Write a C program that inputs a series of integers and passes them one at a time to function even, which uses the modulus operator to determine whether an integer is even. The function should take an integer argument and return true if the integer is even and false otherwise.
时间: 2024-02-14 10:12:12 浏览: 127
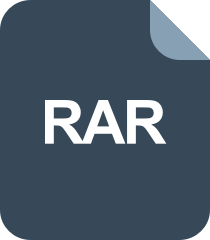
A MEASURE example uses the analog inputs of the AT91M55800A
Sure, here's a C program that inputs a series of integers and passes them one at a time to function even, which uses the modulus operator to determine whether an integer is even. The function takes an integer argument and returns true if the integer is even and false otherwise:
```c
#include <stdio.h>
#include <stdbool.h>
bool even(int num);
int main() {
int num;
printf("Enter a series of integers (enter 0 to stop):\n");
do {
scanf("%d", &num);
if (even(num)) {
printf("%d is even.\n", num);
}
else {
printf("%d is odd.\n", num);
}
} while (num != 0);
return 0;
}
bool even(int num) {
if (num % 2 == 0) {
return true;
}
else {
return false;
}
}
```
In this program, the main function first prompts the user to enter a series of integers. It then uses a do-while loop to repeatedly read in integers using scanf(), and pass them to the even function. The even function uses the modulus operator to determine whether the integer is even, and returns true if it is and false otherwise. Finally, the main function prints out whether each integer is even or odd using printf().
阅读全文
相关推荐
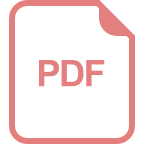













