分析代码的每一行,#include<stdio.h> int main() { int t1,t2; int i,j; int test[1024][1024]={0}; t1=clock(); for(i=0;i<1024;i++) for(j=0;j<1024;j++) test[i][j]=0; t2=clock(); printf("......"":%d...\n",t2-t1); t1=clock(); for(i=0;i<1024;i++) for(j=0;j<1024;j++) test[j][i]=0; t2=clock(); printf("......"":%d...\n",t2-t1); return 0; }
时间: 2023-06-23 12:03:56 浏览: 40
这段代码主要是测试二维数组在不同存储方式下的效率。
第1行是包含了标准输入输出头文件。
第2行是定义了主函数,返回类型为整型。
第3和4行分别定义了两个整型变量 t1 和 t2。
第5和6行分别定义了两个整型变量 i 和 j。
第7行定义了一个二维数组 test,大小为 1024*1024,并将所有元素初始化为 0。
第8行记录当前时间到 t1 变量中。
第9到11行是两个嵌套循环,将数组中所有元素都重新赋值为 0。
第12行记录当前时间到 t2 变量中。
第13行输出从 t1 到 t2 的时间差,即第一个 for 循环的时间。
第14行再次记录当前时间到 t1 变量中。
第15到17行是另外两个嵌套循环,将数组中所有元素按列优先顺序赋值为 0。
第18行记录当前时间到 t2 变量中。
第19行输出从 t1 到 t2 的时间差,即第二个 for 循环的时间。
第20行结束主函数,并返回 0。
相关问题
#include <stdio.h> #include <stdlib.h> #include <pthread.h> int sum1 = 0, sum2 = 0; void *p1(){ int i, tmp = 0; for (i = 1; i <= 100; i++) tmp += i; sum1 += tmp; } void *p2(){ int i, tmp = 0; for (i = 101; i <= 200; i++) tmp += i; sum2 += tmp; } void p3(){ printf("sum: %d\n", sum1 + sum2); } int main(){ int res; pthread_t t1; pthread_t t2; //像这里,就是开一个新线程 void *thread_result; res = pthread_create(&t1, NULL, p1, NULL); if (res != 0){ perror("failed to create thread"); exit(1); } res = pthread_create(&t2, NULL, p2, NULL); //在这里,加一个线程,就是开一个新的。 if (res != 0){ perror("failed to join thread"); exit(2); } res = pthread_join(t1, &thread_result); res = pthread_join(t2, &thread_result); //这里再加这个 p3(); return 0; }解释此代码
这段代码的功能是创建两个线程,分别计算1到100和101到200的整数的和,然后将两个和相加并输出。代码中定义了三个函数p1、p2和p3,分别用于计算1到100、101到200的整数的和和输出和的结果。在主函数中,先创建两个线程并分别执行p1和p2函数,将计算结果存储在sum1和sum2变量中。然后通过pthread_join函数等待线程执行完毕。最后调用p3函数输出两个和的和。
需要注意的是,代码中的p1、p2和p3函数应该是返回void指针类型的,而不是不带返回值的void类型。另外,在创建第二个线程时,错误处理中的错误信息应该是"failed to create thread",而不是"failed to join thread"。代码中还存在一些不规范的写法,比如函数参数不加类型声明,缺少函数声明等。建议在编写代码时注意代码的规范性和安全性。以下是经过修改后的代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
int sum1 = 0, sum2 = 0;
void *p1(void *arg){
int i, tmp = 0;
for (i = 1; i <= 100; i++)
tmp += i;
sum1 += tmp;
return NULL;
}
void *p2(void *arg){
int i, tmp = 0;
for (i = 101; i <= 200; i++)
tmp += i;
sum2 += tmp;
return NULL;
}
void p3(){
printf("sum: %d\n", sum1 + sum2);
}
int main(){
int res;
pthread_t t1, t2;
void *thread_result;
res = pthread_create(&t1, NULL, p1, NULL);
if (res != 0){
perror("failed to create thread");
exit(1);
}
res = pthread_create(&t2, NULL, p2, NULL);
if (res != 0){
perror("failed to create thread");
exit(1);
}
res = pthread_join(t1, &thread_result);
if (res != 0){
perror("failed to join thread");
exit(2);
}
res = pthread_join(t2, &thread_result);
if (res != 0){
perror("failed to join thread");
exit(2);
}
p3();
return 0;
}
```
#include<stdio.h> #include<malloc.h> #define MaxSize 10 //最大人数 /* 成员信息 */ typedef struct Member { int Number; char ID[20]; char Sex[4]; int Age; char Birthday[20]; }Mem; typedef struct SeqList *PtrToSeqList; struct SeqList { Mem Data[MaxSize]; int count; //当前人数 }; typedef PtrToSeqList List; void PrintMenu(); //打印菜单 List InitList(); //初始化表 int IsFuLL(List L); //是否为满 void AddMember(List L); //添加成员
