c语言根据公告中发布参考文献,构建5个学生构成链表,完成插入,删除和遍历链表元素的功能。 struct Student{ int num; char name[20]; float score; }
时间: 2024-12-06 08:22:05 浏览: 9
在C语言中,为了实现一个包含5个学生的链表结构,并提供插入、删除和遍历功能,你需要首先定义`Student`结构体和一个节点结构`Node`,然后创建链表头指针。以下是关键步骤:
1. **定义结构体**:
```c
struct Node {
struct Student student;
struct Node *next; // 指向下一个节点的指针
};
struct Student {
int num;
char name[20];
float score;
};
```
2. **初始化链表**:
```c
struct Node* head = NULL; // 初始链表为空
```
3. **插入操作**:
- 插入到头部:
```c
void insertAtStart(struct Node** head, struct Student newStudent) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->student = newStudent;
newNode->next = *head;
*head = newNode;
}
```
- 插入到指定位置:
```c
void insertAtPosition(struct Node** head, int position, struct Student newStudent) {
if (position <= 0) return; // 防止越界
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->student = newStudent;
newNode->next = NULL;
if (*head == NULL || position == 1) {
newNode->next = *head;
*head = newNode;
return;
}
struct Node* current = *head;
for (int i = 1; i < position - 1 && current != NULL; ++i) {
current = current->next;
}
if (current != NULL)
newNode->next = current->next;
current->next = newNode;
}
```
4. **删除操作**:
- 删除头部节点:
```c
void deleteFromStart(struct Node** head) {
if (*head != NULL) {
struct Node* temp = *head;
*head = (*head)->next;
free(temp);
}
}
// 删除指定位置节点
void deleteFromPosition(struct Node** head, int position) {
if (*head == NULL || position <= 0) return;
if (position == 1) {
deleteFromStart(head);
return;
}
struct Node* current = *head;
for (int i = 1; i < position && current != NULL; ++i) {
current = current->next;
}
if (current == NULL) return;
struct Node* temp = current->next;
current->next = temp->next;
free(temp);
}
```
5. **遍历链表**:
```c
void traverseList(struct Node* head) {
if (head == NULL) {
printf("链表为空.\n");
} else {
struct Node* temp = head;
while (temp != NULL) {
printf("学号: %d, 姓名: %s, 成绩: %.2f\n", temp->student.num, temp->student.name, temp->student.score);
temp = temp->next;
}
}
}
```
使用以上函数时,记得处理内存分配失败的情况。现在你可以根据需要构造和管理这个学生链表了。
阅读全文
相关推荐
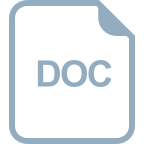
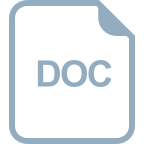
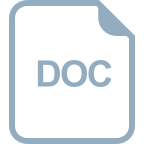















