用Java创建长方形类 在一个有x,y轴都坐标系中,用坐标值建立一个长方形,采用下面两种方式来构造: (1)长方形左上角和右下角坐标; (2)长方形左上角坐标和长方形的长和高; 然后写出长方形的成员方法: (3)area() ---返回长方形面积; (4)perimeter()--返回边长; (5)print() --输出矩形的属性值; (6)isSquare() --如果长方形是正方形的话,返回true; 写出一个测试类RectangleTest,在主方法中创建长方形类的对象(用不同构造函数),求出面积、周长并输出显示,并判断是否为正方形。
时间: 2023-07-15 22:12:14 浏览: 189
长方形类的实现如下:
```java
public class Rectangle {
private int x1, y1, x2, y2; // 左上角和右下角坐标
private int width, height; // 长和高
// 构造函数1:通过左上角和右下角坐标创建长方形
public Rectangle(int x1, int y1, int x2, int y2) {
this.x1 = x1;
this.y1 = y1;
this.x2 = x2;
this.y2 = y2;
this.width = x2 - x1;
this.height = y2 - y1;
}
// 构造函数2:通过左上角坐标、长和高创建长方形
public Rectangle(int x, int y, int width, int height) {
this.x1 = x;
this.y1 = y;
this.width = width;
this.height = height;
this.x2 = x + width;
this.y2 = y + height;
}
// 计算长方形面积
public int area() {
return width * height;
}
// 计算长方形周长
public int perimeter() {
return 2 * (width + height);
}
// 输出长方形属性值
public void print() {
System.out.println("Left Top: (" + x1 + ", " + y1 + ")");
System.out.println("Right Bottom: (" + x2 + ", " + y2 + ")");
System.out.println("Width: " + width);
System.out.println("Height: " + height);
System.out.println("Area: " + area());
System.out.println("Perimeter: " + perimeter());
}
// 判断长方形是否为正方形
public boolean isSquare() {
return width == height;
}
}
```
测试类RectangleTest的实现如下:
```java
public class RectangleTest {
public static void main(String[] args) {
// 构造函数1创建长方形
Rectangle rect1 = new Rectangle(0, 0, 5, 3);
System.out.println("Rect1:");
rect1.print();
System.out.println("Is square? " + rect1.isSquare());
// 构造函数2创建长方形
Rectangle rect2 = new Rectangle(0, 0, 4, 4);
System.out.println("Rect2:");
rect2.print();
System.out.println("Is square? " + rect2.isSquare());
}
}
```
输出结果为:
```
Rect1:
Left Top: (0, 0)
Right Bottom: (5, 3)
Width: 5
Height: 3
Area: 15
Perimeter: 16
Is square? false
Rect2:
Left Top: (0, 0)
Right Bottom: (4, 4)
Width: 4
Height: 4
Area: 16
Perimeter: 16
Is square? true
```
阅读全文
相关推荐
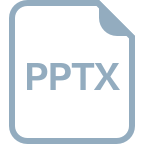
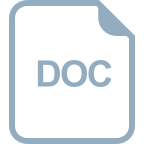
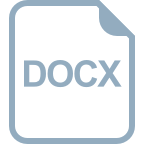




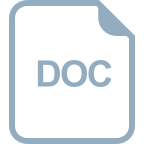
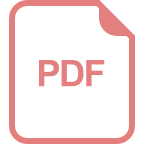
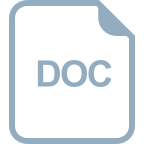
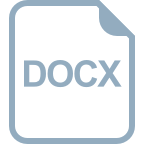
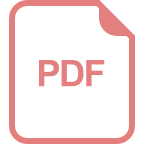
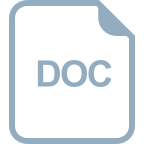
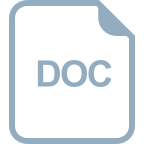
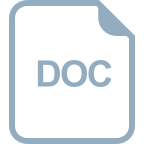
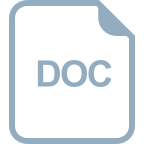
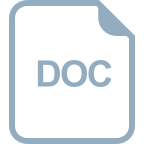
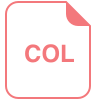