将不会输出任何信息。
- sep:表示一个分隔符,用于在脚本中插入分隔线,方便输出信息的阅读。
这些变量的定义可以使脚本的执行更加灵活,并且可以方便地修改变量的值来适应不同的执行环境。
import numpy as np from numpy import int64 def bilinear_interp_baseline(a: np.ndarray, b: np.ndarray) -> np.ndarray: """ This is the baseline implementation of bilinear interpolation without vectorization. - a is a ND array with shape [N, H1, W1, C], dtype = int64 - b is a ND array with shape [N, H2, W2, 2], dtype = float64 - return a ND array with shape [N, H2, W2, C], dtype = int64 """ # Get axis size from ndarray shape N, H1, W1, C = a.shape N1, H2, W2, _ = b.shape assert N == N1 # Do iteration res = np.empty((N, H2, W2, C), dtype=int64) for n in range(N): for i in range(H2): for j in range(W2): x, y = b[n, i, j] x_idx, y_idx = int(np.floor(x)), int(np.floor(y)) _x, _y = x - x_idx, y - y_idx # For simplicity, we assume all x are in [0, H1 - 1), all y are in [0, W1 - 1) res[n, i, j] = a[n, x_idx, y_idx] * (1 - _x) * (1 - _y) + a[n, x_idx + 1, y_idx] * _x * (1 - _y) + \ a[n, x_idx, y_idx + 1] * (1 - _x) * _y + a[n, x_idx + 1, y_idx + 1] * _x * _y return res 将这段代码改成向量化的方式
下面是将代码改写为向量化方式的版本:
```python
import numpy as np
from numpy import int64
def bilinear_interp_vectorized(a: np.ndarray, b: np.ndarray) -> np.ndarray:
"""
This is the vectorized implementation of bilinear interpolation.
- a is a ND array with shape [N, H1, W1, C], dtype = int64
- b is a ND array with shape [N, H2, W2, 2], dtype = float64
- return a ND array with shape [N, H2, W2, C], dtype = int64
"""
# Get axis size from ndarray shape
N, H1, W1, C = a.shape
N1, H2, W2, _ = b.shape
assert N == N1
# Calculate the indices for interpolation
x_idx = np.floor(b[:, :, :, 0]).astype(int)
y_idx = np.floor(b[:, :, :, 1]).astype(int)
x = b[:, :, :, 0] - x_idx
y = b[:, :, :, 1] - y_idx
# Calculate the interpolation values
res = a[:, x_idx, y_idx] * (1 - x) * (1 - y) + a[:, x_idx + 1, y_idx] * x * (1 - y) + \
a[:, x_idx, y_idx + 1] * (1 - x) * y + a[:, x_idx + 1, y_idx + 1] * x * y
return res.astype(int64)
```
这个向量化的版本通过使用数组切片和广播操作,避免了显式的循环,从而提高了代码的执行效率。
阅读全文
相关推荐
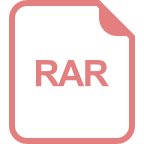
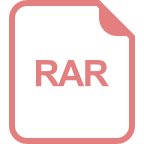
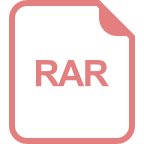













