商人过河问题python代码
时间: 2023-10-30 10:01:38 浏览: 130
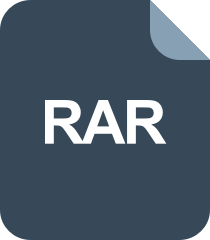
cccccccccccc.rar_c语言过河_人工智能_商人过河
下面是一个用Python编写的商人过河问题的解决方案:
```python
from itertools import combinations
def valid_state(state):
# 检查状态是否合法
if state[0] < state[1] and state[0] != 0:
return False
if state[2] < state[3] and state[2] != 0:
return False
return True
def solve():
# 初始状态:(商人数量, 马数量, 目的地)
initial_state = (3, 3, 1)
# 目标状态:(0, 0, 0)
target_state = (0, 0, 0)
# 使用广度优先搜索
queue = [(initial_state, [])]
visited = set([initial_state])
while queue:
state, actions = queue.pop(0)
if state == target_state:
return actions
m, c, b = state
# 根据当前状态生成可能的下一步动作
possible_actions = []
if b == 1:
possible_actions = [(x, y, 0) for x in range(m+1) for y in range(c+1) if 1 <= x + y <= 2]
else:
possible_actions = [(x, y, 1) for x in range(4-m, 4) for y in range(4-c, 4) if 1 <= x + y <= 2]
# 遍历可能的下一步动作
for action in possible_actions:
if not valid_state(action):
continue
new_state = (m - action[0], c - action[1], action[2])
if new_state not in visited:
queue.append((new_state, actions + [action]))
visited.add(new_state)
return None
if __name__ == "__main__":
actions = solve()
if actions:
for action in actions:
if action[2] == 1:
print(f"商人和马过河,左岸: {3-action[0]}个商人, {3-action[1]}匹马;右岸: {action[0]}个商人, {action[1]}匹马")
else:
print(f"商人和马返回,左岸: {3-action[0]}个商人, {3-action[1]}匹马;右岸: {action[0]}个商人, {action[1]}匹马")
else:
print("无解")
```
这段代码使用了广度优先搜索算法来解决商人过河问题。它首先定义了一个 `valid_state` 函数来检查状态是否合法。然后,使用一个队列 `queue` 来存储当前状态和到达该状态的动作序列。通过不断从队列中弹出状态并生成可能的下一步动作,直到找到目标状态或队列为空为止。
注意:这个代码只是一个简单的实现,并没有进行优化处理,可能在处理大规模问题时效率较低。
阅读全文
相关推荐
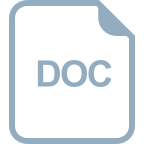
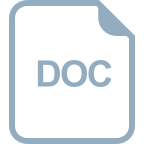


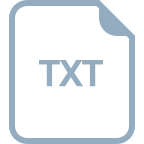










