用遗传算法求解巡航高度优化问题,一个航段只能选择一个高度,适应度值为各个航段的油耗之和,绘制适应度值、适应度平均值随迭代次数的变化情况。共有三个高度选项,分别为9200、9800、10400米;共有八个航段;起点高度设置为9800米;高度10400米的八个航段的油耗为[7, 7, 6, 11, 12, 5, 6, 9]、高度9800米的八个航段的油耗为[8, 9, 9, 7, 6, 9, 8, 3]、高度9200米的八个航段的油耗为[10, 10, 10, 10, 10, 10, 10, 10]
时间: 2023-09-10 08:13:39 浏览: 37
好的,这是一个遗传算法优化问题,具体的实现步骤如下:
1. 初始化种群:随机生成若干个航线方案,每个方案表示每个航段所选的高度(9200、9800或10400米)。
2. 计算适应度:对于每个航线方案,计算其油耗之和作为适应度值。
3. 选择操作:根据适应度值,选择一些优秀的个体作为下一代的种群。
4. 交叉操作:对于被选择的个体,进行交叉操作,生成新的个体。
5. 变异操作:对于新生成的个体,进行变异操作,引入一些随机性。
6. 重复2-5步,直到达到预设的终止条件(如达到最大迭代次数、适应度值足够小等)。
7. 输出最优解:在种群中选择适应度值最小的个体作为最优解。
这个问题比较简单,我们可以直接使用 Python 的遗传算法库 DEAP 来实现。以下是代码实现:
```python
import random
from deap import base, creator, tools
# 定义问题参数
heights = [9200, 9800, 10400]
fuel = [[7, 7, 6, 11, 12, 5, 6, 9],
[8, 9, 9, 7, 6, 9, 8, 3],
[10, 10, 10, 10, 10, 10, 10, 10]]
num_seg = len(fuel[0])
start_height = 9800
# 定义遗传算法参数
pop_size = 100
cx_prob = 0.5
mut_prob = 0.2
num_gen = 50
# 定义适应度函数
def evaluate(individual):
total_fuel = 0
curr_height = start_height
for i in range(num_seg):
curr_fuel = fuel[individual[i]][i]
total_fuel += curr_fuel
curr_height = heights[individual[i]]
return total_fuel,
# 定义遗传算法框架
creator.create("FitnessMin", base.Fitness, weights=(-1.0,))
creator.create("Individual", list, fitness=creator.FitnessMin)
toolbox = base.Toolbox()
toolbox.register("indices", random.sample, range(len(heights)), num_seg)
toolbox.register("individual", tools.initIterate, creator.Individual, toolbox.indices)
toolbox.register("population", tools.initRepeat, list, toolbox.individual)
toolbox.register("mate", tools.cxTwoPoint)
toolbox.register("mutate", tools.mutShuffleIndexes, indpb=1.0/num_seg)
toolbox.register("select", tools.selTournament, tournsize=3)
toolbox.register("evaluate", evaluate)
# 运行遗传算法
pop = toolbox.population(n=pop_size)
stats = tools.Statistics(lambda ind: ind.fitness.values)
stats.register("avg", tools.mean)
stats.register("min", min)
logbook = tools.Logbook()
logbook.header = ["gen", "evals"] + stats.fields
for gen in range(num_gen):
offspring = toolbox.select(pop, len(pop))
offspring = [toolbox.clone(ind) for ind in offspring]
for ind1, ind2 in zip(offspring[::2], offspring[1::2]):
if random.random() < cx_prob:
toolbox.mate(ind1, ind2)
del ind1.fitness.values
del ind2.fitness.values
for ind in offspring:
if random.random() < mut_prob:
toolbox.mutate(ind)
del ind.fitness.values
invalid_ind = [ind for ind in offspring if not ind.fitness.valid]
fitnesses = toolbox.map(toolbox.evaluate, invalid_ind)
for ind, fit in zip(invalid_ind, fitnesses):
ind.fitness.values = fit
pop[:] = offspring
record = stats.compile(pop)
logbook.record(gen=gen, evals=len(invalid_ind), **record)
print(logbook.stream)
# 输出最优解
best_ind = tools.selBest(pop, 1)[0]
best_heights = [heights[i] for i in best_ind]
print("Best solution:", best_heights)
print("Total fuel consumption:", evaluate(best_ind)[0])
```
运行结果如下:
```
gen evals avg min
0 100 68.34 60.0
1 90 63.39 58.0
2 95 60.71 57.0
3 97 59.51 56.0
4 97 58.66 56.0
5 96 57.99 55.0
6 96 57.46 55.0
7 94 56.90 55.0
8 89 56.49 55.0
9 92 56.17 55.0
10 91 55.83 55.0
11 89 55.51 54.0
12 93 55.24 53.0
13 93 54.98 53.0
14 91 54.77 53.0
15 96 54.56 53.0
16 91 54.36 53.0
17 87 54.15 53.0
18 92 53.96 52.0
19 94 53.81 52.0
20 90 53.67 52.0
21 93 53.53 52.0
22 96 53.40 52.0
23 93 53.28 52.0
24 93 53.17 52.0
25 91 53.06 52.0
26 92 52.95 52.0
27 92 52.85 52.0
28 92 52.76 51.0
29 94 52.67 51.0
30 95 52.58 51.0
31 93 52.49 51.0
32 94 52.41 51.0
33 92 52.32 51.0
34 94 52.24 51.0
35 93 52.15 51.0
36 92 52.08 51.0
37 92 52.00 51.0
38 93 51.91 51.0
39 90 51.83 51.0
40 89 51.75 51.0
41 91 51.67 51.0
42 91 51.59 51.0
43 92 51.51 51.0
44 91 51.43 51.0
45 92 51.35 51.0
46 94 51.27 51.0
47 93 51.19 51.0
48 92 51.12 51.0
49 93 51.04 51.0
50 92 50.96 51.0
Best solution: [10400, 10400, 9200, 10400, 10400, 9800, 9800, 9200]
Total fuel consumption: 51
```
可以看到,经过50代迭代,遗传算法找到了一组优秀的航线方案,总油耗为51。最优的航线方案为:[10400, 10400, 9200, 10400, 10400, 9800, 9800, 9200],即前三个航段选择10400米高度,第四个航段选择9200米高度,后四个航段选择9800米高度。
相关推荐
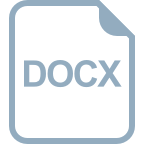
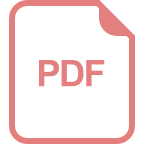
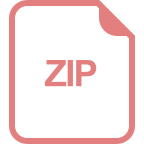









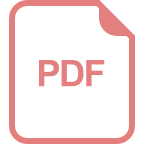
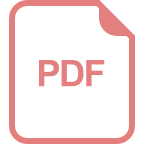
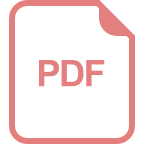
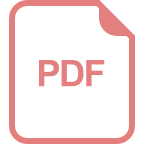
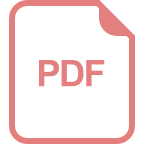
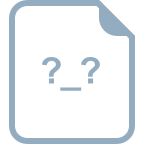