用Python画出双摆系统中第二个摆球的运动轨迹
时间: 2024-10-08 20:05:39 浏览: 30
在Python中绘制双摆系统中第二个摆球的运动轨迹通常需要结合物理学原理和数值模拟。这里我们可以使用matplotlib库来可视化动态过程,NumPy处理数学计算,以及可能需要用到的科学计算库如scipy。下面是一个简化的步骤:
1. **导入必要的库**:
```python
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.animation import FuncAnimation
```
2. **设置物理参数**:
```python
g = 9.81 # 重力加速度
l1 = l2 = 1 # 摆长(假设两个摆长相等)
theta0 = [np.pi / 4, 0] # 初始角度
omega0 = [0, 0] # 初始角速度
t_max = 10 # 总时间
dt = 0.01 # 时间步长
```
3. **定义摆动方程(通过欧拉法或其他数值方法)**:
```python
def derivatives(theta, omega, t):
theta1, theta2 = theta
omega1, omega2 = omega
dtheta1_dt = omega1
dtheta2_dt = (omega2 * l2 * np.sin(theta2) - g / l2 * np.cos(theta2)) / (1 + l1 * l2 * np.sin(theta1) * np.sin(theta2))
return [dtheta1_dt, dtheta2_dt]
# 使用odeint函数解微分方程
times = np.arange(0, t_max, dt)
solution = odeint(derivatives, theta0 + omega0, times)
```
4. **创建图形和动画**:
```python
fig, ax = plt.subplots()
line1, line2 = ax.plot([], [], 'o-', [], [], 'ro-')
def animate(i):
x1 = l1 * np.sin(solution[i][0])
y1 = -l1 * np.cos(solution[i][0])
x2 = x1 + l2 * np.sin(solution[i][1])
y2 = y1 - l2 * np.cos(solution[i][1])
line1.set_data([0, x1], [0, y1])
line2.set_data([x1, x2], [y1, y2])
ani = FuncAnimation(fig, animate, frames=len(times), interval=50)
plt.xlim([-2, 2])
plt.ylim([-2, 2])
plt.xlabel('x')
plt.ylabel('y')
plt.title('双摆系统中第二个摆球的运动轨迹')
plt.show()
阅读全文
相关推荐
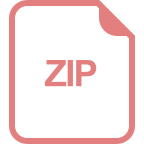
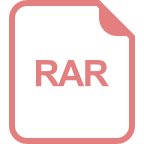
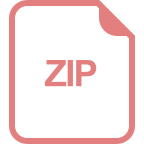




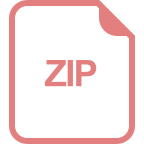
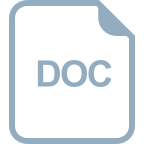
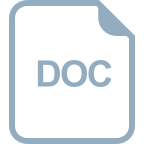
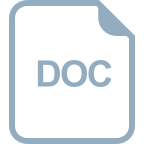
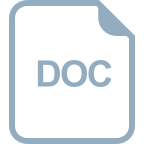
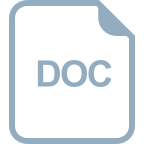
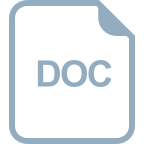
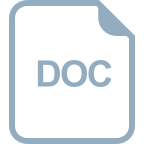



