react实现轮播图
时间: 2023-07-11 14:10:00 浏览: 115
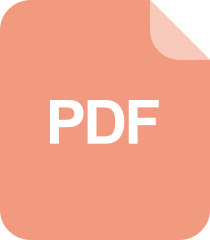
React实现轮播效果
好的,以下是使用 React 实现轮播图的示例代码:
首先,需要定义一个 Carousel 组件,该组件包含一个容器元素和多个轮播项元素。轮播项元素可以通过 props 传入,以便动态渲染轮播项。
```jsx
import React, { useState } from 'react';
function Carousel({ items }) {
const [currentIndex, setCurrentIndex] = useState(0);
function showItem(index) {
setCurrentIndex(index);
}
function prev() {
setCurrentIndex(currentIndex - 1);
if (currentIndex === 0) {
setCurrentIndex(items.length - 1);
}
}
function next() {
setCurrentIndex(currentIndex + 1);
if (currentIndex === items.length - 1) {
setCurrentIndex(0);
}
}
return (
<div className="carousel-container">
{items.map((item, index) => (
<div
key={index}
className={`carousel-item ${index === currentIndex ? 'active' : ''}`}
>
<img src={item.image} alt={item.caption} />
<div className="carousel-caption">
<h3>{item.caption}</h3>
<p>{item.description}</p>
</div>
</div>
))}
<div className="carousel-prev" onClick={prev}></div>
<div className="carousel-next" onClick={next}></div>
</div>
);
}
export default Carousel;
```
在上述代码中,使用了 useState 钩子来管理当前显示的轮播项索引。通过 showItem、prev 和 next 函数分别设置当前显示的轮播项索引,从而完成轮播图的切换。
接下来,为 Carousel 组件添加 CSS 样式,实现容器元素和轮播项的样式,以及左右切换按钮的样式。
```css
.carousel-container {
position: relative;
width: 800px;
height: 500px;
overflow: hidden;
}
.carousel-item {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
opacity: 0;
transition: all 1s ease-in-out;
}
.carousel-item.active {
opacity: 1;
}
.carousel-item img {
width: 100%;
height: 100%;
object-fit: cover;
}
.carousel-caption {
position: absolute;
bottom: 0;
left: 0;
width: 100%;
padding: 20px;
background-color: rgba(0, 0, 0, 0.5);
color: #fff;
font-size: 24px;
line-height: 1.5;
}
.carousel-prev,
.carousel-next {
position: absolute;
top: 50%;
transform: translateY(-50%);
width: 50px;
height: 50px;
background-color: rgba(0, 0, 0, 0.5);
cursor: pointer;
}
.carousel-prev {
left: 0;
}
.carousel-next {
right: 0;
}
```
最后,使用 Carousel 组件来渲染轮播图,传入轮播项数据。
```jsx
import React from 'react';
import Carousel from './Carousel';
function App() {
const items = [
{
image: 'image1.jpg',
caption: 'Image 1',
description: 'Description 1',
},
{
image: 'image2.jpg',
caption: 'Image 2',
description: 'Description 2',
},
{
image: 'image3.jpg',
caption: 'Image 3',
description: 'Description 3',
},
{
image: 'image4.jpg',
caption: 'Image 4',
description: 'Description 4',
},
{
image: 'image5.jpg',
caption: 'Image 5',
description: 'Description 5',
},
];
return <Carousel items={items} />;
}
export default App;
```
以上代码示例是一个简单的 React 轮播图实现,具体的实现方式可以根据实际需求进行调整和扩展。
阅读全文
相关推荐
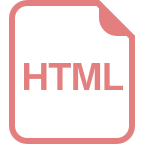
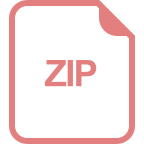


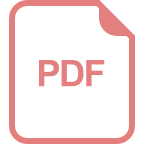
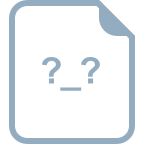
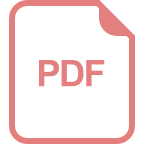
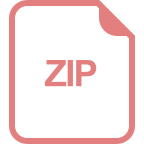
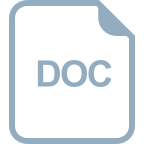





