帮我用C语言写一个生产者消费者模型
时间: 2023-04-01 13:01:40 浏览: 82
好的,以下是一个简单的生产者消费者模型的 C 语言代码:
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define BUFFER_SIZE 10
int buffer[BUFFER_SIZE];
int in = , out = ;
pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER;
pthread_cond_t empty = PTHREAD_COND_INITIALIZER;
pthread_cond_t full = PTHREAD_COND_INITIALIZER;
void *producer(void *arg) {
int i;
for (i = ; i < 20; i++) {
pthread_mutex_lock(&mutex);
while ((in + 1) % BUFFER_SIZE == out) {
pthread_cond_wait(&empty, &mutex);
}
buffer[in] = i;
in = (in + 1) % BUFFER_SIZE;
pthread_cond_signal(&full);
pthread_mutex_unlock(&mutex);
}
pthread_exit(NULL);
}
void *consumer(void *arg) {
int i, data;
for (i = ; i < 20; i++) {
pthread_mutex_lock(&mutex);
while (in == out) {
pthread_cond_wait(&full, &mutex);
}
data = buffer[out];
out = (out + 1) % BUFFER_SIZE;
pthread_cond_signal(&empty);
pthread_mutex_unlock(&mutex);
printf("Consumer: %d\n", data);
}
pthread_exit(NULL);
}
int main() {
pthread_t producer_thread, consumer_thread;
pthread_create(&producer_thread, NULL, producer, NULL);
pthread_create(&consumer_thread, NULL, consumer, NULL);
pthread_join(producer_thread, NULL);
pthread_join(consumer_thread, NULL);
return ;
}
阅读全文
相关推荐
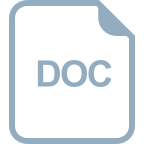
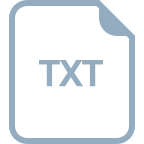
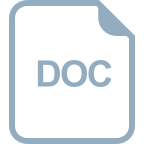
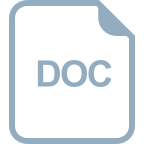
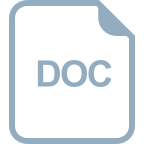
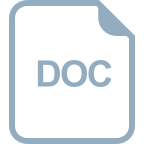
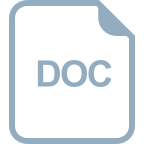
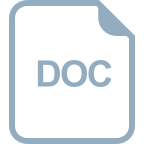
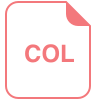





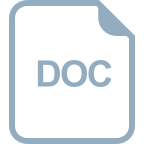
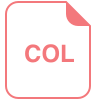

