用cpp编写:定义一个点Point类,有点坐标属性(x, y),再定义一个矩形Rectangle类,其属性为两个Point点(分别表示矩形对角线上两点的坐标),及含有计算矩形的周长及面积的成员函数;再编写两个Point类的友元函数,分别计算两个点组成的矩形的周长与面积。在主函数中进行测试。
时间: 2024-03-27 20:40:04 浏览: 7
下面是用C++编写的实现上述功能的代码:
```cpp
#include <iostream>
#include <cmath>
using namespace std;
class Point {
private:
int x, y;
public:
Point(int x = 0, int y = 0): x(x), y(y) {}
friend class Rectangle;
friend double calcArea(const Point& p1, const Point& p2);
friend double calcPerimeter(const Point& p1, const Point& p2);
};
class Rectangle {
private:
Point p1, p2;
public:
Rectangle(Point p1, Point p2): p1(p1), p2(p2) {}
double calcArea() {
return abs((p1.x - p2.x) * (p1.y - p2.y));
}
double calcPerimeter() {
return 2 * (abs(p1.x - p2.x) + abs(p1.y - p2.y));
}
};
double calcArea(const Point& p1, const Point& p2) {
Rectangle r(p1, p2);
return r.calcArea();
}
double calcPerimeter(const Point& p1, const Point& p2) {
Rectangle r(p1, p2);
return r.calcPerimeter();
}
int main() {
Point p1(1, 1);
Point p2(3, 4);
Rectangle r(p1, p2);
cout << "Rectangle area: " << r.calcArea() << endl;
cout << "Rectangle perimeter: " << r.calcPerimeter() << endl;
cout << "Area of rectangle using two points: " << calcArea(p1, p2) << endl;
cout << "Perimeter of rectangle using two points: " << calcPerimeter(p1, p2) << endl;
return 0;
}
```
在这个程序中,Point类表示一个点,Rectangle类表示一个矩形,其中矩形由两个点来表示。程序中定义了两个Point类的友元函数,分别计算两个点组成的矩形的周长与面积。在主函数中进行测试,使用Rectangle类的成员函数计算矩形的周长和面积,同时也使用两个Point类的友元函数来计算矩形的周长和面积。
相关推荐
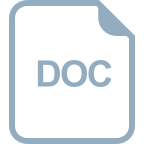














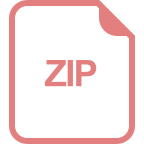
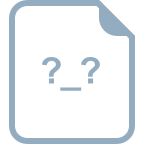