编写一个使用matlab语言做矩阵lu分解的代码
时间: 2024-02-03 14:02:41 浏览: 75
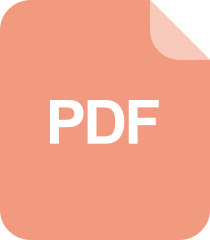
MATLAB中矩阵LU分解.pdf
好的,以下是一个简单的使用Matlab实现矩阵LU分解的代码:
```matlab
function [L, U] = lu_decomp(A)
% LU分解
% A: 要分解的矩阵
% L: 下三角矩阵
% U: 上三角矩阵
n = size(A, 1);
L = eye(n);
U = zeros(n);
for k = 1:n
U(k, k:end) = A(k, k:end) - L(k, 1:k-1) * U(1:k-1, k:end);
L(k+1:end, k) = (A(k+1:end, k) - L(k+1:end, 1:k-1) * U(1:k-1, k)) / U(k, k);
end
end
```
使用方法:
假设要对一个3x3的矩阵A进行LU分解,只需要调用该函数即可:
```matlab
A = [1 2 3; 4 5 6; 7 8 9];
[L, U] = lu_decomp(A);
```
该代码会返回下三角矩阵L和上三角矩阵U。
阅读全文
相关推荐
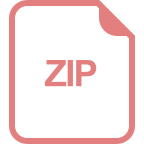




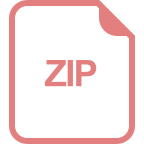
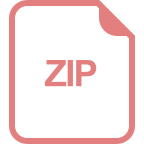
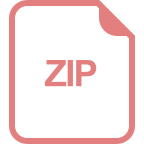
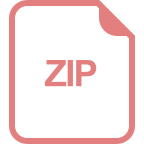
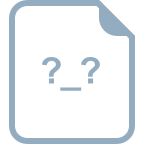
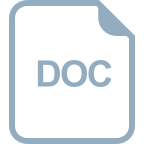
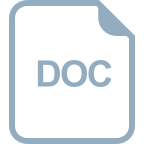




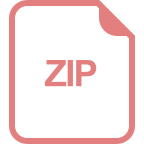