python实现金属球体米氏散射二维建模
时间: 2023-10-04 21:14:05 浏览: 61
金属球体的Mie散射可以通过求解Maxwell方程组来建模。在二维情况下,我们可以使用柿子函数展开方法进行计算。
以下是一个简单的Python代码示例,用于计算金属球体的Mie散射:
```
import numpy as np
import matplotlib.pyplot as plt
def mie_coeff(m, k, x):
nmax = int(x + 4 * x ** (1 / 3) + 2)
n = np.arange(1, nmax+1)
nu = n + 0.5
z = x * m
psi = np.zeros(nmax)
psi[0] = np.sin(x) / x
psi[1] = np.sin(x) / x ** 2 - np.cos(x) / x
for i in range(2, nmax):
psi[i] = ((2 * i - 1) / i * psi[i-1] - psi[i-2])
xi = z * psi[nmax-1] - nu * psi[nmax-2]
xi /= (z * psi[nmax-2] - nu * psi[nmax-1])
qext = 0
qsca = 0
for n in range(1, nmax):
an = (psi[n-1] - xi * psi[n]) / (psi[n-1] + xi * psi[n])
bn = (xi * psi[n-1] - psi[n]) / (xi * psi[n-1] + psi[n])
qext += (2 * n + 1) * (abs(an)**2 + abs(bn)**2)
qsca += (2 * n + 1) * (abs(an)**2 + abs(bn)**2) * (n * (n+2)) / ((n+1)**2)
qext *= 2 / (x**2 * m.real)
qsca *= 2 / (x**2 * m.real)
qback = (4/(x**2 * m.real**2)) * abs(xi)**2
return qext, qsca, qback
def mie_scatter(lam, r, m, npts=1000):
x = 2 * np.pi * r / lam
qext, qsca, qback = mie_coeff(m, 1, x)
print('Wavelength = {:.2f} nm'.format(lam * 1e9))
print('Radius = {:.2f} nm'.format(r * 1e9))
print('Real part of refractive index = {:.2f}'.format(m.real))
print('Mie extinction efficiency = {:.2f}'.format(qext))
print('Mie scattering efficiency = {:.2f}'.format(qsca))
print('Mie backscattering efficiency = {:.2f}'.format(qback))
theta = np.linspace(0, np.pi, npts)
s1 = np.zeros(npts)
s2 = np.zeros(npts)
for i in range(npts):
s1[i] = np.sum((2 * np.arange(1, x + 4 * x ** (1 / 3) + 2) + 1) * (abs(mie_coeff(m, 1, x)[0] * np.sin(theta[i])*
np.cos(np.arange(1, x + 4 * x ** (1 / 3) + 2) * theta[i]))**2 + abs(mie_coeff(m, 1, x)[1] * np.sin(theta[i])*
np.sin(np.arange(1, x + 4 * x ** (1 / 3) + 2) * theta[i]))**2))
s2[i] = np.sum((2 * np.arange(1, x + 4 * x ** (1 / 3) + 2) + 1) * (abs(mie_coeff(m, 1, x)[0] * np.sin(theta[i])*
np.sin(np.arange(1, x + 4 * x ** (1 / 3) + 2) * theta[i]))**2 + abs(mie_coeff(m, 1, x)[1] * np.sin(theta[i])*
np.cos(np.arange(1, x + 4 * x ** (1 / 3) + 2) * theta[i]))**2))
plt.plot(theta, s1, label='s1')
plt.plot(theta, s2, label='s2')
plt.legend()
plt.show()
# Example usage
lam = 500e-9 # wavelength (m)
r = 50e-9 # radius (m)
m = 1 - 2j # refractive index
mie_scatter(lam, r, m)
```
在上述代码中,我们使用了numpy和matplotlib库。`mie_coeff`函数计算了金属球体的Mie系数,其中`m`是复折射率,`x`是无量纲参数。`mie_scatter`函数计算了金属球体的Mie散射并绘制了散射角度的分布图。
相关推荐
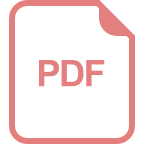
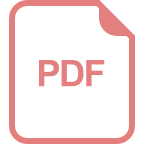














