用c语言链表求两多项式相乘
时间: 2024-11-18 10:16:35 浏览: 27
在C语言中,可以使用链表来表示多项式的系数和指数,然后通过遍历两个多项式链表来进行相乘。这里是一种基本的步骤:
1. 定义一个结构体表示节点,包含一个数值(系数)和一个指向下一个节点的指针(通常为NULL表示这是一个常数项):
```c
typedef struct {
int coefficient;
struct Node* next;
} Term;
```
2. 创建函数来创建新节点并初始化它们:
```c
Term* createNode(int coeff) {
Term* newNode = (Term*)malloc(sizeof(Term));
newNode->coefficient = coeff;
newNode->next = NULL;
return newNode;
}
```
3. 对于每个多项式,从最高次幂开始,计算当前项的乘积,并添加到结果链表中。这涉及迭代两个链表,将对应项相乘并处理可能产生的更高次幂:
```c
void multiplyPolynomials(Term** poly1, Term** poly2, Term** result) {
int i = getDegree(*poly1) - 1; // 获取第一个多项式的最高次幂
int j = getDegree(*poly2) - 1; // 获取第二个多项式的最高次幂
while (i >= 0 && j >= 0) {
Term* term1 = *poly1;
Term* term2 = *poly2;
int coefficientProduct = term1->coefficient * term2->coefficient;
int exponentSum = i + j;
// 添加新的项到结果链表
if (exponentSum > 0) {
Term* newTerm = createNode(coefficientProduct);
newTerm->next = *result;
*result = newTerm;
}
// 移动到下一项
if (term1->next != NULL)
term1 = term1->next;
if (term2->next != NULL)
term2 = term2->next;
i--;
j--;
}
}
```
4. 最后,你可以递归地获取链表的度(degree),如果链表为空,则返回0:
```c
int getDegree(Term* poly) {
if (poly == NULL)
return 0;
else
return 1 + getDegree(poly->next);
}
```
阅读全文
相关推荐
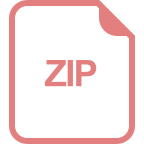
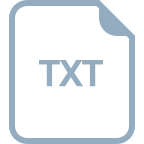
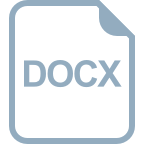

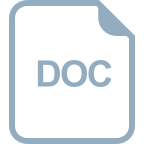

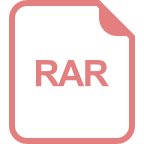



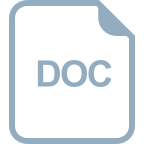
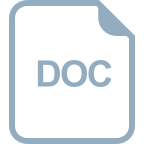


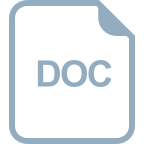