解释这段代码int main() { int choice; while (true) { showMenu(); cout << "请选择操作:"; cin >> choice; switch (choice) { case 1: insertStudent(); break; case 2: deleteStudent(); break; case 3: modifyStudent(); break; case 4: sortByScore(); break; case 5: calculateAverageScore(); break; case 6: countFailedStudents(); break; case 7: sortByAverageScore(); break; case 8: printScoreTable(); break; case 9: saveToFile(); break; case 10: loadFromFile(); break; case 0: return 0; default: cout << "无效的选择!\n";
时间: 2024-02-10 19:34:52 浏览: 170
这段代码是一个简单的学生成绩管理系统的主函数。它使用一个无限循环,不断显示菜单并等待用户输入选项。当用户选择一个选项时,会调用相应的函数来进行相应的操作。
具体来说,这个系统支持以下操作:
1. 插入学生信息
2. 删除学生信息
3. 修改学生信息
4. 按成绩排序
5. 计算平均成绩
6. 统计不及格学生
7. 按平均成绩排序
8. 打印成绩表格
9. 保存到文件
10. 从文件加载
当用户选择退出时,主函数返回0,程序结束。如果用户输入了无效的选项,主函数会输出一个错误信息并重新显示菜单等待用户输入。
相关问题
编写一个售卖系统程序: (1)售卖系统中包括3项数据:商品名称、商品数量和商品价格 (2)对商品的录入、查询、删除、售卖、修改5项功能;(3)设计主控菜单,模块化设计,通过main函数调用;5(4)界面美观;用include代码实现
好的,我可以帮你编写一个售卖系统程序。以下是代码实现:
```
#include <iostream>
#include <string>
#include <vector>
using namespace std;
// 商品类
class Product {
private:
string name; // 商品名称
int quantity; // 商品数量
double price; // 商品价格
public:
// 构造函数
Product(string n = "", int q = 0, double p = 0.0) {
name = n;
quantity = q;
price = p;
}
// 获取商品名称
string getName() {
return name;
}
// 获取商品数量
int getQuantity() {
return quantity;
}
// 获取商品价格
double getPrice() {
return price;
}
// 设置商品名称
void setName(string n) {
name = n;
}
// 设置商品数量
void setQuantity(int q) {
quantity = q;
}
// 设置商品价格
void setPrice(double p) {
price = p;
}
// 输出商品信息
void print() {
cout << "商品名称:" << name << endl;
cout << "商品数量:" << quantity << endl;
cout << "商品价格:" << price << endl;
}
};
// 售卖系统类
class SalesSystem {
private:
vector<Product> products; // 商品列表
public:
// 添加商品
void addProduct() {
string name;
int quantity;
double price;
cout << "请输入商品名称:";
cin >> name;
cout << "请输入商品数量:";
cin >> quantity;
cout << "请输入商品价格:";
cin >> price;
Product product(name, quantity, price);
products.push_back(product);
cout << "商品添加成功!" << endl;
}
// 查询商品
void queryProduct() {
string name;
cout << "请输入要查询的商品名称:";
cin >> name;
bool found = false;
for (int i = 0; i < products.size(); i++) {
if (products[i].getName() == name) {
products[i].print();
found = true;
break;
}
}
if (!found) {
cout << "没有找到该商品!" << endl;
}
}
// 删除商品
void deleteProduct() {
string name;
cout << "请输入要删除的商品名称:";
cin >> name;
bool found = false;
for (int i = 0; i < products.size(); i++) {
if (products[i].getName() == name) {
products.erase(products.begin() + i);
found = true;
cout << "商品删除成功!" << endl;
break;
}
}
if (!found) {
cout << "没有找到该商品!" << endl;
}
}
// 售卖商品
void sellProduct() {
string name;
int quantity;
cout << "请输入要售卖的商品名称:";
cin >> name;
cout << "请输入要售卖的商品数量:";
cin >> quantity;
bool found = false;
for (int i = 0; i < products.size(); i++) {
if (products[i].getName() == name) {
if (products[i].getQuantity() >= quantity) {
products[i].setQuantity(products[i].getQuantity() - quantity);
cout << "商品售卖成功!" << endl;
found = true;
break;
} else {
cout << "商品数量不足!" << endl;
found = true;
break;
}
}
}
if (!found) {
cout << "没有找到该商品!" << endl;
}
}
// 修改商品
void modifyProduct() {
string name;
cout << "请输入要修改的商品名称:";
cin >> name;
bool found = false;
for (int i = 0; i < products.size(); i++) {
if (products[i].getName() == name) {
string newName;
int newQuantity;
double newPrice;
cout << "请输入新的商品名称:";
cin >> newName;
cout << "请输入新的商品数量:";
cin >> newQuantity;
cout << "请输入新的商品价格:";
cin >> newPrice;
products[i].setName(newName);
products[i].setQuantity(newQuantity);
products[i].setPrice(newPrice);
cout << "商品修改成功!" << endl;
found = true;
break;
}
}
if (!found) {
cout << "没有找到该商品!" << endl;
}
}
// 显示菜单
void showMenu() {
cout << "欢迎使用售卖系统!" << endl;
cout << "1. 添加商品" << endl;
cout << "2. 查询商品" << endl;
cout << "3. 删除商品" << endl;
cout << "4. 售卖商品" << endl;
cout << "5. 修改商品" << endl;
cout << "0. 退出系统" << endl;
}
// 运行系统
void run() {
int choice;
do {
showMenu();
cout << "请输入您的选择:";
cin >> choice;
switch (choice) {
case 1:
addProduct();
