vue axios 全局节流防抖
时间: 2023-09-29 08:08:31 浏览: 62
在 Vue 中使用 Axios 全局节流防抖的方法有很多,以下是其中一种常见的方法:
1. 在 main.js 中,引入 lodash 的 throttle 和 debounce 方法
```javascript
import _ from 'lodash'
Vue.prototype.$throttle = _.throttle
Vue.prototype.$debounce = _.debounce
```
2. 在 Vue 实例中,使用 $throttle 或 $debounce 方法包装 Axios 请求方法
```javascript
import axios from 'axios'
export default {
methods: {
// 使用 $throttle 包装 Axios 请求方法,实现全局节流
async $throttleAxios(url, data, config) {
return this.$throttle(async () => {
try {
const response = await axios.post(url, data, config)
return response.data
} catch (error) {
console.error(error)
}
}, 1000)
},
// 使用 $debounce 包装 Axios 请求方法,实现全局防抖
async $debounceAxios(url, data, config) {
return this.$debounce(async () => {
try {
const response = await axios.post(url, data, config)
return response.data
} catch (error) {
console.error(error)
}
}, 1000)
},
},
}
```
在上述代码中,$throttleAxios 和 $debounceAxios 方法分别使用 $throttle 和 $debounce 方法包装了 Axios 请求方法,实现了全局的节流和防抖。其中,$throttleAxios 的节流时间间隔为 1000ms,$debounceAxios 的防抖时间间隔也为 1000ms。
3. 在组件中调用全局节流防抖的 Axios 请求方法
```javascript
export default {
methods: {
async fetchData() {
// 调用 $throttleAxios 方法获取数据
const data = await this.$throttleAxios('/api/data', { page: 1 })
// 调用 $debounceAxios 方法保存数据
await this.$debounceAxios('/api/save', data)
},
},
}
```
在组件中,通过调用 $throttleAxios 和 $debounceAxios 方法,即可实现全局节流防抖的 Axios 请求。
相关推荐
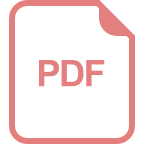
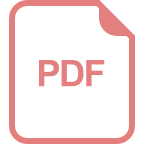














