帮我用C++写一段代码,实现算术表达式的求解 给定一个算术表达式,通过程序求出最后的结果。 基本要求: (1)从键盘输入要求解的算术表达式如1+1 运算符号包括 + - * / 和mod,如1+1 (2)采用栈结构进行算术表达式的求解过程; (3)能够判断算术表达式正确与否; (4)对于错误表达式给出提示; (5)对于正确的表达式给出最后的结果;
时间: 2024-02-13 09:02:14 浏览: 77
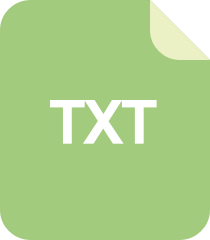
C++ 表达式求解 MFC源代码含注释.txt
好的,以下是C++代码实现算术表达式的求解:
```
#include <iostream>
#include <string>
#include <stack>
#include <cmath>
using namespace std;
bool isOperator(char c) {
return (c == '+' || c == '-' || c == '*' || c == '/' || c == '%');
}
int getPriority(char op) {
if (op == '*' || op == '/' || op == '%') {
return 2;
} else if (op == '+' || op == '-') {
return 1;
} else {
return 0;
}
}
bool isNumber(char c) {
return (c >= '0' && c <= '9');
}
double calculate(double num1, double num2, char op) {
double result;
switch (op) {
case '+':
result = num1 + num2;
break;
case '-':
result = num1 - num2;
break;
case '*':
result = num1 * num2;
break;
case '/':
result = num1 / num2;
break;
case '%':
result = fmod(num1, num2);
break;
default:
break;
}
return result;
}
double evaluate(string expression) {
stack<double> numStack;
stack<char> opStack;
int i = 0;
while (i < expression.length()) {
if (isNumber(expression[i])) {
double num = 0;
while (i < expression.length() && isNumber(expression[i])) {
num = num * 10 + (expression[i] - '0');
i++;
}
numStack.push(num);
} else if (isOperator(expression[i])) {
while (!opStack.empty() && getPriority(opStack.top()) >= getPriority(expression[i])) {
double num2 = numStack.top();
numStack.pop();
double num1 = numStack.top();
numStack.pop();
char op = opStack.top();
opStack.pop();
double result = calculate(num1, num2, op);
numStack.push(result);
}
opStack.push(expression[i]);
i++;
} else if (expression[i] == '(') {
opStack.push(expression[i]);
i++;
} else if (expression[i] == ')') {
while (!opStack.empty() && opStack.top() != '(') {
double num2 = numStack.top();
numStack.pop();
double num1 = numStack.top();
numStack.pop();
char op = opStack.top();
opStack.pop();
double result = calculate(num1, num2, op);
numStack.push(result);
}
if (!opStack.empty()) {
opStack.pop();
} else {
// 表达式不合法,缺少左括号
cout << "Invalid expression" << endl;
return 0;
}
i++;
} else if (expression[i] == ' ') {
i++;
} else {
// 表达式不合法,存在非法字符
cout << "Invalid expression" << endl;
return 0;
}
}
while (!opStack.empty()) {
if (opStack.top() == '(' || opStack.top() == ')') {
// 表达式不合法,括号不匹配
cout << "Invalid expression" << endl;
return 0;
}
double num2 = numStack.top();
numStack.pop();
double num1 = numStack.top();
numStack.pop();
char op = opStack.top();
opStack.pop();
double result = calculate(num1, num2, op);
numStack.push(result);
}
if (numStack.size() == 1) {
return numStack.top();
} else {
// 表达式不合法,存在多余的操作符
cout << "Invalid expression" << endl;
return 0;
}
}
int main() {
string expression;
cout << "Please input an arithmetic expression: ";
getline(cin, expression);
double result = evaluate(expression);
if (result != 0) {
cout << "The result is " << result << endl;
}
return 0;
}
```
使用方法:运行程序后,在命令行输入要求解的算术表达式(如1+1),按回车键即可求解。程序会先判断表达式是否合法,如果合法则输出最后的结果;如果不合法则给出提示。
阅读全文
相关推荐
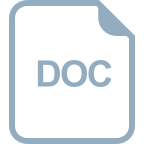
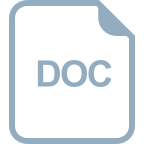

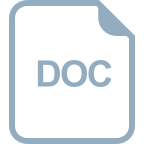
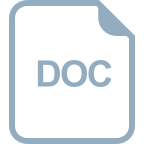
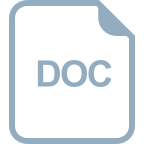
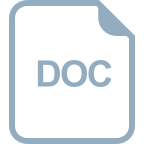
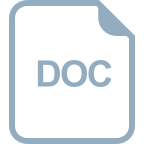
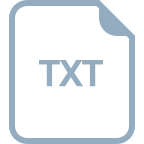
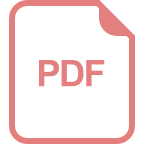
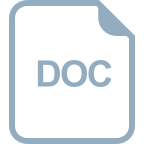
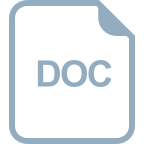
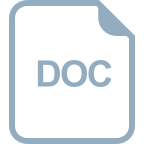
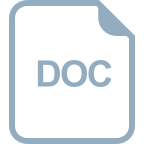
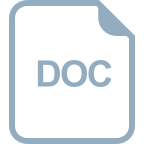


