已知f为单链表的表头指针,链表中存储的都是整型数据,试写出实现下列运算的递归算法和非递归算法:(1)求链表中的最大整数。(2)求所有整数的平均值
时间: 2024-10-13 07:05:39 浏览: 79
(1)递归算法求链表中的最大整数:
```python
def find_max_recursively(head):
# 基本情况:空链表返回None
if not head:
return None
# 递归情况:比较当前节点值与当前最大值,取较大者
max_val = head.val
if max_val > find_max_recursively(head.next):
max_val = head.val
return max_val
# 非递归算法求链表中的最大整数(迭代)
def find_max_iteratively(head):
max_val = head.val
current = head.next
while current:
if current.val > max_val:
max_val = current.val
current = current.next
return max_val
```
(2)递归算法求链表中所有整数的平均值:
```python
def average_of_list_recursively(head):
def sum_and_count(node):
if not node:
return 0, 0
total, count = sum_and_count(node.next)
total += node.val
count += 1
return total, count
total, count = sum_and_count(head)
if count == 0:
return None # 防止除以零的情况
else:
return total / count
# 非递归算法求链表中所有整数的平均值(迭代)
def average_of_list_iteratively(head):
total = 0
count = 0
current = head
while current:
total += current.val
count += 1
current = current.next
return total / count if count != 0 else None
```
阅读全文
相关推荐
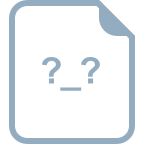
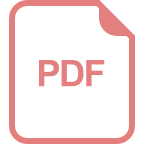
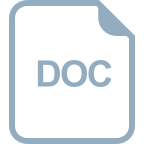













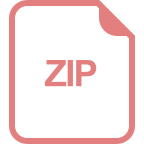