用stm32写一个无感foc算法
时间: 2023-12-09 16:06:12 浏览: 75
好的,以下是一个简单的基于STM32的无感FOC算法示例,使用STM32 HAL库:
```c
#include "stm32f1xx_hal.h"
// 定义FOC参数
float Kp = 1.0;
float Ki = 0.5;
float Kd = 0.2;
// 定义FOC变量
float Setpoint = 0.0;
float Current_A = 0.0;
float Current_B = 0.0;
float Voltage_A = 0.0;
float Voltage_B = 0.0;
float Theta = 0.0;
float Theta_Est = 0.0;
float Theta_Diff = 0.0;
float Integral = 0.0;
float Last_Error = 0.0;
float Torque = 0.0;
// 定义FOC限制
float Voltage_Max = 24.0;
float Voltage_Min = -24.0;
// 定义FOC计算时间间隔
uint32_t Interval = 10;
// 定义FOC计算函数
void FOC_Update(float setpoint, float current_a, float current_b)
{
// 计算电角度
Theta += (2.0 * M_PI * Interval) / 1000000.0;
// 计算电流矢量
float I_alpha = current_a * cos(Theta) + current_b * sin(Theta);
float I_beta = -current_a * sin(Theta) + current_b * cos(Theta);
// 估算转子电角度
Theta_Est += Theta_Diff;
Theta_Diff = Kp * (I_alpha * sin(Theta_Est) - I_beta * cos(Theta_Est)) + Ki * Integral - Kd * Theta_Diff;
Integral += Theta_Diff * Interval;
// 计算电动势矢量
float V_alpha = Kp * (setpoint - I_alpha) + Ki * Integral - Kd * (I_alpha - Last_Error);
float V_beta = Kp * (-I_beta) + Ki * Integral - Kd * (-I_beta);
// 计算电压矢量
Voltage_A = V_alpha * cos(Theta) - V_beta * sin(Theta);
Voltage_B = V_alpha * sin(Theta) + V_beta * cos(Theta);
// 限制电压矢量
if (Voltage_A > Voltage_Max) {
Voltage_A = Voltage_Max;
} else if (Voltage_A < Voltage_Min) {
Voltage_A = Voltage_Min;
}
if (Voltage_B > Voltage_Max) {
Voltage_B = Voltage_Max;
} else if (Voltage_B < Voltage_Min) {
Voltage_B = Voltage_Min;
}
// 计算电磁转矩
Torque = I_alpha * Voltage_B - I_beta * Voltage_A;
// 保存误差
Last_Error = I_alpha;
}
int main(void)
{
HAL_Init();
while (1) {
// 读取电流传感器数据
Current_A = read_sensor_a();
Current_B = read_sensor_b();
// 计算FOC输出
FOC_Update(Setpoint, Current_A, Current_B);
// 控制电机
control_motor(Voltage_A, Voltage_B);
// 延时
HAL_Delay(Interval);
}
}
```
在上面的代码中,我们定义了FOC参数、变量和限制,然后定义了一个FOC计算函数`FOC_Update()`,该函数接受设定点和电流矢量作为参数,并计算出电压矢量和电磁转矩。我们在主函数中循环调用该函数,从电流传感器读取电流矢量并控制电机。在每次循环中,我们还使用HAL_Delay()函数进行延时,以保证每次计算的时间间隔是固定的。需要注意的是,该示例代码中的FOC算法是基于三相电机的,如果你的电机是单相或双相的,需要进行相应的修改。
相关推荐
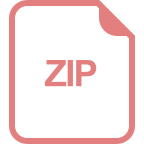
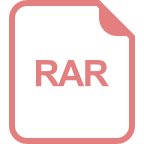
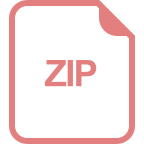


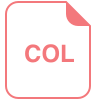
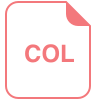
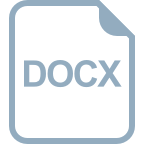
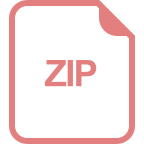
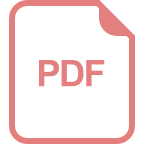
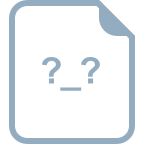
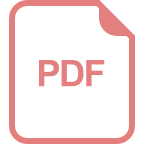
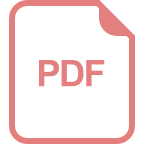
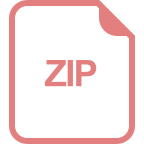