c语言写一个函数用来向一个动态链表插入节点规定插入到链表的尾部。函数原型为void insert(struct Student **head, struct Student *ins)
时间: 2023-06-20 09:03:40 浏览: 99
下面是一个示例代码,实现了向链表尾部插入节点的功能:
```c
#include <stdio.h>
#include <stdlib.h>
struct Student {
int id;
char name[20];
struct Student *next;
};
void insert(struct Student **head, struct Student *ins) {
if (*head == NULL) { // 如果链表为空,则将节点插入头部
*head = ins;
} else {
struct Student *p = *head;
while (p->next != NULL) { // 找到链表尾部
p = p->next;
}
p->next = ins; // 将节点插入尾部
}
}
int main() {
struct Student *head = NULL; // 定义链表头指针
struct Student *p;
// 向链表中插入三个节点
for (int i = 1; i <= 3; i++) {
p = (struct Student*)malloc(sizeof(struct Student));
p->id = i;
sprintf(p->name, "Student%d", i);
p->next = NULL;
insert(&head, p);
}
// 遍历链表并输出每个节点的信息
p = head;
while (p != NULL) {
printf("ID: %d, Name: %s\n", p->id, p->name);
p = p->next;
}
return 0;
}
```
在该示例代码中,`insert`函数用来向链表插入节点。该函数接受两个参数,第一个参数是链表头指针的地址,第二个参数是要插入的节点指针。如果链表为空,则将节点插入头部;否则,找到链表尾部,将节点插入尾部。
在`main`函数中,我们向链表中插入了三个节点,并遍历链表输出每个节点的信息。
阅读全文
相关推荐
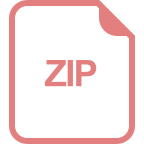

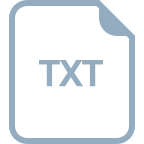
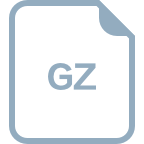
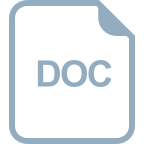
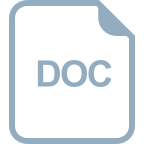
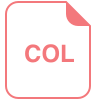
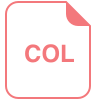








