c语言基于邻接表创建一个图,图的创建用键盘输入
时间: 2024-02-13 13:06:31 浏览: 64
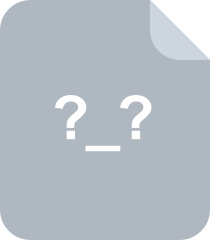
以邻接表创建图实现图的基本操作

下面是基于邻接表创建图的代码,可以通过键盘输入实现:
```
#include <stdio.h>
#include <stdlib.h>
// 定义邻接表结构体
typedef struct node {
int vertex; // 存储顶点编号
struct node* next; // 指向下一个邻接点的指针
} Node;
// 创建邻接表结点
Node* createNode(int v) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->vertex = v;
newNode->next = NULL;
return newNode;
}
// 添加边
void addEdge(Node** graph, int u, int v) {
// 将v添加到u的邻接表中
Node* newNode = createNode(v);
newNode->next = graph[u];
graph[u] = newNode;
// 将u添加到v的邻接表中
newNode = createNode(u);
newNode->next = graph[v];
graph[v] = newNode;
}
// 创建图
Node** createGraph(int n) {
Node** graph = (Node**)malloc(n * sizeof(Node*));
int i;
for (i = 0; i < n; i++) {
graph[i] = NULL;
}
int u, v;
while (1) {
printf("请输入边的起始顶点和结束顶点(输入-1结束):");
scanf("%d %d", &u, &v);
if (u == -1 || v == -1) {
break;
}
addEdge(graph, u, v);
}
return graph;
}
// 打印图
void printGraph(Node** graph, int n) {
int i;
for (i = 0; i < n; i++) {
printf("顶点%d的邻接表:", i);
Node* p = graph[i];
while (p != NULL) {
printf("%d ", p->vertex);
p = p->next;
}
printf("\n");
}
}
int main() {
int n;
printf("请输入顶点数量:");
scanf("%d", &n);
Node** graph = createGraph(n);
printGraph(graph, n);
return 0;
}
```
在主函数中,首先输入顶点数量,然后调用`createGraph`函数创建图,该函数通过循环输入边的起始顶点和结束顶点,并调用`addEdge`函数添加边。最后,调用`printGraph`函数打印图的邻接表。
阅读全文
相关推荐


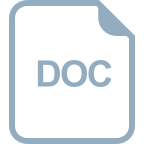












