请写一个linux PC端的串口通信程序要求对接收数据进行解析,还要实现发送
时间: 2024-04-11 19:33:40 浏览: 22
对于一个 Linux PC 端的串口通信程序,你可以使用 C/C++ 编程语言来实现。下面是一个简单的示例代码,可以实现接收数据的解析和发送功能:
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <termios.h>
#include <unistd.h>
#define SERIAL_PORT "/dev/ttyUSB0" // 串口设备路径
int open_serial_port() {
int fd = open(SERIAL_PORT, O_RDWR | O_NOCTTY | O_NDELAY);
if (fd == -1) {
perror("无法打开串口设备");
exit(EXIT_FAILURE);
}
return fd;
}
void configure_serial_port(int fd) {
struct termios options;
tcgetattr(fd, &options);
cfsetispeed(&options, B9600); // 设置波特率为9600
cfsetospeed(&options, B9600);
options.c_cflag |= (CLOCAL | CREAD);
options.c_cflag &= ~PARENB; // 无奇偶校验
options.c_cflag &= ~CSTOPB; // 1位停止位
options.c_cflag &= ~CSIZE; // 8位数据位
options.c_cflag |= CS8;
options.c_cc[VMIN] = 1; // 读取最少一个字符
options.c_cc[VTIME] = 0; // 等待时间为0
tcsetattr(fd, TCSANOW, &options);
}
void receive_data(int fd) {
char buffer[255];
int bytesRead = read(fd, buffer, sizeof(buffer));
if (bytesRead > 0) {
// 对接收的数据进行解析或处理
printf("接收到数据:%.*s\n", bytesRead, buffer);
}
}
void send_data(int fd, const char* data) {
write(fd, data, strlen(data));
}
int main() {
int fd = open_serial_port();
configure_serial_port(fd);
while (1) {
receive_data(fd);
// 在这里可以根据需要发送数据
send_data(fd, "Hello, World!\n");
usleep(100000); // 稍微延时一下
}
close(fd);
return 0;
}
```
请确保将 `SERIAL_PORT` 宏定义设置为正确的串口设备路径,例如 `/dev/ttyUSB0`。该示例程序会循环接收数据并解析,同时发送一条固定的消息。你可以根据实际需求进行修改和扩展。
相关推荐
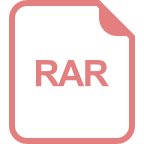
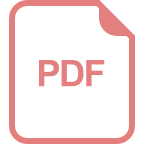
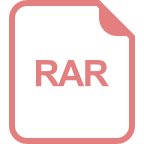
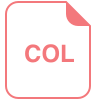
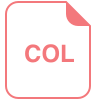
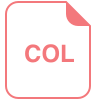
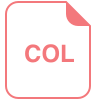
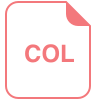









