C语言编写数据结构一元多项式计算器,输入多项式系数和指数,排序使其按指数升序排列,计算相加相减相乘结果,并将结果输出
时间: 2023-12-10 16:06:21 浏览: 31
好的,下面是一个简单的 C 语言代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
struct Node{
float coef;
int expn;
struct Node *next;
};
void createPoly(struct Node **head, int n);
void sortPoly(struct Node *head);
void addPoly(struct Node *p1, struct Node *p2, struct Node **res);
void subPoly(struct Node *p1, struct Node *p2, struct Node **res);
void mulPoly(struct Node *p1, struct Node *p2, struct Node **res);
void printPoly(struct Node *head);
int main() {
struct Node *poly1 = NULL, *poly2 = NULL, *addRes = NULL, *subRes = NULL, *mulRes = NULL;
int n1, n2;
printf("请输入第一个多项式的项数:");
scanf("%d", &n1);
createPoly(&poly1, n1);
sortPoly(poly1);
printf("请输入第二个多项式的项数:");
scanf("%d", &n2);
createPoly(&poly2, n2);
sortPoly(poly2);
addPoly(poly1, poly2, &addRes);
subPoly(poly1, poly2, &subRes);
mulPoly(poly1, poly2, &mulRes);
printf("\n第一个多项式为:");
printPoly(poly1);
printf("\n第二个多项式为:");
printPoly(poly2);
printf("\n相加结果为:");
printPoly(addRes);
printf("\n相减结果为:");
printPoly(subRes);
printf("\n相乘结果为:");
printPoly(mulRes);
return 0;
}
void createPoly(struct Node **head, int n) {
*head = (struct Node*)malloc(sizeof(struct Node));
(*head)->next = NULL;
struct Node *p = *head;
for (int i = 0; i < n; i++) {
float coef;
int expn;
printf("请输入第 %d 项的系数和指数:", i + 1);
scanf("%f %d", &coef, &expn);
struct Node *newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->coef = coef;
newNode->expn = expn;
p->next = newNode;
p = p->next;
}
p->next = NULL;
}
void sortPoly(struct Node *head) {
struct Node *p, *q;
float coef;
int expn;
for (p = head->next; p != NULL; p = p->next) {
for (q = p->next; q != NULL; q = q->next) {
if (p->expn > q->expn) {
coef = p->coef;
expn = p->expn;
p->coef = q->coef;
p->expn = q->expn;
q->coef = coef;
q->expn = expn;
}
}
}
}
void addPoly(struct Node *p1, struct Node *p2, struct Node **res) {
struct Node *p = p1->next, *q = p2->next;
float coef;
int expn;
*res = (struct Node*)malloc(sizeof(struct Node));
(*res)->next = NULL;
struct Node *r = *res;
while (p != NULL && q != NULL) {
if (p->expn == q->expn) {
coef = p->coef + q->coef;
expn = p->expn;
p = p->next;
q = q->next;
} else if (p->expn > q->expn) {
coef = p->coef;
expn = p->expn;
p = p->next;
} else {
coef = q->coef;
expn = q->expn;
q = q->next;
}
struct Node *newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->coef = coef;
newNode->expn = expn;
r->next = newNode;
r = r->next;
}
while (p != NULL) {
coef = p->coef;
expn = p->expn;
p = p->next;
struct Node *newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->coef = coef;
newNode->expn = expn;
r->next = newNode;
r = r->next;
}
while (q != NULL) {
coef = q->coef;
expn = q->expn;
q = q->next;
struct Node *newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->coef = coef;
newNode->expn = expn;
r->next = newNode;
r = r->next;
}
r->next = NULL;
}
void subPoly(struct Node *p1, struct Node *p2, struct Node **res) {
struct Node *p = p1->next, *q = p2->next;
float coef;
int expn;
*res = (struct Node*)malloc(sizeof(struct Node));
(*res)->next = NULL;
struct Node *r = *res;
while (p != NULL && q != NULL) {
if (p->expn == q->expn) {
coef = p->coef - q->coef;
expn = p->expn;
p = p->next;
q = q->next;
} else if (p->expn > q->expn) {
coef = p->coef;
expn = p->expn;
p = p->next;
} else {
coef = -q->coef;
expn = q->expn;
q = q->next;
}
struct Node *newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->coef = coef;
newNode->expn = expn;
r->next = newNode;
r = r->next;
}
while (p != NULL) {
coef = p->coef;
expn = p->expn;
p = p->next;
struct Node *newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->coef = coef;
newNode->expn = expn;
r->next = newNode;
r = r->next;
}
while (q != NULL) {
coef = -q->coef;
expn = q->expn;
q = q->next;
struct Node *newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->coef = coef;
newNode->expn = expn;
r->next = newNode;
r = r->next;
}
r->next = NULL;
}
void mulPoly(struct Node *p1, struct Node *p2, struct Node **res) {
struct Node *p = p1->next, *q = p2->next;
float coef;
int expn;
*res = (struct Node*)malloc(sizeof(struct Node));
(*res)->next = NULL;
struct Node *r = *res, *t;
while (p != NULL) {
q = p2->next;
while (q != NULL) {
coef = p->coef * q->coef;
expn = p->expn + q->expn;
t = r->next;
while (t != NULL && t->expn < expn) {
r = t;
t = t->next;
}
if (t != NULL && t->expn == expn) {
t->coef += coef;
} else {
struct Node *newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->coef = coef;
newNode->expn = expn;
newNode->next = t;
r->next = newNode;
}
q = q->next;
}
p = p->next;
}
}
void printPoly(struct Node *head) {
struct Node *p = head->next;
while (p != NULL) {
if (p->expn == 0) {
printf("%.2f", p->coef);
} else if (p->expn == 1) {
printf("%.2fx", p->coef);
} else {
printf("%.2fx^%d", p->coef, p->expn);
}
p = p->next;
if (p != NULL) {
printf(" + ");
}
}
}
```
这个程序中,我们定义了一个结构体 `Node` 来表示一个多项式的每一项,这个结构体包含两个成员:系数 `coef` 和指数 `expn`。我们使用一个链表来存储多项式,链表的头结点不存储数据,头结点的下一个结点开始存储多项式的第一项。
在 `createPoly()` 函数中,我们可以输入多项式的每一项的系数和指数,然后将这些项存储到链表中,最后调用 `sortPoly()` 函数将链表中的项按指数升序排列。
在 `addPoly()` 函数中,我们首先将两个多项式的第一项相加,然后比较两个多项式当前项的指数大小,将较小的那个多项式的当前项加入结果链表中,并前进一项,直到其中一个多项式遍历完。最后将剩余的项加入结果链表中。
在 `subPoly()` 函数中,我们实现的方法与 `addPoly()` 函数类似,只是将两个多项式的当前项相减。
在 `mulPoly()` 函数中,我们首先遍历第一个多项式的每一项,然后遍历第二个多项式的每一项,计算两项的乘积,并将结果插入到结果链表中,最后将结果链表中的项按指数升序排列。
在 `printPoly()` 函数中,我们输出多项式的每一项,如果指数为 0,则只输出系数,如果指数为 1,则输出 `x`,否则输出 `x^指数`。
以上就是一个简单的 C 语言代码实现。
相关推荐
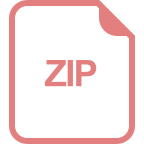
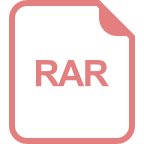
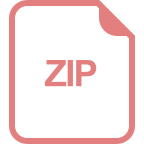














