如何用正则表达式判断一个字符串是否是时间格式
时间: 2024-02-05 10:10:56 浏览: 171
可以使用以下正则表达式进行匹配:
```
^([01]\d|2[0-3]):[0-5]\d:[0-5]\d$
```
该正则表达式可以匹配 HH:MM:SS 格式的时间字符串,其中 HH 可以是 00~23 之间的任意数字,MM 和 SS 可以是 00~59 之间的任意数字。使用 ^ 和 $ 符号限定整个字符串必须符合该格式,避免误匹配其他字符串。
相关问题
java正则表达式判断字符串是不是时间格式
可以使用Java正则表达式来判断字符串是否符合时间格式。以下是一个示例代码:
```
public static boolean checkIsTimeFormat(String str){
if(str == null || "".equals(str)){
return false;
}
String regex = "^([01]\\d|2[0-3]):([0-5]\\d)$";
Pattern p = Pattern.compile(regex);
Matcher matcher = p.matcher(str);
return matcher.matches();
}
```
该方法使用正则表达式来匹配符合时间格式的字符串,其中正则表达式`^([01]\\d|2[0-3]):([0-5]\\d)$`表示以0或1开头的两位数字,或者以2开头的0到3之间的数字,后面跟着一个冒号,再后面是0到5之间的数字,共计5个字符。如果字符串符合该正则表达式,则返回true,否则返回false。
令字符串 s='马丽于2022-4-29 10:10刊发了一首诗《Listen to me》,诗词的大意是:\n Mama you taught me to do the right things,\n So now you have to let your baby fly.\n You've given me everything that I will need,\n To make it through this crazy thing called life,\n Thank you Mum!' ,通过编写函数实现下述各题功能。 1 应用正则表达式判断该字符串是否包含0~9的数字和大小写英文字母。 2 根据正则表达式使用中文逗号、中文句号、英文逗号、英文点号、英文感叹号、英文换行符号将该字符串分割成多个子串。 3 应用正则表达式提取该字符串中的日期时间字符串。 4 应用正则表达式提取该字符串中的诗词名称。 5 应用正则表达式提取该字符串中的姓名。
1. 使用re模块判断字符串是否包含0~9的数字和大小写英文字母:
```python
import re
s = '马丽于2022-4-29 10:10刊发了一首诗《Listen to me》,诗词的大意是:\n Mama you taught me to do the right things,\n So now you have to let your baby fly.\n You\'ve given me everything that I will need,\n To make it through this crazy thing called life,\n Thank you Mum!'
pattern = re.compile('[0-9a-zA-Z]+')
result = pattern.findall(s)
if result:
print('字符串中包含数字和英文字母')
else:
print('字符串中不包含数字和英文字母')
```
2. 使用正则表达式将该字符串分割成多个子串:
```python
import re
s = '马丽于2022-4-29 10:10刊发了一首诗《Listen to me》,诗词的大意是:\n Mama you taught me to do the right things,\n So now you have to let your baby fly.\n You\'ve given me everything that I will need,\n To make it through this crazy thing called life,\n Thank you Mum!'
pattern = re.compile('[,,.。!!\n]')
result = pattern.split(s)
print(result)
```
3. 使用正则表达式提取该字符串中的日期时间字符串:
```python
import re
s = '马丽于2022-4-29 10:10刊发了一首诗《Listen to me》,诗词的大意是:\n Mama you taught me to do the right things,\n So now you have to let your baby fly.\n You\'ve given me everything that I will need,\n To make it through this crazy thing called life,\n Thank you Mum!'
pattern = re.compile('\d{4}-\d{1,2}-\d{1,2} \d{1,2}:\d{1,2}')
result = pattern.findall(s)
print(result)
```
4. 使用正则表达式提取该字符串中的诗词名称:
```python
import re
s = '马丽于2022-4-29 10:10刊发了一首诗《Listen to me》,诗词的大意是:\n Mama you taught me to do the right things,\n So now you have to let your baby fly.\n You\'ve given me everything that I will need,\n To make it through this crazy thing called life,\n Thank you Mum!'
pattern = re.compile('《(.+?)》')
result = pattern.findall(s)
print(result)
```
5. 使用正则表达式提取该字符串中的姓名:
```python
import re
s = '马丽于2022-4-29 10:10刊发了一首诗《Listen to me》,诗词的大意是:\n Mama you taught me to do the right things,\n So now you have to let your baby fly.\n You\'ve given me everything that I will need,\n To make it through this crazy thing called life,\n Thank you Mum!'
pattern = re.compile('[\u4e00-\u9fa5]{2,3}')
result = pattern.findall(s)
print(result)
```
阅读全文
相关推荐
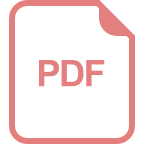
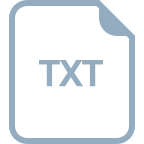
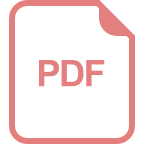
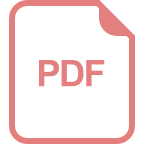
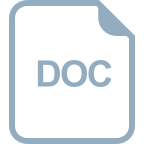
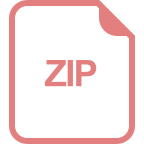
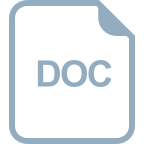
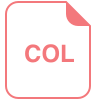
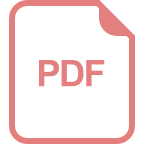
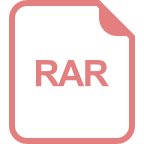
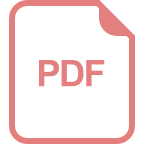
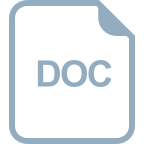
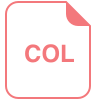
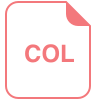
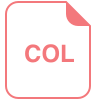