32.Controller中的方法给客户端响应的是List集合,客户端如果使用的是Axios框架发出的请求,接收到响应时response.data得到的是数组. A.False B.True
时间: 2024-06-06 18:06:09 浏览: 40
选项 B. True。在 Spring MVC 的 Controller 中,如果处理方法返回的是 List 集合,那么客户端接收到响应时,得到的是数组。
Axios 是一个基于 Promise 的 HTTP 请求库,它可以在浏览器和 Node.js 中使用。当客户端使用 Axios 框架发出请求时,它会自动解析服务器返回的 JSON 数据,将其转换成 JavaScript 对象或数组。因此,如果服务器返回的是 List 集合,那么客户端接收到的响应数据就是数组。
所以,选项 B. True 是正确的描述。
相关问题
springboot 使用JavaScript利用axios向MySQL数据库获得全部信息返回前端渲染输出到div块中
首先,在Spring Boot中使用JavaScript,需要在HTML页面中引入JavaScript文件,可以在HTML页面中添加如下代码:
```html
<script src="https://cdn.jsdelivr.net/npm/axios/dist/axios.min.js"></script>
<script type="text/javascript" src="path/to/your/javascript/file.js"></script>
```
接着,在JavaScript文件中使用axios向后端发送请求,获取MySQL数据库中的全部信息。可以使用如下代码:
```javascript
axios.get('/api/getAllInfo')
.then(function(response) {
// 处理响应数据
var data = response.data;
// 将数据渲染输出到div块中
var output = document.getElementById('output');
data.forEach(function(item) {
var div = document.createElement('div');
div.innerHTML = item;
output.appendChild(div);
});
})
.catch(function(error) {
console.log(error);
});
```
在后端,需要编写一个Controller类来处理前端发送的请求,并将MySQL数据库中的数据返回给前端。可以使用如下代码:
```java
@RestController
@RequestMapping("/api")
public class MyController {
@Autowired
private MyService myService;
@GetMapping("/getAllInfo")
public List<String> getAllInfo() {
return myService.getAllInfoFromMySQL();
}
}
```
在上述代码中,`MyService`是一个服务类,用于从MySQL数据库中获取数据。可以根据自己的需求来编写该类。
最后,在HTML页面中添加一个div块,用于将获取到的数据渲染输出。可以使用如下代码:
```html
<div id="output"></div>
```
当JavaScript代码执行完毕后,MySQL数据库中的全部信息将会被渲染输出到上述div块中。
springboot 使用JavaScript利用axios向MySQL数据库获得多条信息返回前端渲染输出到多个div块中
首先,需要在前端页面引入axios库,并在后端使用SpringBoot框架搭建RESTful API提供数据接口。
1. 前端页面
在前端页面中,我们需要使用JavaScript代码调用axios库向后端发送请求,并将返回的数据渲染到页面上。
首先,我们需要在HTML文件中定义多个div块,用于展示不同的信息。例如:
```
<div id="div1"></div>
<div id="div2"></div>
<div id="div3"></div>
```
然后,在JavaScript中编写如下代码:
```
axios.get('/api/getData').then(function (response) {
// 获取到数据后,将数据渲染到对应的div块中
document.getElementById('div1').innerHTML = response.data[0];
document.getElementById('div2').innerHTML = response.data[1];
document.getElementById('div3').innerHTML = response.data[2];
}).catch(function (error) {
console.log(error);
});
```
在上述代码中,我们首先使用axios库向后端发送GET请求,请求对应的数据接口。接口的URL为“/api/getData”。在获取到数据后,我们将数据渲染到对应的div块中。这里假设返回的数据是一个数组,包含三个元素,分别对应三个div块中的内容。
2. 后端代码
在后端代码中,我们需要使用SpringBoot框架搭建RESTful API,提供数据接口给前端页面调用。在本例中,我们需要提供一个接口,返回多条信息供前端渲染。代码如下:
```
@RestController
@RequestMapping("/api")
public class ApiController {
@Autowired
private DataService dataService;
@GetMapping("/getData")
public List<String> getData() {
// 调用DataService中的方法获取数据
return dataService.getData();
}
}
```
在上述代码中,我们首先使用@RestController注解标记该类为RESTful API控制器,使用@RequestMapping注解指定路由前缀为“/api”。然后,我们定义了一个名为getData的接口,使用@GetMapping注解指定请求方法为GET,请求URL为“/getData”。在接口实现中,我们调用了一个名为dataService的服务,获取数据并返回给前端。
3. 数据库操作
在上述代码中,我们调用了一个名为dataService的服务,用于获取数据。在服务的实现中,我们需要使用JdbcTemplate或MyBatis等库进行数据库操作,获取需要的数据。这里以JdbcTemplate为例,代码如下:
```
@Service
public class DataService {
@Autowired
private JdbcTemplate jdbcTemplate;
public List<String> getData() {
// 查询数据库,获取需要的数据
List<String> data = jdbcTemplate.queryForList("SELECT field1, field2, field3 FROM table", String.class);
return data;
}
}
```
在上述代码中,我们首先使用@Service注解标记该类为服务类,使用@Autowired注解注入JdbcTemplate对象。然后,在getData方法中,我们使用JdbcTemplate进行数据库查询,获取需要的数据,并返回给调用方。
以上就是使用JavaScript利用axios向MySQL数据库获得多条信息返回前端渲染输出到多个div块中的方法。
阅读全文
相关推荐
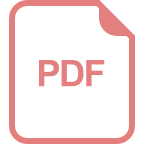
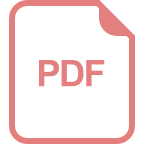
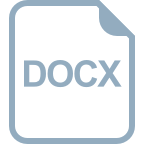
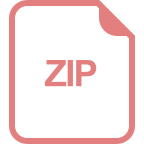
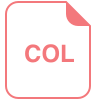
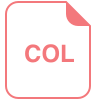
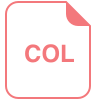









