帮我写一段贪吃蛇代码
时间: 2023-07-10 22:24:55 浏览: 79
好的,以下是一个简单的贪吃蛇游戏的 Python 代码,你可以在命令行中运行它:
```python
import curses
import time
import random
# 初始化 curses 库
screen = curses.initscr()
curses.curs_set(0)
screen_height, screen_width = screen.getmaxyx()
window = curses.newwin(screen_height, screen_width, 0, 0)
window.keypad(1)
window.timeout(100)
# 初始化蛇和食物
snake_x = screen_width // 4
snake_y = screen_height // 2
snake = [[snake_y, snake_x], [snake_y, snake_x - 1], [snake_y, snake_x - 2]]
food = [screen_height // 2, screen_width // 2]
window.addch(food[0], food[1], curses.ACS_PI)
# 初始化游戏状态
key = curses.KEY_RIGHT
score = 0
# 开始游戏循环
while True:
next_key = window.getch()
key = key if next_key == -1 else next_key
# 计算蛇的下一个位置
snake_head = [snake[0][0], snake[0][1]]
if key == curses.KEY_DOWN:
snake_head[0] += 1
elif key == curses.KEY_UP:
snake_head[0] -= 1
elif key == curses.KEY_LEFT:
snake_head[1] -= 1
elif key == curses.KEY_RIGHT:
snake_head[1] += 1
snake.insert(0, snake_head)
# 判断蛇是否吃到了食物
if snake_head == food:
food = None
score += 1
while food is None:
new_food = [random.randint(1, screen_height - 1),
random.randint(1, screen_width - 1)]
food = new_food if new_food not in snake else None
window.addch(food[0], food[1], curses.ACS_PI)
else:
tail = snake.pop()
window.addch(tail[0], tail[1], ' ')
# 绘制蛇和食物
window.addch(snake[0][0], snake[0][1], curses.ACS_CKBOARD)
window.addch(snake[-1][0], snake[-1][1], curses.ACS_CKBOARD)
for i in range(1, len(snake) - 1):
window.addch(snake[i][0], snake[i][1], curses.ACS_BLOCK)
# 判断游戏是否结束
if (snake_head[0] in [0, screen_height - 1] or
snake_head[1] in [0, screen_width - 1] or
snake_head in snake[1:]):
msg = "Game over! Your score is %d" % score
window.addstr(screen_height // 2, (screen_width - len(msg)) // 2, msg)
window.refresh()
time.sleep(3)
break
# 结束游戏并关闭 curses 库
curses.endwin()
```
这段代码使用了 curses 库来实现了一个简单的贪吃蛇游戏。在运行代码之前,需要先安装 curses 库,你可以使用以下命令进行安装:
```
pip install windows-curses # Windows 系统上
pip install curses # Linux 或者 macOS 系统上
```
希望这个代码能够对你有所帮助!
相关推荐
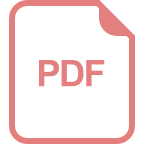
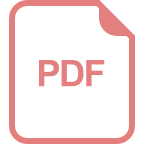
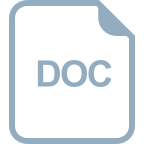











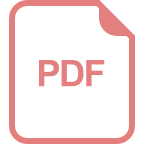
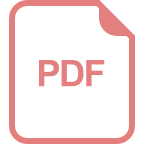
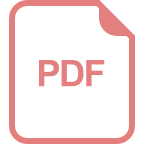
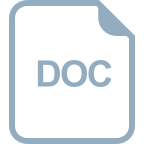