人的 DNA 和病毒的 DNA 均表示成由一些字母组成的字符串序列。检测某种病毒的 DNA 序列是否在患者的 DNA 序列中出现过,如果出现过,则此人感染了该病毒,否则没有感染C语言代码
时间: 2024-11-12 12:32:54 浏览: 8
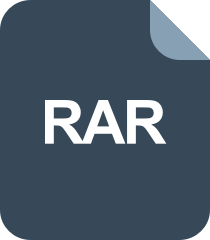
病毒感染检测.rar
在C语言中,用于检测DNA序列相似性的常见方法之一是KMP(Knuth-Morris-Pratt)算法或Boyer-Moore算法,它们主要用于字符串匹配查找。不过,由于DNA数据量庞大,实际应用通常会借助于更为高效的生物信息学库,如BLAST (Basic Local Alignment Search Tool) 或 BioPython等。
以下是一个简化版的KMP算法示例,用于演示基本思路,但请注意这不适合处理大型DNA数据:
```c
#include <stdio.h>
#include <string.h>
void compute_lps(char *pattern, int lps[]) {
int len = strlen(pattern);
lps[0] = 0;
for(int i = 1; i < len; ++i) {
if(pattern[i] == pattern[lps[i - 1]]) {
lps[i] = lps[i - 1] + 1;
} else {
if(lps[i - 1] != 0) {
lps[i] = lps[i - 1];
} else {
lps[i] = 0;
}
}
}
}
int kmp_search(char text[], char pattern[], int n, int m) {
int lps[m]; // longest prefix suffix array
compute_lps(pattern, lps);
int i = 0, j = 0;
while(i < n && j < m) {
if(text[i] == pattern[j]) {
i++;
j++;
} else {
if(j != 0) {
j = lps[j - 1];
} else {
i++;
}
}
}
if(j == m) return i - j; // Return index if pattern found
return -1; // Pattern not found
}
int main() {
char patient_dna[] = "..."
char virus_dna[] = "..."; // replace with actual DNA sequences
int index = kmp_search(patient_dna, virus_dna, strlen(patient_dna), strlen(virus_dna));
if(index != -1)
printf("Patient is infected with the virus.\n");
else
printf("Patient does not have the virus.\n");
return 0;
}
```
注意:这个代码仅作为一个概念展示,真实场景下会涉及更复杂的文件输入、预处理以及错误处理,并且需要使用专业的生物信息学库来进行大数据处理。
阅读全文
相关推荐
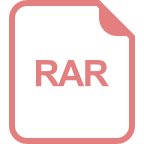
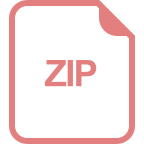



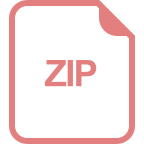
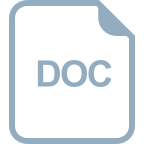


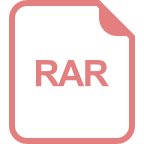
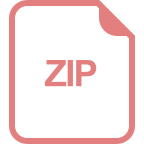
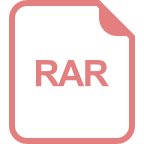
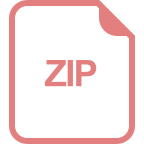
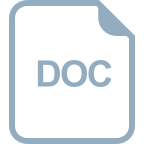




