Write a C program that inputs a series of integers and passes them one at a time to function even which uses the modulus operator to determine whether an integer is even.The function should take an integer argument and re
时间: 2024-02-14 13:16:20 浏览: 145
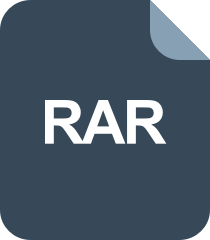
c语言开发的一个计算器程序
Sure, here's an example C program that takes a series of integers as input and passes them to a function called `even` that checks whether each integer is even or odd:
```c
#include <stdio.h>
int even(int num) {
if (num % 2 == 0) {
return 1;
}
else {
return 0;
}
}
int main() {
int num, result;
printf("Enter a series of integers (enter -1 to end):\n");
do {
scanf("%d", &num);
if (num != -1) {
result = even(num);
if (result == 1) {
printf("%d is even\n", num);
}
else {
printf("%d is odd\n", num);
}
}
} while (num != -1);
return 0;
}
```
In this program, we use a `do-while` loop to repeatedly prompt the user for input until they enter `-1`. Each integer entered by the user is passed to the `even` function, which uses the modulus operator (`%`) to determine whether the integer is even or odd. If the result is 0, the integer is odd; if the result is 1, the integer is even. The function returns either 1 or 0 depending on whether the integer is even or odd.
In the `main` function, we call `even` for each integer entered by the user and print a message indicating whether the integer is even or odd.
阅读全文
相关推荐













