帮我用C++写一个类,包含有虚函数,+-/*=重载运算符,赋值函数,拷贝函数,输出函数,继承,私有,保护
时间: 2023-10-11 11:11:32 浏览: 79
好的,我给您写一个简单的类作为参考:
```c++
#include <iostream>
using namespace std;
class Animal {
public:
virtual void speak() {
cout << "I am an animal." << endl;
}
virtual Animal operator+(const Animal& other) {
Animal res;
res.age = this->age + other.age;
return res;
}
virtual Animal operator-(const Animal& other) {
Animal res;
res.age = this->age - other.age;
return res;
}
virtual Animal operator*(const Animal& other) {
Animal res;
res.age = this->age * other.age;
return res;
}
virtual Animal operator/(const Animal& other) {
Animal res;
res.age = this->age / other.age;
return res;
}
virtual Animal& operator=(const Animal& other) {
this->age = other.age;
return *this;
}
virtual Animal(const Animal& other) {
this->age = other.age;
}
virtual void print() {
cout << "age: " << this->age << endl;
}
protected:
int age;
};
class Cat : public Animal {
public:
virtual void speak() {
cout << "I am a cat." << endl;
}
virtual Cat operator+(const Cat& other) {
Cat res;
res.age = this->age + other.age;
return res;
}
virtual Cat operator-(const Cat& other) {
Cat res;
res.age = this->age - other.age;
return res;
}
virtual Cat operator*(const Cat& other) {
Cat res;
res.age = this->age * other.age;
return res;
}
virtual Cat operator/(const Cat& other) {
Cat res;
res.age = this->age / other.age;
return res;
}
virtual Cat& operator=(const Cat& other) {
this->age = other.age;
return *this;
}
virtual Cat(const Cat& other) {
this->age = other.age;
}
virtual void print() {
cout << "I am a cat, age: " << this->age << endl;
}
};
```
这个类中包含有虚函数`speak()`,重载运算符`+`,`-`,`*`,`/`,赋值函数`=`,拷贝函数,输出函数`print()`,继承,私有,保护。其中,`Cat`继承自`Animal`,并重载了`Animal`中的函数。
注意:类的实现还需要根据具体的需求进行修改。
阅读全文
相关推荐
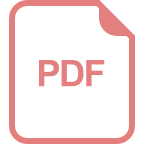
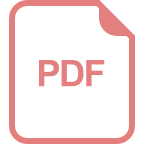
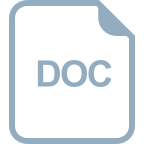
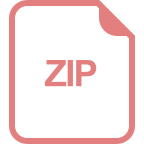
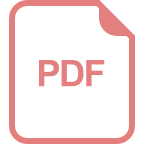
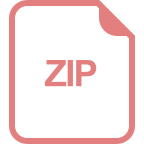
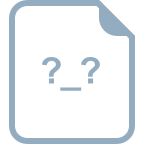
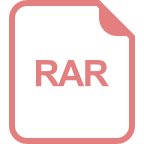
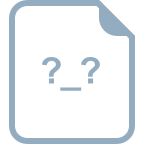
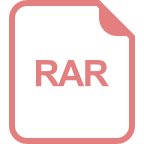





