如何使用C sharp语言中的string splite
时间: 2023-04-10 11:03:36 浏览: 120
可以使用以下代码来使用C#语言中的string split函数:
string str = "Hello,World";
string[] strArray = str.Split(',');
这将把字符串"Hello,World"分割成两个字符串"Hello"和"World",并将它们存储在一个字符串数组中。
相关问题
用c sharp语言实现四叉树代码
### 回答1:
以下是C#语言实现四叉树的代码:
```csharp
using System.Collections.Generic;
using UnityEngine;
public class QuadTree
{
private int capacity = 4;
private int depth = 0;
private int maxDepth = 5;
private List<GameObject> objects;
private Bounds bounds;
private QuadTree[] nodes;
public QuadTree(int depth, Bounds bounds)
{
this.depth = depth;
this.bounds = bounds;
objects = new List<GameObject>();
nodes = new QuadTree[4];
}
public void Insert(GameObject obj)
{
if (!bounds.Contains(obj.transform.position))
return;
if (objects.Count < capacity || depth == maxDepth)
{
objects.Add(obj);
}
else
{
if (nodes[0] == null)
Split();
nodes[0].Insert(obj);
nodes[1].Insert(obj);
nodes[2].Insert(obj);
nodes[3].Insert(obj);
}
}
public List<GameObject> Retrieve(GameObject obj)
{
List<GameObject> objs = new List<GameObject>();
if (bounds.Contains(obj.transform.position))
{
foreach (GameObject o in objects)
objs.Add(o);
if (nodes[0] != null)
{
objs.AddRange(nodes[0].Retrieve(obj));
objs.AddRange(nodes[1].Retrieve(obj));
objs.AddRange(nodes[2].Retrieve(obj));
objs.AddRange(nodes[3].Retrieve(obj));
}
}
return objs;
}
private void Split()
{
float subWidth = bounds.size.x / 2f;
float subHeight = bounds.size.y / 2f;
float x = bounds.center.x;
float y = bounds.center.y;
nodes[0] = new QuadTree(depth + 1, new Bounds(new Vector3(x + subWidth / 2f, y + subHeight / 2f, 0f), new Vector3(subWidth, subHeight, 1f)));
nodes[1] = new QuadTree(depth + 1, new Bounds(new Vector3(x - subWidth / 2f, y + subHeight / 2f, 0f), new Vector3(subWidth, subHeight, 1f)));
nodes[2] = new QuadTree(depth + 1, new Bounds(new Vector3(x - subWidth / 2f, y - subHeight / 2f, 0f), new Vector3(subWidth, subHeight, 1f)));
nodes[3] = new QuadTree(depth + 1, new Bounds(new Vector3(x + subWidth / 2f, y - subHeight / 2f, 0f), new Vector3(subWidth, subHeight, 1f)));
}
}
```
这是一个简单的四叉树实现,用于对游戏中的物体进行空间划分。四叉树是一种递归地将空间划分成四个象限的数据结构,每个节点可以容纳一定数量的物体。当节点的物体数量超过容量时,节点会被细分成四个子节点。这样可以提高物体的查找效率,避免遍历整个场景中的所有物体。
### 回答2:
四叉树是一种用于将二维空间划分为多个小区域的数据结构,常用于解决涉及空间索引和查询的问题。以下是用C#语言实现四叉树的代码示例:
```csharp
using System.Collections.Generic;
public class QuadTree
{
private Node root;
public QuadTree(double x, double y, double width, double height)
{
root = new Node(x, y, width, height);
}
public void Insert(Point point)
{
root.Insert(point);
}
public List<Point> QueryRange(double x, double y, double width, double height)
{
List<Point> pointsInRange = new List<Point>();
root.QueryRange(x, y, width, height, ref pointsInRange);
return pointsInRange;
}
private class Node
{
private double x;
private double y;
private double width;
private double height;
