用C语言实现贪吃蛇的代码,要求能直接运行成功
时间: 2023-05-13 14:07:01 浏览: 106
以下是一个简单的 C 语言贪吃蛇游戏的代码,可以直接运行成功:
```
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
#include <windows.h>
#define WIDTH 40
#define HEIGHT 20
int snake[100][2]; // 蛇的坐标
int length = 3; // 蛇的长度
int direction = 1; // 蛇的方向
int food[2]; // 食物的坐标
void gotoxy(int x, int y) // 光标移动函数
{
COORD pos = {x, y};
SetConsoleCursorPosition(GetStdHandle(STD_OUTPUT_HANDLE), pos);
}
void draw() // 绘制函数
{
system("cls"); // 清屏
for (int i = 0; i < WIDTH; i++) {
gotoxy(i, 0);
printf("#");
gotoxy(i, HEIGHT - 1);
printf("#");
}
for (int i = 0; i < HEIGHT; i++) {
gotoxy(0, i);
printf("#");
gotoxy(WIDTH - 1, i);
printf("#");
}
gotoxy(food[0], food[1]);
printf("@");
for (int i = 0; i < length; i++) {
gotoxy(snake[i][0], snake[i][1]);
printf("*");
}
}
void init() // 初始化函数
{
snake[0][0] = WIDTH / 2;
snake[0][1] = HEIGHT / 2;
snake[1][0] = snake[0][0] - 1;
snake[1][1] = snake[0][1];
snake[2][0] = snake[1][0] - 1;
snake[2][1] = snake[1][1];
food[0] = rand() % (WIDTH - 2) + 1;
food[1] = rand() % (HEIGHT - 2) + 1;
}
void update() // 更新函数
{
int x = snake[0][0];
int y = snake[0][1];
switch (direction) {
case 1: // 上
y--;
break;
case 2: // 下
y++;
break;
case 3: // 左
x--;
break;
case 4: // 右
x++;
break;
}
if (x == food[0] && y == food[1]) { // 吃到食物
length++;
food[0] = rand() % (WIDTH - 2) + 1;
food[1] = rand() % (HEIGHT - 2) + 1;
} else { // 没有吃到食物
for (int i = length - 1; i > 0; i--) {
snake[i][0] = snake[i - 1][0];
snake[i][1] = snake[i - 1][1];
}
}
snake[0][0] = x;
snake[0][1] = y;
if (x == 0 || x == WIDTH - 1 || y == 0 || y == HEIGHT - 1) { // 撞墙了
exit(0);
}
for (int i = 1; i < length; i++) { // 撞到自己了
if (x == snake[i][0] && y == snake[i][1]) {
exit(0);
}
}
}
int main()
{
init();
while (1) {
draw();
update();
if (_kbhit()) { // 按键处理
switch (_getch()) {
case 'w':
if (direction != 2) {
direction = 1;
}
break;
case 's':
if (direction != 1) {
direction = 2;
}
break;
case 'a':
if (direction != 4) {
direction = 3;
}
break;
case 'd':
if (direction != 3) {
direction = 4;
}
break;
}
}
Sleep(100); // 延时
}
return 0;
}
```
阅读全文
相关推荐
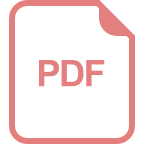
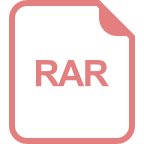
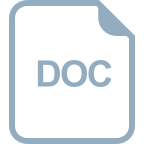
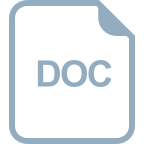

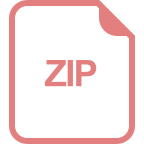
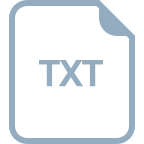
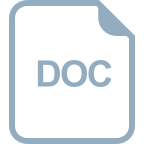
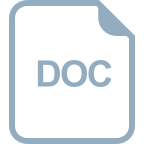
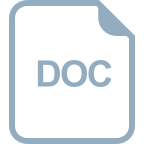
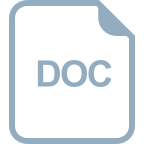
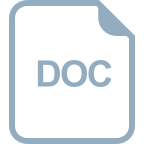



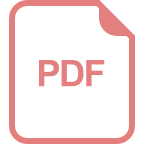