break;
case 2:
queryProduct();
break;
case 3:
deleteProduct();
break;
case 4:
sellProduct();
break;
case 5:
modifyProduct();
break;
case 0:
cout << "谢谢使用,再见!" << endl;
break;
default:
cout << "输入有误,请重新输入!" << endl;
break;
}
} while (choice != 0);
}
};
int main() {
SalesSystem salesSystem;
salesSystem.run();
return 0;
}
```
以上就是一个简单的售卖系统程序,包括商品类和售卖系统类,实现了添加商品、查询商品、删除商品、售卖商品、修改商品等功能,并且通过主控菜单进行模块化设计,界面简洁美观。
c++实验环境中,给出含有n个学生的成绩表,每条记录由学号、姓名和分数组成,编写具体函数完成下列操作:设计一个菜单操作界面,实现录入学生基本信息,直接插入排序,折半插入排序,冒泡排序,快速排序,简单选择排序,输出学生信息,退出等基本操作
好的,我可以帮你解答这个问题。
首先,需要定义一个学生结构体,包含学号、姓名和分数三个属性。
```c++
struct Student {
int id;
char name[20];
float score;
};
```
接着,需要定义一个动态数组,来存储学生信息。
```c++
Student* studentList = nullptr;
int studentCount = 0;
```
接下来,我们可以设计菜单操作界面,让用户选择相应的操作。
```c++
void showMenu() {
cout << "1. 录入学生信息" << endl;
cout << "2. 直接插入排序" << endl;
cout << "3. 折半插入排序" << endl;
cout << "4. 冒泡排序" << endl;
cout << "5. 快速排序" << endl;
cout << "6. 简单选择排序" << endl;
cout << "7. 输出学生信息" << endl;
cout << "8. 退出" << endl;
}
```
接下来,我们可以实现每个操作对应的具体函数。
```c++
// 录入学生信息
void inputStudent() {
cout << "请输入学生数量:" << endl;
cin >> studentCount;
studentList = new Student[studentCount];
for (int i = 0; i < studentCount; i++) {
cout << "请输入第" << i + 1 << "个学生的信息:" << endl;
cout << "学号:";
cin >> studentList[i].id;
cout << "姓名:";
cin >> studentList[i].name;
cout << "分数:";
cin >> studentList[i].score;
}
}
// 直接插入排序
void insertionSort() {
for (int i = 1; i < studentCount; i++) {
Student temp = studentList[i];
int j = i - 1;
while (j >= 0 && studentList[j].id > temp.id) {
studentList[j + 1] = studentList[j];
j--;
}
studentList[j + 1] = temp;
}
}
// 折半插入排序
void binaryInsertionSort() {
for (int i = 1; i < studentCount; i++) {
Student temp = studentList[i];
int left = 0, right = i - 1;
while (left <= right) {
int mid = (left + right) / 2;
if (studentList[mid].id > temp.id) {
right = mid - 1;
} else {
left = mid + 1;
}
}
for (int j = i - 1; j >= left; j--) {
studentList[j + 1] = studentList[j];
}
studentList[left] = temp;
}
}
// 冒泡排序
void bubbleSort() {
for (int i = 0; i < studentCount - 1; i++) {
for (int j = 0; j < studentCount - 1 - i; j++) {
if (studentList[j].id > studentList[j + 1].id) {
Student temp = studentList[j];
studentList[j] = studentList[j + 1];
studentList[j + 1] = temp;
}
}
}
}
// 快速排序
void quickSort(int left, int right) {
if (left >= right) {
return;
}
int i = left, j = right;
Student pivot = studentList[left];
while (i < j) {
while (i < j && studentList[j].id >= pivot.id) {
j--;
}
studentList[i] = studentList[j];
while (i < j && studentList[i].id <= pivot.id) {
i++;
}
studentList[j] = studentList[i];
}
studentList[i] = pivot;
quickSort(left, i - 1);
quickSort(i + 1, right);
}
// 简单选择排序
void selectionSort() {
for (int i = 0; i < studentCount - 1; i++) {
int minIndex = i;
for (int j = i + 1; j < studentCount; j++) {
if (studentList[j].id < studentList[minIndex].id) {
minIndex = j;
}
}
if (minIndex != i) {
Student temp = studentList[i];
studentList[i] = studentList[minIndex];
studentList[minIndex] = temp;
}
}
}
// 输出学生信息
void outputStudent() {
for (int i = 0; i < studentCount; i++) {
cout << studentList[i].id << "\t" << studentList[i].name << "\t" << studentList[i].score << endl;
}
}
// 退出程序
void exitProgram() {
delete[] studentList;
studentList = nullptr;
studentCount = 0;
exit(0);
}
```
最后,我们可以在主函数中调用菜单操作界面,根据用户选择调用相应的函数。
```c++
int main() {
while (true) {
showMenu();
int choice;
cin >> choice;
switch (choice) {
case 1:
inputStudent();
break;
case 2:
insertionSort();
break;
case 3:
binaryInsertionSort();
break;
case 4:
bubbleSort();
break;
case 5:
quickSort(0, studentCount - 1);
break;
case 6:
selectionSort();
break;
case 7:
outputStudent();
break;
case 8:
exitProgram();
break;
default:
cout << "输入有误,请重新输入!" << endl;
break;
}
}
return 0;
}
```
以上就是完整的代码实现,你可以根据自己的需要进行修改和优化。
阅读全文
相关推荐
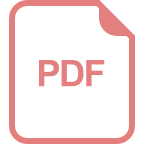
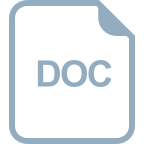
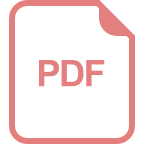