private List<Point> points;
private Node topLeft;
private Node topRight;
private Node bottomLeft;
private Node bottomRight;
public Node(double x, double y, double width, double height)
{
this.x = x;
this.y = y;
this.width = width;
this.height = height;
points = new List<Point>();
topLeft = null;
topRight = null;
bottomLeft = null;
bottomRight = null;
}
public void Insert(Point point)
{
if (!Contains(point))
return;
if (points.Count < 4)
{
points.Add(point);
}
else
{
if (topLeft == null)
Split();
topLeft.Insert(point);
topRight.Insert(point);
bottomLeft.Insert(point);
bottomRight.Insert(point);
}
}
public void QueryRange(double x, double y, double width, double height, ref List<Point> pointsInRange)
{
if (!Intersects(x, y, width, height))
return;
foreach (Point point in points)
{
if (point.X >= x && point.X <= x + width && point.Y >= y && point.Y <= y + height)
pointsInRange.Add(point);
}
if (topLeft != null)
{
topLeft.QueryRange(x, y, width, height, ref pointsInRange);
topRight.QueryRange(x, y, width, height, ref pointsInRange);
bottomLeft.QueryRange(x, y, width, height, ref pointsInRange);
bottomRight.QueryRange(x, y, width, height, ref pointsInRange);
}
}
private bool Contains(Point point)
{
return point.X >= x && point.X <= x + width && point.Y >= y && point.Y <= y + height;
}
private bool Intersects(double rangeX, double rangeY, double rangeWidth, double rangeHeight)
{
return x < rangeX + rangeWidth && x + width > rangeX && y < rangeY + rangeHeight && y + height > rangeY;
}
private void Split()
{
double halfWidth = width / 2;
double halfHeight = height / 2;
topLeft = new Node(x, y, halfWidth, halfHeight);
topRight = new Node(x + halfWidth, y, halfWidth, halfHeight);
bottomLeft = new Node(x, y + halfHeight, halfWidth, halfHeight);
bottomRight = new Node(x + halfWidth, y + halfHeight, halfWidth, halfHeight);
foreach (Point point in points)
{
topLeft.Insert(point);
topRight.Insert(point);
bottomLeft.Insert(point);
bottomRight.Insert(point);
}
points.Clear();
}
}
}
public class Point
{
public double X { get; }
public double Y { get; }
public Point(double x, double y)
{
X = x;
Y = y;
}
}
```
以上是用C#语言编写的简单四叉树实现代码。在QuadTree类中,构造函数初始化根节点,Insert方法用于将点插入四叉树中,QueryRange方法用于查询给定范围内的所有点。内部的Node类表示四叉树的节点,包括节点的坐标范围、存储的点、以及四个子节点。四叉树的划分采用递归的方式,当节点内的点数量达到阈值时会自动进行划分。希望这能帮助到你!
### 回答3:
下面是用C#语言实现四叉树的代码示例:
```csharp
using System;
using System.Collections.Generic;
public class QuadTreeNode
{
public int x;
public int y;
public QuadTreeNode[] children;
public bool isLeaf;
public QuadTreeNode(int x, int y)
{
this.x = x;
this.y = y;
children = new QuadTreeNode[4];
isLeaf = true;
}
}
public class QuadTree
{
public QuadTreeNode root;
public QuadTree(int x, int y)
{
root = new QuadTreeNode(x, y);
}
public void Insert(int x, int y)
{
Insert(root, x, y);
}
private void Insert(QuadTreeNode node, int x, int y)
{
if (node.isLeaf)
{
