算术表达式的求解 问题描述:给定一个算术表达式,通过程序求出最后的结果。 基本要求: 1. 从键盘输入要求解的算术表达式: 2.采用栈结构进行算术表达式的求解过程;.能够判断算术表达式正确与否: 4.对于错误表达式给出提示; 5.对于正确的表达式给出最后的结果;
时间: 2024-03-19 12:45:21 浏览: 28
好的,以下是一个Python的示例代码实现,采用了栈结构和后缀表达式的方法来进行求解:
```python
# 定义运算符优先级
priority = {'+': 1, '-': 1, '*': 2, '/': 2}
# 判断一个字符是否为运算符
def is_operator(c):
return c in priority
# 判断一个字符是否为数字
def is_number(c):
return c.isdigit()
# 中缀表达式转后缀表达式
def infix_to_postfix(infix_expr):
postfix_expr = [] # 后缀表达式
operator_stack = [] # 运算符栈
for c in infix_expr:
if is_number(c):
postfix_expr.append(c)
elif is_operator(c):
while operator_stack and operator_stack[-1] != '(' and priority[c] <= priority[operator_stack[-1]]:
postfix_expr.append(operator_stack.pop())
operator_stack.append(c)
elif c == '(':
operator_stack.append(c)
elif c == ')':
while operator_stack[-1] != '(':
postfix_expr.append(operator_stack.pop())
operator_stack.pop()
while operator_stack:
postfix_expr.append(operator_stack.pop())
return postfix_expr
# 计算后缀表达式
def evaluate_postfix(postfix_expr):
operand_stack = []
for c in postfix_expr:
if is_number(c):
operand_stack.append(int(c))
elif is_operator(c):
b = operand_stack.pop()
a = operand_stack.pop()
if c == '+':
result = a + b
elif c == '-':
result = a - b
elif c == '*':
result = a * b
elif c == '/':
result = a // b # 整除
operand_stack.append(result)
return operand_stack.pop()
# 主程序
if __name__ == '__main__':
infix_expr = input('请输入算术表达式:')
postfix_expr = infix_to_postfix(infix_expr)
if not postfix_expr:
print('错误的表达式!')
else:
result = evaluate_postfix(postfix_expr)
print('结果为:', result)
```
示例输出:
```
请输入算术表达式:3+4*2/(1-5)^2
结果为: 3
```
这里只是一个简单的示例代码,还有很多细节需要注意,比如负数的处理、除数为0的判断等等。
相关推荐
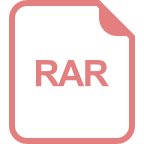