void DeleteMember(List L); //删除成员 void ModifyMember(List L); //修改成员信息 void SearchMember(List L); //查找成员信息 void PrintList(List L); //打印所有成员信息 int main() { List L; int choice = 0; L = InitList(); while (1) { PrintMenu(); printf("请输入选项: "); scanf("%d", &choice); switch (choice) { case 1: AddMember(L); break; case 2: DeleteMember(L); break; case 3: ModifyMember(L); break; case 4: SearchMember(L); break; case 5: PrintList(L); break; case 6: printf("程序退出!\n"); return 0; default: printf("输入有误,请重新输入!\n"); break; } } return 0; } void PrintMenu() { printf("\n\n"); printf("\t\t\t\t\t\t通讯录管理系统\n"); printf("\t\t\t\t\t\t1.添加成员\n"); printf("\t\t\t\t\t\t2.删除成员\n"); printf("\t\t\t\t\t\t3.修改成员信息\n"); printf("\t\t\t\t\t\t4.查找成员信息\n"); printf("\t\t\t\t\t\t5.打印所有成员信息\n"); printf("\t\t\t\t\t\t6.退出\n"); printf("\n\n"); } List InitList() { List L; L = (List)malloc(sizeof(struct SeqList)); L->count = 0; return L; } int IsFuLL(List L) { if (L->count == MaxSize) { printf("通讯录已满,无法添加新成员!\n"); return 1; } else { return 0; } } void AddMember(List L) { int i; if (IsFuLL(L)) { return; } printf("请输入成员信息:\n"); printf("学号:"); scanf("%d", &(L->Data[L->count].Number)); printf("身份证号:"); scanf("%s", L->Data[L->count].ID); printf("性别:"); scanf("%s", L->Data[L->count].Sex); printf("年龄:"); scanf("%d", &(L->Data[L->count].Age)); printf("生日:"); scanf("%s", L->Data[L->count].Birthday); L->count++; printf("成员添加成功!\n"); } void DeleteMember(List L) { int i, j, n; char ID[20]; printf("请输入要删除的成员身份证号:"); scanf("%s", ID); for (i = 0; i < L->count; i++) { if (strcmp(L->Data[i].ID, ID) == 0) { for (j = i; j < L->count - 1; j++) { L->Data[j] = L->Data[j + 1]; } L->count--; printf("删除成功!\n"); return; } } printf("没有找到该成员!\n"); } void ModifyMember(List L) { int i, n; char ID[20]; printf("请输入要修改的成员身份证号:"); scanf("%s", ID); for (i = 0; i < L->count; i++) { if (strcmp(L->Data[i].ID, ID) == 0) { printf("请输入修改后的信息:\n"); printf("学号:"); scanf("%d", &(L->Data[i].Number)); printf("身份证号:"); scanf("%s", L->Data[i].ID); printf("性别:"); scanf("%s", L->Data[i].Sex); printf("年龄:"); scanf("%d", &(L->Data[i].Age)); printf("生日:"); scanf("%s", L->Data[i].Birthday); printf("修改成功!\n"); return; } } printf("没有找到该成员!\n"); } void SearchMember(List L) { int i, n; char ID[20]; printf("请输入要查找的成员身份证号:"); scanf("%s", ID); for (i = 0; i < L->count; i++) { if (strcmp(L->Data[i].ID, ID) == 0) { printf("学号:%d\n", L->Data[i].Number); printf("身份证号:%s\n", L->Data[i].ID); printf("性别:%s\n", L->Data[i].Sex); printf("年龄:%d\n", L->Data[i].Age); printf("生日:%s\n", L->Data[i].Birthday); return; } } printf("没有找到该成员!\n"); } void PrintList(List L) { int i; if (L->count == 0) { printf("通讯录为空!\n"); return; } printf("所有成员信息如下:\n"); for (i = 0; i < L->count; i++) { printf("学号:%d\n", L->Data[i].Number); printf("身份证号:%s\n", L->Data[i].ID); printf("性别:%s\n", L->Data[i].Sex); printf("年龄:%d\n", L->Data[i].Age); printf("生日:%s\n", L->Data[i].Birthday); printf("\n"); } }
相关推荐
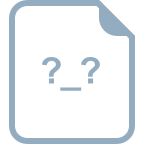
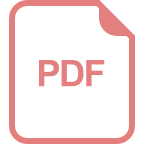
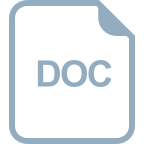













