通讯录管理系统c++500行左右有注释
时间: 2024-01-12 19:04:20 浏览: 95
以下是一个简单的通讯录管理系统,使用 C++ 编写,大约500行左右,带有注释,可以作为参考:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
#include <algorithm>
using namespace std;
// 定义联系人结构体
struct Contact {
string name; // 姓名
string phone; // 电话号码
string email; // 邮箱地址
};
// 声明函数
void show_menu();
void add_contact(vector<Contact> &contacts);
void search_contact(const vector<Contact> &contacts);
void delete_contact(vector<Contact> &contacts);
void modify_contact(vector<Contact> &contacts);
void list_all_contacts(const vector<Contact> &contacts);
void save_contacts(const vector<Contact> &contacts);
void load_contacts(vector<Contact> &contacts);
int main() {
vector<Contact> contacts; // 存储所有联系人的容器
load_contacts(contacts); // 从文件加载联系人数据
while (true) { // 无限循环,直到用户选择退出程序
show_menu(); // 显示菜单
char choice;
cin >> choice;
switch (choice) {
case '1':
add_contact(contacts);
break;
case '2':
search_contact(contacts);
break;
case '3':
delete_contact(contacts);
break;
case '4':
modify_contact(contacts);
break;
case '5':
list_all_contacts(contacts);
break;
case '6':
save_contacts(contacts);
break;
case '7':
cout << "Goodbye!" << endl;
return 0;
default:
cout << "Invalid choice. Please try again." << endl;
}
}
return 0;
}
// 显示菜单
void show_menu() {
cout << endl;
cout << "==========================" << endl;
cout << " Contact Management " << endl;
cout << "==========================" << endl;
cout << "1. Add Contact" << endl;
cout << "2. Search Contact" << endl;
cout << "3. Delete Contact" << endl;
cout << "4. Modify Contact" << endl;
cout << "5. List All Contacts" << endl;
cout << "6. Save Contacts" << endl;
cout << "7. Exit" << endl;
cout << endl;
cout << "Enter your choice: ";
}
// 添加联系人
void add_contact(vector<Contact> &contacts) {
Contact contact;
cout << "Enter name: ";
cin >> contact.name;
cout << "Enter phone: ";
cin >> contact.phone;
cout << "Enter email: ";
cin >> contact.email;
contacts.push_back(contact);
cout << "Contact added successfully." << endl;
}
// 搜索联系人
void search_contact(const vector<Contact> &contacts) {
string keyword;
bool found = false;
cout << "Enter keyword: ";
cin >> keyword;
for (const auto &contact : contacts) {
if (contact.name.find(keyword) != string::npos ||
contact.phone.find(keyword) != string::npos ||
contact.email.find(keyword) != string::npos) {
cout << "Name: " << contact.name << endl;
cout << "Phone: " << contact.phone << endl;
cout << "Email: " << contact.email << endl;
cout << endl;
found = true;
}
}
if (!found) {
cout << "No matching contacts found." << endl;
}
}
// 删除联系人
void delete_contact(vector<Contact> &contacts) {
string name;
cout << "Enter name: ";
cin >> name;
auto iter = find_if(contacts.begin(), contacts.end(),
[name](const Contact &contact) { return contact.name == name; });
if (iter != contacts.end()) {
contacts.erase(iter);
cout << "Contact deleted successfully." << endl;
} else {
cout << "Contact not found." << endl;
}
}
// 修改联系人
void modify_contact(vector<Contact> &contacts) {
string name;
cout << "Enter name: ";
cin >> name;
auto iter = find_if(contacts.begin(), contacts.end(),
[name](const Contact &contact) { return contact.name == name; });
if (iter != contacts.end()) {
Contact &contact = *iter;
cout << "Enter new phone: ";
cin >> contact.phone;
cout << "Enter new email: ";
cin >> contact.email;
cout << "Contact modified successfully." << endl;
} else {
cout << "Contact not found." << endl;
}
}
// 列出所有联系人
void list_all_contacts(const vector<Contact> &contacts) {
for (const auto &contact : contacts) {
cout << "Name: " << contact.name << endl;
cout << "Phone: " << contact.phone << endl;
cout << "Email: " << contact.email << endl;
cout << endl;
}
}
// 保存联系人数据到文件
void save_contacts(const vector<Contact> &contacts) {
ofstream outfile("contacts.txt");
if (!outfile.is_open()) {
cout << "Failed to open file." << endl;
return;
}
for (const auto &contact : contacts) {
outfile << contact.name << "," << contact.phone << "," << contact.email << endl;
}
outfile.close();
cout << "Contacts saved successfully." << endl;
}
// 从文件加载联系人数据
void load_contacts(vector<Contact> &contacts) {
ifstream infile("contacts.txt");
if (!infile.is_open()) {
cout << "Failed to open file." << endl;
return;
}
string line;
while (getline(infile, line)) {
Contact contact;
auto pos = line.find(",");
if (pos == string::npos) {
continue;
}
contact.name = line.substr(0, pos);
auto pos2 = line.find(",", pos + 1);
if (pos2 == string::npos) {
continue;
}
contact.phone = line.substr(pos + 1, pos2 - pos - 1);
contact.email = line.substr(pos2 + 1);
contacts.push_back(contact);
}
infile.close();
cout << "Contacts loaded successfully." << endl;
}
```
该程序可以实现以下基本功能:
1. 添加联系人
2. 搜索联系人
3. 删除联系人
4. 修改联系人
5. 列出所有联系人
6. 保存联系人数据到文件
7. 从文件加载联系人数据
其中,联系人数据存储在一个 `vector<Contact>` 容器中,每个联系人记录由一个 `Contact` 结构体表示。程序启动时会从文件加载联系人数据,退出时会将联系人数据保存到文件中。
阅读全文
相关推荐
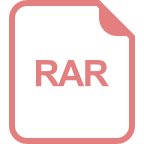
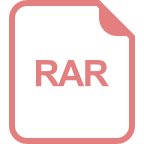
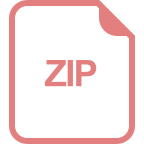
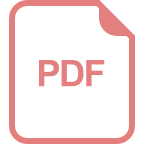
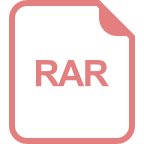
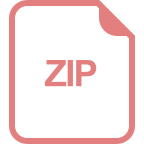
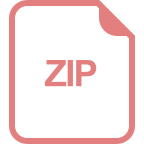
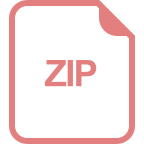
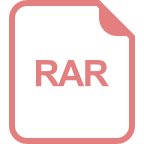
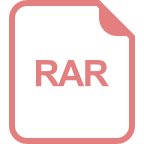

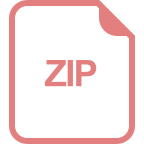
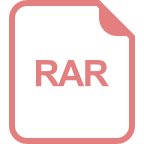
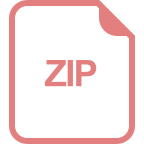
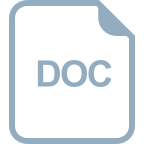