采用c语言链表实现多项式相乘
时间: 2024-04-05 07:27:59 浏览: 152
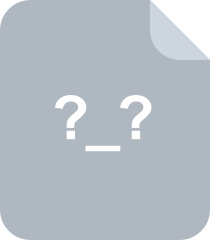
链表实现多项式加法和乘法(C语言实现)

采用C语言链表实现多项式相乘的基本思路是将两个多项式分别表示为链表的形式,然后按照多项式相乘的规则进行计算,并将结果保存在一个新的链表中。
具体实现步骤如下:
1. 定义一个结构体来表示多项式的每一项,包括系数和指数。
```c
typedef struct Node {
int coefficient; // 系数
int exponent; // 指数
struct Node* next; // 指向下一个节点的指针
} Node;
```
2. 创建两个链表,分别表示两个多项式,并初始化为空链表。
```c
Node* poly1 = NULL; // 第一个多项式链表
Node* poly2 = NULL; // 第二个多项式链表
```
3. 分别读取两个多项式的系数和指数,并将其插入到对应的链表中。
```c
// 插入节点到链表中
void insertNode(Node** head, int coefficient, int exponent) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->coefficient = coefficient;
newNode->exponent = exponent;
newNode->next = NULL;
if (*head == NULL) {
*head = newNode;
} else {
Node* temp = *head;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = newNode;
}
}
// 读取多项式的系数和指数,并插入到链表中
void readPolynomial(Node** head) {
int coefficient, exponent;
int numTerms;
printf("请输入多项式的项数:");
scanf("%d", &numTerms);
for (int i = 0; i < numTerms; i++) {
printf("请输入第%d项的系数和指数:", i + 1);
scanf("%d %d", &coefficient, &exponent);
insertNode(head, coefficient, exponent);
}
}
// 读取第一个多项式
printf("请输入第一个多项式:\n");
readPolynomial(&poly1);
// 读取第二个多项式
printf("请输入第二个多项式:\n");
readPolynomial(&poly2);
```
4. 定义一个函数来实现多项式相乘的操作,并返回结果链表。
```c
// 多项式相乘
Node* multiplyPolynomials(Node* poly1, Node* poly2) {
Node* result = NULL; // 结果链表
// 遍历第一个多项式的每一项
Node* temp1 = poly1;
while (temp1 != NULL) {
// 遍历第二个多项式的每一项
Node* temp2 = poly2;
while (temp2 != NULL) {
// 计算相乘后的系数和指数
int coefficient = temp1->coefficient * temp2->coefficient;
int exponent = temp1->exponent + temp2->exponent;
// 在结果链表中插入相乘后的项
insertNode(&result, coefficient, exponent);
temp2 = temp2->next;
}
temp1 = temp1->next;
}
return result;
}
// 调用多项式相乘函数
Node* result = multiplyPolynomials(poly1, poly2);
```
5. 最后,遍历结果链表并输出相乘后的多项式。
```c
// 遍历链表并输出多项式
void printPolynomial(Node* head) {
Node* temp = head;
while (temp != NULL) {
printf("%dx^%d ", temp->coefficient, temp->exponent);
if (temp->next != NULL) {
printf("+ ");
}
temp = temp->next;
}
printf("\n");
}
// 输出相乘后的多项式
printf("相乘后的多项式为:");
printPolynomial(result);
```
以上就是采用C语言链表实现多项式相乘的基本步骤。希望对你有所帮助!
阅读全文
相关推荐
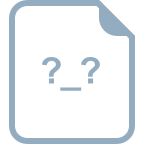
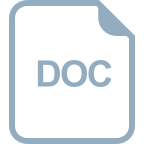

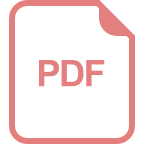
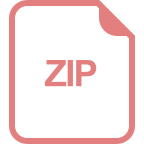
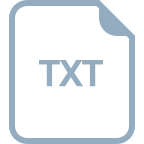
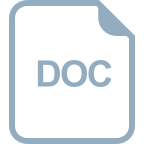
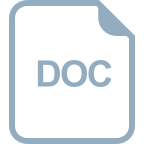


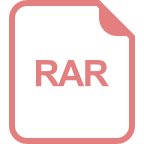
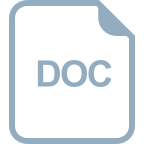
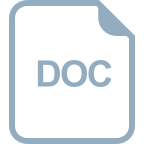
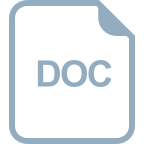
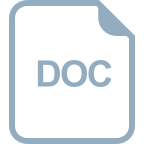
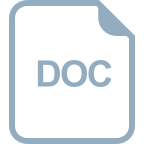
