opencv 读取txt文件内坐标
时间: 2023-08-31 13:43:38 浏览: 121
### 回答1:
如果你的txt文件内的坐标是以一定格式进行存储的,比如每行一个坐标,或者每行有多个坐标,可以使用以下代码读取:
```python
import cv2
# 读取txt文件
with open('coords.txt', 'r') as f:
coords = f.readlines()
# 遍历每个坐标并绘制
img = cv2.imread('image.jpg')
for coord in coords:
x, y = coord.strip().split(',')
x, y = int(x), int(y)
img = cv2.circle(img, (x, y), 5, (0, 255, 0), -1)
cv2.imshow('image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在这个例子中,我们假设txt文件内每行以逗号分隔两个坐标,代码将每个坐标读取并绘制成绿色的圆圈。你需要根据你的实际情况调整代码。
### 回答2:
要使用OpenCV读取txt文件内的坐标,首先要确保txt文件中的坐标数据是以某种格式存储的,比如每行一个坐标,坐标之间用逗号或空格分隔。下面是一个具体的步骤:
1. 打开txt文件并读取内容。
```python
file = open("coordinates.txt", "r")
content = file.read()
file.close()
```
2. 将坐标数据按照分隔符进行切割,并转换为浮点数。
```python
coordinates = content.split(',') # 假设以逗号分隔
coordinates = [float(coord) for coord in coordinates]
```
3. 将坐标数据还原为原始的格式,比如每个点有(x, y)两个坐标值。这里需要注意切割的数量必须是偶数个。
```python
points = [(coordinates[i], coordinates[i+1]) for i in range(0, len(coordinates), 2)]
```
4. 可以根据需要对读取的坐标进行后续处理,比如绘制到图像上。
```python
import cv2
image = cv2.imread("image.jpg")
for point in points:
cv2.circle(image, point, 5, (0, 0, 255), -1)
cv2.imshow("Image", image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这样,就可以使用OpenCV读取txt文件内的坐标,并对这些坐标进行后续处理。注意,这仅是一种基本的方法,具体的实现根据实际情况可能有所差异。
### 回答3:
使用OpenCV读取txt文件内的坐标可以通过以下步骤实现:
1. 首先,需要使用Python中的内置函数`open()`打开txt文件。可以使用以下代码打开文件,并将其存储在一个变量中:
```python
file = open('coordinates.txt', 'r')
```
这里使用'r'模式以只读方式打开文件。
2. 然后,可以使用`readlines()`方法读取txt文件中的所有行,并将其存储在一个列表中:
```python
lines = file.readlines()
```
3. 迭代遍历列表中的每一行,然后使用字符串`split()`方法将每一行按照空格或逗号分割成坐标点。可以使用以下代码实现:
```python
coordinates = []
for line in lines:
data = line.split() # 利用空格或逗号分割每一行
x = int(data[0]) # 将第一个字符串转换为整数作为x坐标
y = int(data[1]) # 将第二个字符串转换为整数作为y坐标
coordinates.append((x, y)) # 将每一行的坐标点添加到列表中
```
4. 最后,可以使用OpenCV中的函数和方法处理这些坐标。这取决于具体的需求,可以在图像上绘制这些坐标点,或者进行其他处理。
完整的代码示例如下:
```python
import cv2
file = open('coordinates.txt', 'r')
lines = file.readlines()
coordinates = []
for line in lines:
data = line.split()
x = int(data[0])
y = int(data[1])
coordinates.append((x, y))
# 在图像上绘制坐标
image = cv2.imread('image.jpg')
for point in coordinates:
cv2.circle(image, point, 5, (0, 255, 0), -1)
cv2.imshow('Image', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
以上代码将读取`coordinates.txt`文件中的坐标,并在一张名为`image.jpg`的图像上绘制这些坐标点。
相关推荐
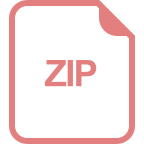














