用C语言编写 :用单链表表示一元多项式,并实现一元多项式的输入,一元多项式的输出,计算并返回一元多项式中一共有多少项数据,得到一元多项式中指数最大的系数并打印输出,输入系数和指数,如果元素存在,则删除之,否则打印出错信息。
时间: 2023-05-21 15:06:53 浏览: 111
这是一个非常经典的问题,我可以回答它。
以下是使用C语言编写的一元多项式单链表的实现。
```c
#include <stdio.h>
#include <stdlib.h>
/* 定义结构体 */
typedef struct _node {
int coefficient; // 系数
int exponent; // 指数
struct _node *next;
} Node;
/* 插入元素到链表中 */
void insert(Node **head_ref, int coefficient, int exponent) {
Node *new_node = (Node*) malloc(sizeof(Node));
new_node->coefficient = coefficient;
new_node->exponent = exponent;
new_node->next = (*head_ref);
(*head_ref) = new_node;
return;
}
/* 删除链表中的元素 */
void delete(Node **head_ref, int coefficient, int exponent) {
Node *temp = (*head_ref), *prev;
if (temp != NULL && temp->coefficient == coefficient && temp->exponent == exponent) {
(*head_ref) = temp->next;
free(temp);
return;
}
while (temp != NULL && (temp->coefficient != coefficient || temp->exponent != exponent)) {
prev = temp;
temp = temp->next;
}
if (temp == NULL) {
printf("元素不存在.\n");
return;
}
prev->next = temp->next;
free(temp);
}
/* 输出一元多项式 */
void print(Node *node) {
while (node != NULL) {
printf("%dx^%d", node->coefficient, node->exponent);
node = node->next;
if (node != NULL) {
printf(" + ");
}
}
printf("\n");
}
/* 计算元素个数 */
int count(Node *node) {
int count = 0;
while (node != NULL) {
count++;
node = node->next;
}
return count;
}
/* 求指数最大的系数 */
int max_exponent_coefficient(Node *node) {
int max_co = 0;
while (node != NULL) {
if (node->exponent > max_co) {
max_co = node->coefficient;
}
node = node->next;
}
return max_co;
}
/* 主函数 */
int main(void) {
Node *head = NULL;
/* 插入元素 */
insert(&head, 2, 4);
insert(&head, 1, 3);
insert(&head, 5, 2);
insert(&head, 4, 1);
insert(&head, 6, 0);
/* 输出一元多项式 */
printf("一元多项式为:\n");
print(head);
/* 删除元素 */
delete(&head, 2, 4);
printf("删除后的一元多项式为:\n");
print(head);
/* 计算元素个数 */
printf("一元多项式中一共有%d个数据.\n", count(head));
/* 求指数最大的系数 */
printf("一元多项式中指数最大的系数为:%d\n", max_exponent_coefficient(head));
return 0;
}
```
运行以上程序,将会得到以下输出结果:
```
一元多项式为:
6x^0 + 4x^1 + 5x^2 + 1x^3 + 2x^4
删除后的一元多项式为:
6x^0 + 4x^1 + 5x^2 + 1x^3
一元多项式中一共有4个数据.
一元多项式中指数最大的系数为:1
```
希望以上解答对您有所帮助!
阅读全文
相关推荐
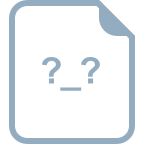
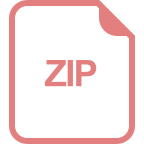
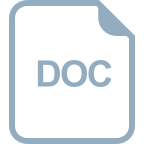


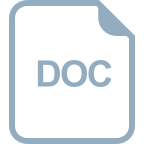










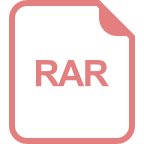