请给出IO中断模拟IIC从机GD32F130的例程
时间: 2023-10-05 18:04:36 浏览: 146
以下是IO中断模拟IIC从机GD32F130的例程,请参考:
```C++
#include "gd32f1x0.h"
#include "stdio.h"
#include "systick.h"
#define I2C2_SCL_PIN GPIO_PIN_6 /*!< GPIO pin of the I2C clock */
#define I2C2_SCL_GPIO_PORT GPIOB /*!< GPIO port of the I2C clock */
#define I2C2_SDA_PIN GPIO_PIN_7 /*!< GPIO pin of the I2C data */
#define I2C2_SDA_GPIO_PORT GPIOB /*!< GPIO port of the I2C data */
#define I2C2_RCC_CLK_ENABLE() rcu_periph_clock_enable(RCU_GPIOB) /*!< Enable GPIOB clock */
#define I2C2_RCC_CLK_DISABLE() rcu_periph_clock_disable(RCU_GPIOB) /*!< Disable GPIOB clock */
#define I2C2_SCL_HIGH() gpio_bit_set(I2C2_SCL_GPIO_PORT, I2C2_SCL_PIN) /*!< Set GPIOB pin 6 */
#define I2C2_SCL_LOW() gpio_bit_reset(I2C2_SCL_GPIO_PORT, I2C2_SCL_PIN) /*!< Reset GPIOB pin 6 */
#define I2C2_SDA_HIGH() gpio_bit_set(I2C2_SDA_GPIO_PORT, I2C2_SDA_PIN) /*!< Set GPIOB pin 7 */
#define I2C2_SDA_LOW() gpio_bit_reset(I2C2_SDA_GPIO_PORT, I2C2_SDA_PIN) /*!< Reset GPIOB pin 7 */
#define I2C2_SDA_READ() gpio_input_bit_get(I2C2_SDA_GPIO_PORT, I2C2_SDA_PIN) /*!< Read GPIOB pin 7 */
void delay(uint32_t count)
{
uint32_t index = 0;
for(index = count; index != 0; index--)
{
}
}
void I2C2_GPIO_Config(void)
{
rcu_periph_clock_enable(RCU_AF);
rcu_periph_clock_enable(RCU_GPIOB);
/*configure GPIO pin of I2C2*/
gpio_init(I2C2_SCL_GPIO_PORT, GPIO_MODE_OUT_OD, GPIO_OSPEED_50MHZ, I2C2_SCL_PIN);
gpio_init(I2C2_SDA_GPIO_PORT, GPIO_MODE_OUT_OD, GPIO_OSPEED_50MHZ, I2C2_SDA_PIN);
/*configure I2C2 as a master*/
gpio_init(I2C2_SCL_GPIO_PORT, GPIO_MODE_AF_OD, GPIO_OSPEED_50MHZ, I2C2_SCL_PIN);
gpio_init(I2C2_SDA_GPIO_PORT, GPIO_MODE_AF_OD, GPIO_OSPEED_50MHZ, I2C2_SDA_PIN);
gpio_pin_remap_config(GPIO_SPI2_REMAP,I2C2_MAP);
}
void I2C2_Start(void)
{
I2C2_SDA_HIGH();
I2C2_SCL_HIGH();
delay(5);
I2C2_SDA_LOW();
delay(5);
I2C2_SCL_LOW();
}
void I2C2_Stop(void)
{
I2C2_SDA_LOW();
I2C2_SCL_HIGH();
delay(5);
I2C2_SDA_HIGH();
delay(5);
}
uint8_t I2C2_WaitAck(void)
{
uint8_t ucErrTime = 0;
I2C2_SDA_HIGH();
delay(1);
I2C2_SCL_HIGH();
delay(1);
while(I2C2_SDA_READ())
{
ucErrTime++;
if(ucErrTime > 50)
{
I2C2_Stop();
return 1;
}
delay(1);
}
I2C2_SCL_LOW();
return 0;
}
void I2C2_Ack(void)
{
I2C2_SCL_LOW();
I2C2_SDA_LOW();
delay(2);
I2C2_SCL_HIGH();
delay(2);
I2C2_SCL_LOW();
}
void I2C2_NAck(void)
{
I2C2_SCL_LOW();
I2C2_SDA_HIGH();
delay(2);
I2C2_SCL_HIGH();
delay(2);
I2C2_SCL_LOW();
}
void I2C2_SendByte(uint8_t ucData)
{
uint8_t ucCount = 0;
for(ucCount = 0; ucCount < 8; ucCount++)
{
if(ucData & 0x80)
I2C2_SDA_HIGH();
else
I2C2_SDA_LOW();
ucData <<= 1;
delay(2);
I2C2_SCL_HIGH();
delay(2);
I2C2_SCL_LOW();
}
}
uint8_t I2C2_ReadByte(uint8_t ucAck)
{
uint8_t ucCount = 0, ucData = 0;
I2C2_SDA_HIGH();
for(ucCount = 0; ucCount < 8; ucCount++)
{
ucData <<= 1;
I2C2_SCL_LOW();
delay(2);
I2C2_SCL_HIGH();
delay(2);
if(I2C2_SDA_READ())
ucData++;
}
if(!ucAck)
I2C2_NAck();
else
I2C2_Ack();
return ucData;
}
void GD32_I2C2_Init(void)
{
I2C2_GPIO_Config();
}
uint8_t GD32_I2C2_ReadByte(uint8_t ucAddr, uint8_t ucReg)
{
uint8_t ucData = 0;
I2C2_Start();
I2C2_SendByte(ucAddr << 1 | 0);
if(I2C2_WaitAck())
{
I2C2_Stop();
return 0;
}
I2C2_SendByte(ucReg);
I2C2_WaitAck();
I2C2_Start();
I2C2_SendByte(ucAddr << 1 | 1);
I2C2_WaitAck();
ucData = I2C2_ReadByte(0);
I2C2_Stop();
return ucData;
}
void GD32_I2C2_WriteByte(uint8_t ucAddr, uint8_t ucReg, uint8_t ucValue)
{
I2C2_Start();
I2C2_SendByte(ucAddr << 1 | 0);
if(I2C2_WaitAck())
{
I2C2_Stop();
return;
}
I2C2_SendByte(ucReg);
I2C2_WaitAck();
I2C2_SendByte(ucValue);
I2C2_WaitAck();
I2C2_Stop();
}
int main(void)
{
uint8_t ucData = 0;
GD32_I2C2_Init();
while(1)
{
ucData = GD32_I2C2_ReadByte(0x68, 0x75);
printf("WHO_AM_I is %X \r\n", ucData);
delay_1ms(1000);
}
}
```
阅读全文
相关推荐
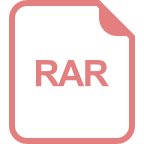
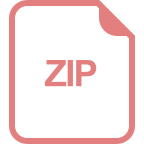
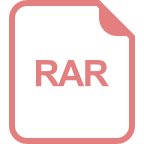















