给我一个C语言的生产者和消费者模型
时间: 2023-06-07 12:08:55 浏览: 110
好的,这是一个基本的 C 语言生产者和消费者模型:
```
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <semaphore.h>
#define BUFFER_SIZE 5
int buffer[BUFFER_SIZE];
int next_in = 0, next_out = 0;
sem_t empty, full;
pthread_mutex_t mutex;
void *producer(void *args) {
int i;
for (i = 0; i < 10; i++) {
sem_wait(&empty);
pthread_mutex_lock(&mutex);
buffer[next_in] = i;
printf("Producer produced %d\n", i);
next_in = (next_in + 1) % BUFFER_SIZE;
pthread_mutex_unlock(&mutex);
sem_post(&full);
}
pthread_exit(NULL);
}
void *consumer(void *args) {
int i, data;
for (i = 0; i < 10; i++) {
sem_wait(&full);
pthread_mutex_lock(&mutex);
data = buffer[next_out];
printf("Consumer consumed %d\n", data);
next_out = (next_out + 1) % BUFFER_SIZE;
pthread_mutex_unlock(&mutex);
sem_post(&empty);
}
pthread_exit(NULL);
}
int main() {
pthread_t prod_thread, cons_thread;
sem_init(&empty, 0, BUFFER_SIZE);
sem_init(&full, 0, 0);
pthread_mutex_init(&mutex, NULL);
pthread_create(&prod_thread, NULL, producer, NULL);
pthread_create(&cons_thread, NULL, consumer, NULL);
pthread_join(prod_thread, NULL);
pthread_join(cons_thread, NULL);
sem_destroy(&empty);
sem_destroy(&full);
pthread_mutex_destroy(&mutex);
return 0;
}
```
这个模型使用两个线程:一个生产者,一个消费者。它们对一个固定大小的循环缓冲区进行操作。生产者线程将数字写入缓冲区,消费者线程从缓冲区读取数字。如果缓冲区满了,生产者会等待,直到消费者从缓冲区中取出一些数据。如果缓冲区为空,消费者会等待,直到生产者放入一些数据。
这个模型需要使用互斥锁和信号量来保证线程之间的同步和互斥。互斥锁用于保护共享资源,即缓冲区和计数器。信号量用于同步线程之间的操作,即当缓冲区上有空间时通知生产者线程,当缓冲区上有数据时通知消费者线程。
阅读全文
相关推荐
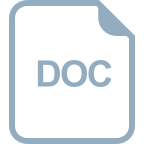
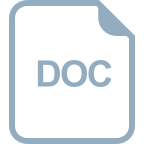
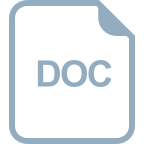
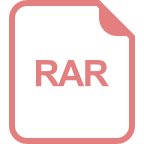
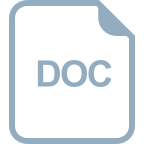
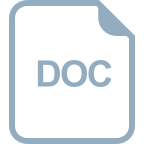
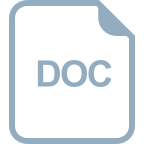
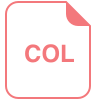
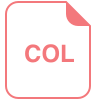









