修改程序def search_neighborhood(x, y): neighbors = [] for line in lines2: row = line.split() if row[0] == x and row[1] == y: neighbors.append(int(float(row[2]))) elif row[0] > x: break return neighbors,找到(x,y)附近的4邻域所有第三列的值并求平均值
时间: 2024-03-25 14:41:21 浏览: 50
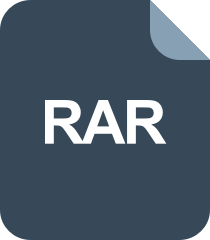
任输入三个数,求得平均值,平均值程序

可以按照以下方式修改程序:
```python
def search_neighborhood(x, y):
neighbors = []
total = 0
count = 0
for line in lines2:
row = line.split()
if row[0] == x and row[1] == y:
neighbors.append(int(float(row[2])))
elif row[0] > x:
break
for val in neighbors:
total += val
count += 1
if count > 0:
return total/count
else:
return 0
```
这个修改后的函数首先遍历整个列表 `lines2`,将符合条件的第三列的值添加到 `neighbors` 列表中,并记录符合条件的数量 `count` 和它们的总和 `total`。接着,如果列表中有任何元素,则计算平均值并返回,否则返回 0。
阅读全文
相关推荐
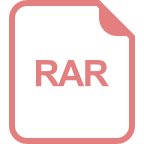
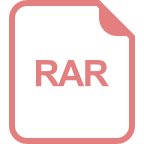





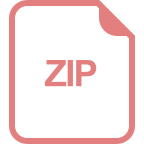
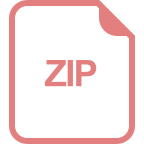
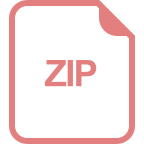
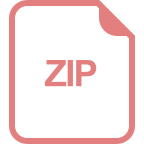
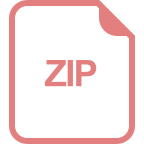
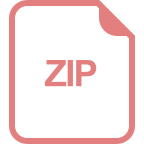



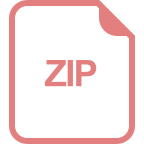
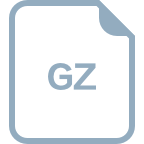