用unity 写30行代码的射击游戏
时间: 2024-06-10 10:07:00 浏览: 250
好的,我可以回答这个问题。您需要先在Unity中创建一个新项目,然后创建一个新的场景。在场景中创建一个主摄像机并调整其位置和角度。然后创建一个空对象并将其命名为“Player”。接下来,您需要添加一个3D模型,并设置其为玩家对象的子对象。您还需要添加一个射击器脚本,该脚本将负责处理射击行为。这个脚本将包含一些简单的代码,如检测玩家输入、实例化子弹对象、控制子弹的移动、检测子弹与敌人的碰撞等。最后,您需要添加敌人对象,并为其创建一个简单的移动脚本。这样,您就可以创建一个简单的射击游戏了,只需约30行代码即可完成。
相关问题
unity 互联网射击游戏代码
互联网射击游戏是一个比较复杂的项目,需要涉及到多个方面的知识和技能。以下是一个简单的 Unity 互联网射击游戏的代码示例,供你参考:
```
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.Networking;
public class PlayerController : NetworkBehaviour {
public GameObject bulletPrefab;
public Transform bulletSpawn;
void Update () {
if (!isLocalPlayer) {
return;
}
float x = Input.GetAxis("Horizontal") * Time.deltaTime * 150.0f;
float z = Input.GetAxis("Vertical") * Time.deltaTime * 3.0f;
transform.Rotate(0, x, 0);
transform.Translate(0, 0, z);
if (Input.GetKeyDown(KeyCode.Space)) {
CmdFire();
}
}
[Command]
void CmdFire() {
GameObject bullet = (GameObject)Instantiate(
bulletPrefab,
bulletSpawn.position,
bulletSpawn.rotation);
bullet.GetComponent<Rigidbody>().velocity = bullet.transform.forward * 6;
NetworkServer.Spawn(bullet);
Destroy(bullet, 2.0f);
}
public override void OnStartLocalPlayer() {
GetComponent<MeshRenderer>().material.color = Color.blue;
}
}
```
此代码示例中,玩家控制器(PlayerController)可以接收输入并将其应用于玩家对象的旋转和移动。如果玩家按下空格键,则会调用 CmdFire() 方法,该方法将在服务器上创建一个新的子弹对象,并将其发送到所有客户端以进行同步。最后,玩家控制器使用 Unity 网络 API 中的 OnStartLocalPlayer() 方法将其自身的颜色设置为蓝色。
请注意,这只是一个简单的示例代码,实际的互联网射击游戏需要更多的功能和代码来实现。
unity射击游戏代码
Unity是一款强大的游戏引擎,用于开发各种类型的游戏,包括射击游戏。在Unity中创建射击游戏的基本步骤和关键代码片段会涉及C#编程语言。以下是一个简化的概述:
1. **项目初始化**:
创建一个新的Unity项目,选择2D或3D项目,并导入必要的资源如角色、武器模型、音效等。
```csharp
using UnityEngine;
public class PlayerController : MonoBehaviour
{
public float moveSpeed = 5f;
//...
}
```
2. **角色控制**:
编写角色移动和射击的脚本,使用Input系统获取玩家输入。
```csharp
void Update()
{
float moveHorizontal = Input.GetAxis("Horizontal");
float moveVertical = Input.GetAxis("Vertical");
transform.position += new Vector3(moveHorizontal, 0, moveSpeed * Time.deltaTime);
if (Input.GetButtonDown("Fire1"))
{
Shoot();
}
}
private void Shoot()
{
//...
}
```
3. **射击行为**:
创建子弹发射器和子弹组件,当触发射击事件时,创建并发射子弹。
```csharp
public class Shooter : MonoBehaviour
{
private Transform weaponPosition;
private GameObject bulletPrefab;
private void Start()
{
weaponPosition = GetComponent<Transform>();
}
public void Shoot()
{
GameObject bullet = Instantiate(bulletPrefab, weaponPosition.position, weaponPosition.rotation);
bullet.GetComponent<Rigidbody2D>().velocity = new Vector2(0, 10f); // 假设是2D游戏
}
}
```
4. **碰撞检测**:
如果需要,可以通过Rigidbody组件实现子弹与敌人的碰撞检测。
5. **音频反馈**:
在适当的地方添加音效播放代码,如射击声、击中声等。
阅读全文
相关推荐
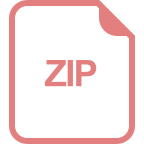
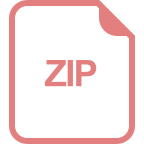
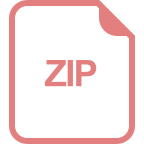
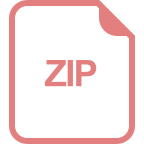
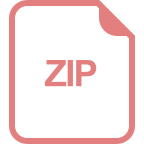
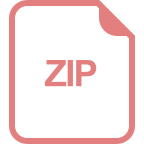




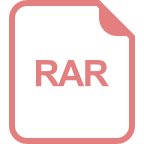
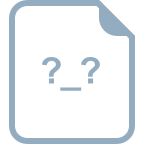
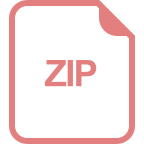