java利用链式队列LinkQueue实现杨辉三角
时间: 2024-12-19 22:19:41 浏览: 10
在Java中,我们可以使用链表数据结构实现链式队列(LinkedQueue),然后结合递归或迭代的方式来构建杨辉三角。杨辉三角是一个经典的组合数学问题,其特点是每个数是它上面两个数的和。下面是一个简单的步骤:
1. 首先,创建一个Node类,用于表示链表中的节点,包含值和指向下一个节点的引用。
```java
class Node {
int value;
Node next;
public Node(int value) {
this.value = value;
this.next = null;
}
}
```
2. 然后,我们创建一个LinkedListQueue类,继承自`java.util.LinkedList`,并添加一些额外的方法来初始化链表。
```java
import java.util.LinkedList;
public class LinkedListQueue<NodeType> extends LinkedList<NodeType> {
// 添加初始化链表的方法
public void initTriangle(int n) {
for (int i = 0; i <= n; i++) {
add(new Node(i));
}
// 将链表变成杨辉三角的形式
buildYuanHuiTriangle();
}
private void buildYuanHuiTriangle() {
Node current = head;
while (current != null && current.next != null) {
Node temp = current.next.next;
current.next.next = current;
current = temp;
}
}
}
// 使用时
LinkedListQueue<Integer> queue = new LinkedListQueue<>();
queue.initTriangle(5);
```
3. `buildYuanHuiTriangle()`方法负责将链表转换成杨辉三角形的样子,即每一层的元素依次前移一个位置。最后的链表形状会形成一个三角形,对应杨辉三角的每一行。
阅读全文
相关推荐
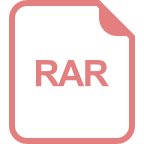
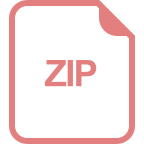
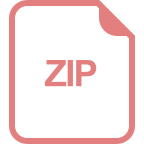


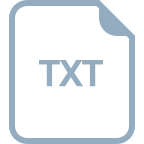
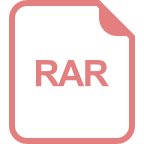
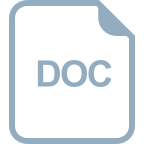
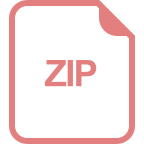
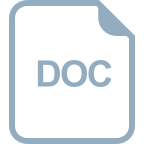
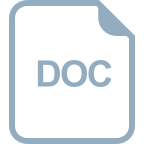
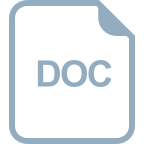
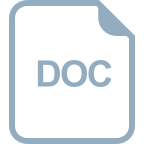






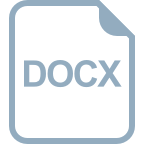