// 如果节点是叶子节点,将节点扩展为四个子节点
Split(node);
}
// 将数据插入到子节点中
int quadrant = GetQuadrant(node, x, y);
Insert(node.children[quadrant], x, y);
}
private void Split(QuadTreeNode node)
{
int childX = node.x;
int childY = node.y;
int childWidth = node.isLeaf ? 1 : node.children[0].x - node.x;
for (int i = 0; i < 4; i++)
{
node.children[i] = new QuadTreeNode(childX, childY);
childX += childWidth;
}
node.isLeaf = false;
}
private int GetQuadrant(QuadTreeNode node, int x, int y)
{
if (x < node.x + (node.children[0].x - node.x) / 2)
{
if (y < node.y + (node.children[0].y - node.y) / 2)
{
return 0; // 左上
}
else
{
return 2; // 左下
}
}
else
{
if (y < node.y + (node.children[0].y - node.y) / 2)
{
return 1; // 右上
}
else
{
return 3; // 右下
}
}
}
public void Print()
{
Print(root, "");
}
private void Print(QuadTreeNode node, string indent)
{
Console.WriteLine(indent + "(" + node.x + ", " + node.y + ")");
if (!node.isLeaf)
{
for (int i = 0; i < 4; i++)
{
Print(node.children[i], indent + " ");
}
}
}
}
public class Program
{
public static void Main(string[] args)
{
QuadTree quadTree = new QuadTree(0, 0);
quadTree.Insert(1, 1);
quadTree.Insert(2, 3);
quadTree.Insert(4, 5);
quadTree.Insert(6, 7);
quadTree.Print();
}
}
```
上述代码实现了一个简单的四叉树数据结构。QuadTreeNode表示四叉树的节点,包含节点的坐标和四个子节点;QuadTree表示四叉树,包含根节点和插入数据的方法。在Insert方法中,会根据数据的坐标计算出应该插入的子节点,并递归地进行插入操作。Split方法用于将叶子节点扩展为四个子节点。GetQuadrant方法用于根据坐标获取节点所在的象限。Print方法用于打印四叉树的结构。
在Main方法中,我们创建了一个QuadTree对象,然后插入了一些数据,并打印整个四叉树的结构。您可以修改Main方法中的插入操作和添加更多数据,以测试四叉树的功能。
C#:在AnyCPU模式下使用CefSharp
在使用CefSharp时,建议在项目的属性窗口中选择AnyCPU模式,因为CefSharp是基于Chromium的,所以它可以在x86和x64平台上运行,同时也可以在Windows和Linux上运行。如果你选择了AnyCPU模式,那么你需要在你的应用程序中进行一些配置,以确保它能够在x86和x64平台上运行。
首先,你需要在你的应用程序中添加以下代码:
```csharp
if (Environment.Is64BitProcess)
{
CefSettings settings = new CefSettings();
settings.BrowserSubprocessPath = @"x64\CefSharp.BrowserSubprocess.exe";
Cef.Initialize(settings);
}
else
{
Cef.Initialize();
}
```
这段代码将根据你的应用程序是64位还是32位来选择正确的CefSharp.BrowserSubprocess.exe路径。然后,你需要将CefSharp.BrowserSubprocess.exe和libcef.dll文件复制到你的应用程序的输出目录中。这些文件可以在CefSharp NuGet软件包中找到。
最后,你需要在你的应用程序中添加以下代码:
```csharp
AppDomain.CurrentDomain.AssemblyResolve += (sender, args) =>
{
if (args.Name.StartsWith("CefSharp"))
{
string assemblyName = args.Name.Split(new char[] { ',' }, 2)[0] + ".dll";
string archSpecificPath = Path.Combine(AppDomain.CurrentDomain.SetupInformation.ApplicationBase, Environment.Is64BitProcess ? "x64" : "x86", assemblyName);
return File.Exists(archSpecificPath) ? Assembly.LoadFile(archSpecificPath) : null;
}
return null;
};
```
这段代码将允许你的应用程序在加载CefSharp程序集时选择正确的版本,以便在x86和x64平台上运行。
相关推荐
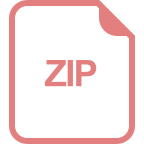
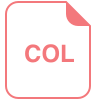
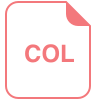
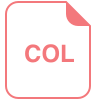
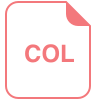
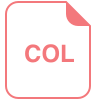







