int listlength(slnode *head){ slnode *p=head; int size=0; while(p->next!=NULL) { size++; p=p->next; } return size; }
时间: 2024-03-15 21:43:31 浏览: 96
这是一个函数,名为 `listlength`,它接受一个指向单向链表头结点的指针 `head` 作为参数,并返回链表中节点的数量。
函数的实现过程如下:
1. 定义一个指向头结点的指针 `p`,并将其初始化为 `head`。
2. 定义一个整型变量 `size`,并将其初始化为 0。
3. 进入循环,循环条件为 `p` 的下一个节点不为 `NULL`。
4. 在循环体内,将 `size` 自增 1,表示当前节点是链表中的一个节点。
5. 将 `p` 指向下一个节点,继续遍历链表。
6. 当循环结束时,返回 `size`,即链表中节点的数量。
需要注意的是,这个函数没有考虑头结点本身是否为链表中的节点,因此在计算链表长度时,头结点也会被算作一个节点。如果头结点不算作链表中的节点,需要在循环体内将 `size` 初始化为 1。
相关问题
void fun2(SeqList *1,int e) {LinkQueue q;q= InitQueueO; for (i=0; i<l->listlength; i++) if (1->elem[i] != e) AddQueue(&q,l->elem[i]); 1->listlength= 1->listlength-1; for (i=0; i< 1->listlength; i++) 1->elem[i]= DeleteQueue(&q); }算法的功能
q, 1->elem[i]); while (!QueueEmpty(q)) {printf("%d ",FrontQueue(q));DeleteQueue(q);} printf("\n");}
This code defines a function named "fun2" that takes in a pointer to a SeqList (which is a structure containing an array of integers and some other information) and an integer "e".
The function initializes a LinkQueue named "q" using the function InitQueueO, which is not provided in this code snippet. The function then iterates over the elements in the SeqList (denoted by "1"), checking to see which elements are not equal to "e". For each element that is not equal to "e", the function adds that element to the queue "q" using the function AddQueue.
Once all of the elements in the SeqList have been processed, the function enters a loop that continues while "q" is not empty. In each iteration of the loop, the function prints the front element of the queue using the function FrontQueue, which returns the value of the front element without removing it. The function then removes the front element from the queue using the function DeleteQueue.
After all of the elements have been printed and removed from the queue, the function prints a newline character ("\n").
LinkList joseph(LinkList S,int m) { int i,len; LinkList p; i=1; len=ListLength(S); p=S; while(p->next!=S) p=p->next;//把p指向第一个结点的前驱 while(len>1){
这是一个约瑟夫问题的解法,使用循环链表来实现。具体实现细节可以参考代码:
LinkList joseph(LinkList S, int m) {
int i, len;
LinkList p, q;
i = 1;
len = ListLength(S);
p = S;
while (p->next != S) p = p->next; // 把p指向第一个结点的前驱
while (len > 1) {
for (i = 1; i < m; i++) {
p = p->next;
}
q = p->next;
p->next = q->next;
len--;
free(q);
}
return p;
}
这个函数的作用是解决约瑟夫问题,其中参数S是一个循环链表的头结点,m是每次删除的数的个数。函数返回最后剩下的结点。
注意,这个函数的实现并不完整,需要自己实现一些辅助函数,比如ListLength等。
阅读全文
相关推荐
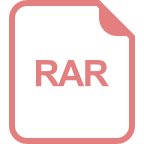
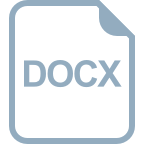
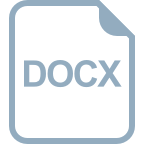














