基于dspic33系列单片机位置单闭环控制有刷直流电机正反转的程序,电机驱动芯片L298N利用霍尔传感器监测电机转速,电机不带编码器。
时间: 2023-11-14 09:05:30 浏览: 46
以下是基于dspic33系列单片机的有刷直流电机正反转控制程序,使用L298N电机驱动芯片和霍尔传感器进行转速监测。本程序假设使用的是两相有刷直流电机。
首先,需要定义一些常量和变量:
```c
#define PWM_PERIOD 3999 // PWM周期(Tcy * (PWM_PERIOD + 1) = 2us * 4000 = 8ms)
#define MAX_SPEED 100 // 最大转速(占空比)
#define MIN_SPEED 0 // 最小转速(占空比)
#define SPEED_STEP 10 // 转速变化步进值
#define HALL_SENSOR_MASK 0b111 // 霍尔传感器状态掩码
#define HALL_SENSOR_A_PIN _RB1 // 霍尔传感器A相引脚
#define HALL_SENSOR_B_PIN _RB2 // 霍尔传感器B相引脚
#define HALL_SENSOR_C_PIN _RB3 // 霍尔传感器C相引脚
int target_speed = 0; // 目标转速(占空比)
int current_speed = 0; // 当前转速(占空比)
int hall_sensor_state = 0; // 霍尔传感器状态
```
在主函数中,需要初始化IO口、PWM模块和霍尔传感器中断:
```c
void main()
{
// 初始化IO口
TRISBbits.TRISB0 = 0; // 控制方向的IO口
TRISBbits.TRISB1 = 1; // 霍尔传感器A相引脚
TRISBbits.TRISB2 = 1; // 霍尔传感器B相引脚
TRISBbits.TRISB3 = 1; // 霍尔传感器C相引脚
// 初始化PWM模块
PTCONbits.PTEN = 0; // 先禁用PWM模块
PTCONbits.PTMOD = 0b00; // PWM模式为边沿对齐模式
PTCONbits.PTCKPS = 0b00;// 分频系数为1
PTPER = PWM_PERIOD; // 设置PWM周期
PWMCON1bits.PEN1H = 1; // 使能PWM1H引脚
PWMCON1bits.PEN2H = 1; // 使能PWM2H引脚
PWMCON2bits.UDIS = 1; // 禁用死区时间
PTCONbits.PTEN = 1; // 启用PWM模块
// 初始化霍尔传感器中断
INTCON2bits.INT0EP = 0; // 下降沿触发中断
IFS0bits.INT0IF = 0; // 清除中断标志
IPC0bits.INT0IP = 5; // 设置中断优先级为5
IEC0bits.INT0IE = 1; // 使能中断
// 设置初始转速
current_speed = target_speed = MIN_SPEED;
set_motor_speed(current_speed);
while(1)
{
// 根据目标转速调整电机转速
if (current_speed < target_speed)
{
current_speed += SPEED_STEP;
if (current_speed > target_speed)
current_speed = target_speed;
set_motor_speed(current_speed);
}
else if (current_speed > target_speed)
{
current_speed -= SPEED_STEP;
if (current_speed < target_speed)
current_speed = target_speed;
set_motor_speed(current_speed);
}
}
}
```
其中,`set_motor_speed`函数用于设置PWM模块输出的占空比:
```c
void set_motor_speed(int speed)
{
if (speed < 0)
{
// 反转
_LATB0 = 1; // 设置方向引脚
speed = -speed;
}
else
{
// 正转
_LATB0 = 0; // 设置方向引脚
}
if (speed > MAX_SPEED)
speed = MAX_SPEED;
else if (speed < MIN_SPEED)
speed = MIN_SPEED;
PDC1 = PWM_PERIOD * speed / 100; // 设置PWM1H引脚占空比
PDC2 = PWM_PERIOD * speed / 100; // 设置PWM2H引脚占空比
}
```
最后,还需要编写一个中断服务程序来处理霍尔传感器中断:
```c
void __attribute__((interrupt, no_auto_psv)) _INT0Interrupt(void)
{
hall_sensor_state = (hall_sensor_state << 1) & HALL_SENSOR_MASK;
if (!HALL_SENSOR_A_PIN)
hall_sensor_state |= 0b001;
if (!HALL_SENSOR_B_PIN)
hall_sensor_state |= 0b010;
if (!HALL_SENSOR_C_PIN)
hall_sensor_state |= 0b100;
// 根据霍尔传感器状态计算电机转速
switch (hall_sensor_state)
{
case 0b001:
case 0b101:
target_speed = MAX_SPEED; // 正转最大速度
break;
case 0b010:
case 0b011:
target_speed = -MAX_SPEED; // 反转最大速度
break;
case 0b100:
case 0b110:
case 0b111:
target_speed = 0; // 停止
break;
default:
break;
}
IFS0bits.INT0IF = 0; // 清除中断标志
}
```
以上就是基于dspic33系列单片机的有刷直流电机正反转控制程序,使用L298N电机驱动芯片和霍尔传感器进行转速监测。由于没有编码器,本程序使用霍尔传感器的状态来估算电机转速。注意,在实际使用中需要根据电机和霍尔传感器的具体参数进行调整。
相关推荐
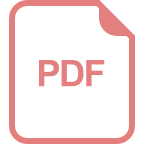
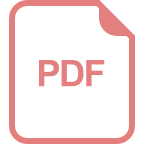
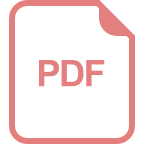














