我自己做的一个raycastVehicle汽车,但是它的轮胎会有一部分再地面之下,就好像没有碰撞,直接穿透了一样,使用applyEngineForce时,穿到地面下的轮胎可以转动,其他的不行,然后有时候一直移动和转向可以将车胎移到地面上
时间: 2023-09-22 18:06:36 浏览: 43
这个问题可能是由于碰撞体的设置或车轮位置的设置不正确导致的。您可以尝试以下几个步骤来解决这个问题:
1. 确保车轮的位置设置正确。车轮的位置应该是相对于车辆的局部坐标系的。您可以在车辆的构造函数中设置每个车轮的位置:
```
// 设置前轮的位置
btVector3 connectionPointCS0(frontWheelPosition.x, frontWheelPosition.y, frontWheelPosition.z);
// 创建车轮
btRaycastVehicle::btVehicleTuning tuning;
btVehicleRaycaster* raycaster = new btDefaultVehicleRaycaster(dynamicsWorld);
btRaycastVehicle* vehicle = new btRaycastVehicle(tuning, chassis, raycaster);
// 设置车轮
vehicle->addWheel(connectionPointCS0, wheelDirectionCS0, wheelAxleCS, suspensionRestLength, wheelRadius, tuning, true);
```
2. 确保车辆的碰撞体设置正确。车辆的碰撞体应该与车辆的形状相对应。您可以在车辆的构造函数中设置车辆的碰撞体:
```
// 创建车辆的碰撞体
btCompoundShape* compound = new btCompoundShape();
btCollisionShape* chassisShape = new btBoxShape(btVector3(chassisWidth * 0.5f, chassisHeight * 0.5f, chassisLength * 0.5f));
compound->addChildShape(btTransform::getIdentity(), chassisShape);
// 设置车辆的质量
btScalar mass = 1000.0f;
btVector3 localInertia(0, 0, 0);
compound->calculateLocalInertia(mass, localInertia);
// 创建车辆的刚体
btDefaultMotionState* motionState = new btDefaultMotionState(btTransform(btQuaternion(0, 0, 0, 1), btVector3(0, 0, 0)));
btRigidBody::btRigidBodyConstructionInfo rbInfo(mass, motionState, compound, localInertia);
btRigidBody* chassis = new btRigidBody(rbInfo);
```
3. 在更新车轮时,确保每个车轮都处于正确的位置:
```
// 更新车轮
for (int i = 0; i < vehicle->getNumWheels(); i++)
{
btWheelInfo& wheel = vehicle->getWheelInfo(i);
wheel.m_suspensionTravelCm = suspensionTravelCm;
wheel.m_maxSuspensionForce = maxSuspensionForce;
wheel.m_wheelsDampingCompression = suspensionCompression;
wheel.m_wheelsDampingRelaxation = suspensionRelaxation;
wheel.m_rollInfluence = rollInfluence;
// 设置车轮位置
btTransform transform = vehicle->getChassisWorldTransform();
btVector3 position = transform.getOrigin();
position += transform.getBasis() * wheel.m_chassisConnectionPointCS;
position += transform.getBasis() * btVector3(0, -wheel.m_suspensionTravelCm * 0.01f, 0);
wheel.m_raycastInfo.m_hardPointWS = position;
wheel.m_raycastInfo.m_wheelDirectionWS = transform.getBasis() * wheel.m_wheelDirectionCS;
wheel.m_raycastInfo.m_wheelAxleWS = transform.getBasis() * wheel.m_wheelAxleCS;
wheel.m_raycastInfo.m_worldTransform.setIdentity();
wheel.m_raycastInfo.m_worldTransform.setOrigin(wheel.m_raycastInfo.m_hardPointWS);
wheel.m_raycastInfo.m_worldTransform.getBasis().setValue(
wheel.m_wheelDirectionCS.getX(), wheel.m_wheelDirectionCS.getY(), wheel.m_wheelDirectionCS.getZ(),
wheel.m_wheelAxleCS.getX(), wheel.m_wheelAxleCS.getY(), wheel.m_wheelAxleCS.getZ(),
0, 0, 0);
}
```
通过以上步骤,您应该能够解决车轮穿透地面的问题。
相关推荐
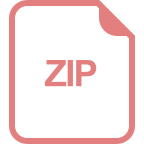
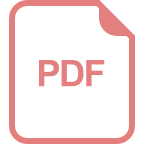














